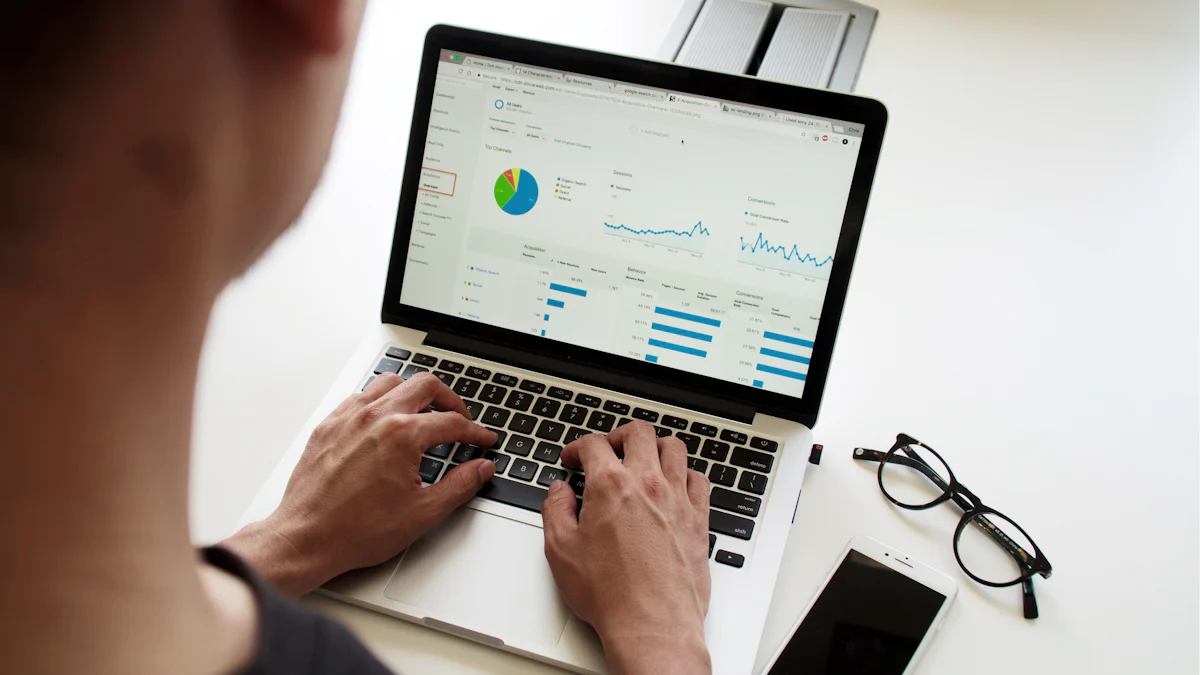
Data structures are the backbone of computer science, playing a pivotal role in how data is organized and manipulated. By understanding what is data structure, developers can significantly enhance software efficiency and performance. The choice of the right data structure can lead to faster processing speeds and reduced computational overhead, which are crucial for handling complex applications and large datasets. This blog delves into the practical applications of data structures, showcasing their impact on real-world scenarios and highlighting their importance in optimizing system performance and scalability.
What is Data Structure
Understanding data structures is fundamental to the world of computer science and software development. They are the frameworks that allow us to store, organize, and manage data efficiently, paving the way for effective algorithm design and application performance.
Understanding the Basics
Definition and Importance
At its core, a data structure is a specialized format for organizing and storing data. It enables efficient access and modification, which is crucial as applications become more complex and data volumes increase. Data structures are not just about storing data; they are about doing so in a way that optimizes space and time complexity. This optimization is vital for ensuring that applications run smoothly and can scale effectively as demands grow.
The importance of data structures extends beyond mere storage. They form the backbone of many algorithms, influencing their design and efficiency. For instance, choosing the right data structure can significantly impact the performance and scalability of an application, as highlighted in various studies. Efficient data organization leads to faster processing speeds and reduced computational overhead, which are essential for handling large datasets and complex operations.
Role in Software Development
In software development, data structures play a pivotal role in shaping how software is designed and implemented. They provide the foundation for building robust and scalable systems. By understanding what is data structure, developers can make informed decisions about which structures to use, depending on the specific needs of their applications.
-
Algorithm Design: Data structures are integral to algorithm design. They determine how data is accessed and manipulated, directly affecting the efficiency of the algorithm. Choosing the right structure can lead to significant improvements in performance.
