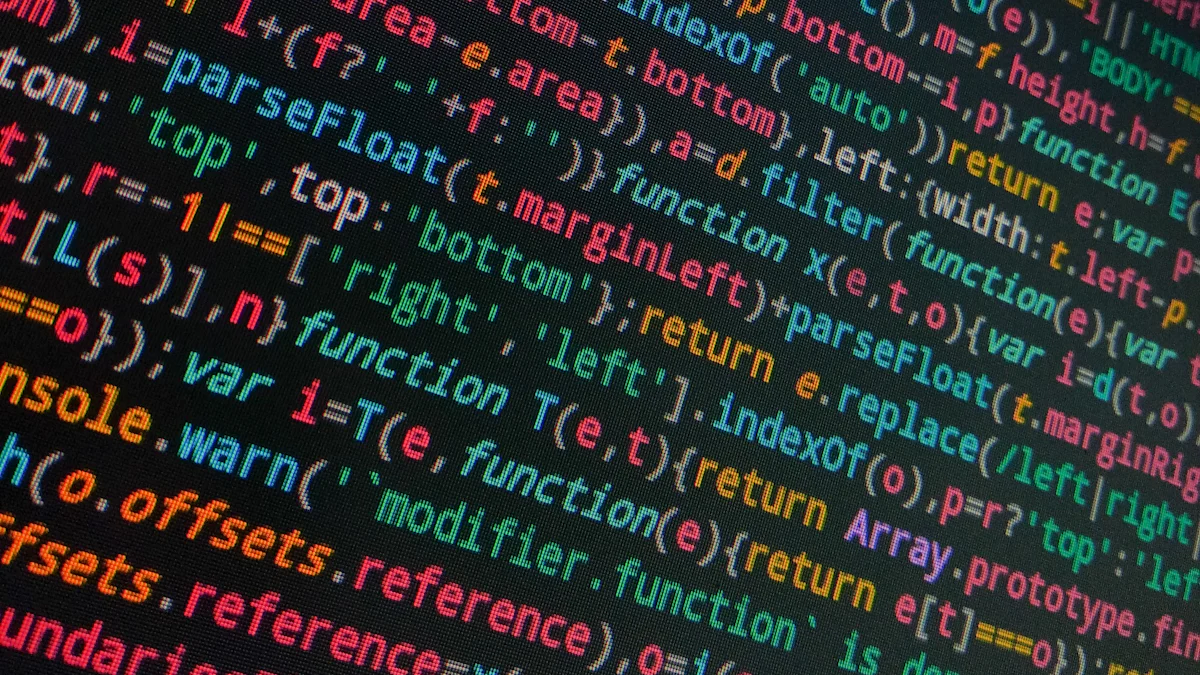
MySQLdb is a crucial library for Python developers working with MySQL databases, facilitating seamless database interactions. However, installing MySQLdb Python can be fraught with challenges, often leaving developers puzzled by cryptic error messages and compatibility issues. This blog aims to demystify these installation hurdles, providing clear and actionable solutions to ensure a smooth setup process. By addressing common pitfalls, we empower developers to focus on building robust applications without the distraction of technical roadblocks.
Understanding MySQLdb and Its Role in Python
What is MySQLdb?
Overview of MySQLdb
MySQLdb is a vital interface that allows Python developers to connect seamlessly with MySQL database servers. It adheres to the Python Database API v2.0 standards, ensuring a consistent and reliable experience for database interactions. Built on top of the robust MySQL C API, MySQLdb provides a powerful yet straightforward way to execute SQL queries, manage transactions, and handle database connections directly from Python code.
Importance in Database Management
In the realm of database management, MySQLdb plays a crucial role by enabling efficient data manipulation and retrieval. It supports a wide array of data types and operations, making it an indispensable tool for developers who need to manage complex datasets. The ability to integrate MySQLdb with Python applications ensures that developers can leverage Python’s extensive libraries and frameworks to build scalable and high-performance applications. This integration is particularly beneficial for organizations that rely on MySQL databases for their critical operations, as it facilitates the creation