-
Problem Solving: In real-world applications, data structures are indispensable tools for solving complex problems. They allow developers to model data in a way that aligns with the problem at hand, facilitating innovative solutions.
-
Scalability and Performance: As applications grow, the ability to scale efficiently becomes paramount. Data structures provide the mechanisms needed to manage large volumes of data without compromising on performance. This is particularly relevant in fields like big data and machine learning, where efficient data handling is critical.
Fundamental Data Structures
Understanding the core building blocks of data structures is essential for any software developer. These structures form the foundation upon which complex algorithms and applications are built. In this section, we delve into the fundamental data structures: arrays, linked lists, stacks, and queues, each serving unique purposes and offering distinct advantages in software development.
Arrays
Characteristics and Operations
Arrays are one of the most basic yet powerful data structures. They consist of a collection of elements, each identified by an index or key. The simplicity of arrays lies in their ability to store multiple items of the same type together, allowing for efficient data access and manipulation.
- Fixed Size: Arrays have a fixed size, meaning the number of elements they can hold is determined at the time of creation.
- Index-Based Access: This feature allows for constant-time complexity, O(1), when accessing elements, making arrays ideal for scenarios where quick data retrieval is necessary.
- Operations: Common operations include insertion, deletion, and traversal, each with varying time complexities depending on the operation and position within the array.
Use Cases in Software Development
Arrays are widely used across various domains due to their efficiency and simplicity:
- Data Storage: In databases, arrays can be used to store collections of records, enabling fast access and updates.
- Image Processing: 2D arrays are often utilized to represent pixel data, facilitating image manipulation and analysis.
- Networking: Arrays help manage routing tables, optimizing data flow across networks.
Linked Lists
Types of Linked Lists
Linked lists offer a dynamic alternative to arrays, providing flexibility in memory allocation. They consist of nodes, each containing data and a reference to the next node in the sequence.
- Singly Linked List: Each node points to the next, forming a unidirectional chain.
- Doubly Linked List: Nodes contain references to both the next and previous nodes, allowing bidirectional traversal.
- Circular Linked List: The last node points back to the first, creating a circular structure.
Applications in Memory Management
Linked lists are invaluable in scenarios requiring dynamic memory management:
- Dynamic Data Structures: They allow for efficient insertion and deletion of elements without the need for reallocation or resizing, as seen in applications like task scheduling and memory allocation.
- Operating Systems: Linked lists are used to manage processes and tasks, ensuring efficient CPU utilization.
Stacks and Queues
LIFO and FIFO Principles
Stacks and queues are specialized data structures that follow specific operational principles:
- Stack (LIFO): Last-In-First-Out, where the last element added is the first to be removed. This principle is akin to a stack of plates, where you add and remove from the top.
- Queue (FIFO): First-In-First-Out, where the first element added is the first to be removed, similar to a line of people waiting for service.
Real-World Examples in Task Scheduling
These structures are pivotal in managing tasks and processes:
- Function Calls: Stacks manage function calls in programming, ensuring proper execution order and memory management.
- Task Scheduling: Queues are used in operating systems to manage process scheduling, optimizing resource allocation and system performance.
Advanced Data Structures
In the realm of computer science, advanced data structures provide the backbone for efficient data management and problem-solving. These structures are designed to handle complex data relationships and operations, offering robust solutions for real-world applications.
Trees
Binary Trees and Their Variants
Binary trees are a cornerstone in the world of data structures, characterized by nodes with up to two children. They are particularly useful for implementing efficient searching, insertion, and deletion operations. Among their variants, binary search trees (BSTs) stand out for maintaining sorted data, making them ideal for applications like dictionaries and database indexing.
- Binary Search Trees (BSTs): These trees allow for quick data retrieval, insertion, and deletion, all in logarithmic time. This efficiency is crucial when managing sorted lists or implementing dictionaries.
- AVL Trees and Red-Black Trees: These are self-balancing BSTs that maintain height balance, ensuring optimal performance even in the worst-case scenarios.
“Binary search trees offer practical ways to sort things and manage objects arranged in a given priority.”
Applications in Database Indexing
In database systems, binary trees are often employed to create indexes, facilitating fast data retrieval. By organizing data hierarchically, they enable efficient searching and sorting, which is vital for maintaining large datasets. This capability is exemplified in the TiDB database, where binary trees help optimize query performance and ensure data consistency.
Graphs
Directed and Undirected Graphs
Graphs are versatile data structures used to model relationships between objects. They consist of nodes (vertices) and edges (connections), with directed graphs having edges with specific directions and undirected graphs having bidirectional edges.
- Directed Graphs: Useful in representing one-way relationships, such as web page links or network routing paths.
- Undirected Graphs: Ideal for modeling mutual connections, like social networks or road maps.
Network Analysis and Pathfinding
Graphs play a pivotal role in network analysis and pathfinding algorithms. They are used to determine the most efficient paths for data transmission, optimize network flow, and analyze connectivity. In applications like GPS navigation and internet routing, graphs help identify shortest paths and improve overall system efficiency.
“Trees and graphs are used in routing protocols to determine the most efficient paths for data transmission.”
Hash Tables
Hashing Techniques
Hash tables are powerful data structures that provide fast data lookup through hashing techniques. They map keys to values using a hash function, allowing for constant-time complexity on average for search, insert, and delete operations.
- Open Addressing and Chaining: These are common methods to handle collisions, ensuring that hash tables remain efficient even when dealing with large datasets.
Use in Data Retrieval and Storage
Hash tables are indispensable in scenarios requiring rapid data retrieval and storage. They are extensively used in databases for indexing, caching mechanisms, and symbol tables. In the TiDB database, hash-based data structures enhance data indexing and retrieval, supporting high-performance applications across various industries.
“Hash-based data structures are used in symbol tables, dictionaries, spell checkers, autocomplete systems, data indexing, and databases for efficient search and retrieval operations.”
Real-World Applications of Data Structures
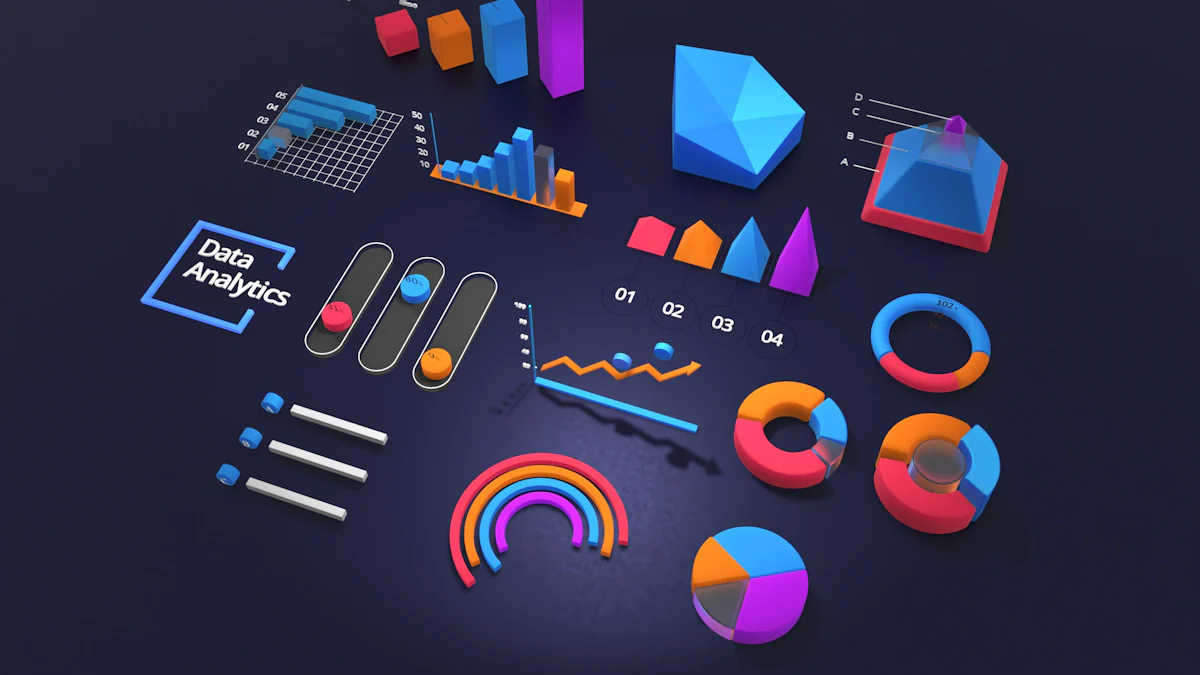
Web Development
Data Structures in Frontend and Backend
In the realm of web development, data structures are pivotal in both frontend and backend operations. On the frontend, data structures like arrays and trees facilitate efficient DOM manipulation and rendering, ensuring that user interfaces are responsive and dynamic. For instance, trees are used to represent the hierarchical structure of HTML documents, allowing browsers to efficiently parse and render web pages.
On the backend, data structures such as hash tables and graphs play a crucial role in managing and retrieving data quickly. Hash tables are often employed in caching mechanisms to speed up data access, while graphs are used to model relationships between users or entities, enhancing functionalities like social networking features or recommendation systems.
Enhancing User Experience and Performance
The strategic use of data structures directly impacts user experience by optimizing performance. Efficient data handling ensures that web applications load faster and respond promptly to user interactions. For example, using queues can manage asynchronous tasks, such as API requests, without blocking the main thread, thus maintaining a smooth user experience. By leveraging appropriate data structures, developers can create scalable and high-performing web applications that meet modern user expectations.
Machine Learning
Data Structures for Model Training
In machine learning, data structures are integral to managing and processing large datasets required for training models. Arrays and matrices are fundamental in representing data points and performing mathematical operations essential for model training. They enable efficient computation of linear algebra operations, which are the backbone of many machine learning algorithms.
Moreover, trees and graphs are utilized in specific machine learning models. Decision trees, for instance, are used for classification tasks, providing a clear path from input features to output predictions. Graph-based models are employed in scenarios where relationships between data points are crucial, such as in social network analysis or recommendation systems.
Optimizing Algorithms with Efficient Data Handling
Efficient data handling is critical in optimizing machine learning algorithms. By choosing the right data structures, such as hash tables for quick data retrieval or heaps for priority queue management, developers can enhance algorithm performance and reduce computational overhead. This optimization is especially important in real-time applications where quick decision-making is required, such as fraud detection or autonomous driving systems.
Database Management with PingCAP’s TiDB
Structuring Data for Fast Access
PingCAP’s TiDB database exemplifies the power of advanced data structures in database management. By employing structures like B-trees and hash indexes, TiDB ensures rapid data access and retrieval, which is crucial for applications requiring high throughput and low latency. These data structures enable TiDB to handle large-scale transactional queries efficiently, supporting businesses in maintaining seamless operations.
Ensuring Data Integrity and Consistency
Data integrity and consistency are paramount in database management, and TiDB excels in this area through its robust data structures. The use of distributed hash tables and consensus algorithms like Raft ensures that data remains consistent across distributed systems. This capability is vital for applications that demand high availability and strong consistency, such as financial transactions and e-commerce platforms.
Case Studies: Huya Live, ZaloPay, Shopee, BIGO
Huya Live leveraged TiDB’s horizontal scalability and strong consistency to overcome challenges related to storage capacity and high latency in live broadcasts. By adopting TiDB, Huya Live improved query performance significantly, enhancing user experience during live streaming events.
ZaloPay, a mobile payment application, utilized TiDB’s scalability to handle a significant volume of users and transactions. The database’s ability to support large-scale transactional queries ensured that ZaloPay could maintain high availability and resiliency, crucial for financial services.
Shopee faced the challenge of managing high-frequency read-only queries during big promotion campaigns. By integrating TiDB, Shopee optimized query performance and stability, ensuring seamless shopping experiences for millions of users.
BIGO deployed TiDB for analytical processing and big data management, benefiting from features like the pessimistic transaction model and real-time HTAP capabilities. This deployment enabled BIGO to handle large-scale data efficiently, supporting their global operations and growth.
These case studies illustrate how PingCAP’s TiDB database, with its advanced data structures, provides robust solutions for diverse industries, enhancing performance, scalability, and reliability.
Choosing the right data structure is pivotal for efficient software design and performance. It ensures that applications run smoothly and effectively, avoiding slow runtimes or unresponsive code. As technology evolves, so do data structures, offering new opportunities for innovation. We encourage you to explore and experiment with various structures to find the best fit for your needs. By doing so, you not only enhance your technical skills but also contribute to the ever-evolving landscape of technology, where data structures continue to play a critical role in shaping the future.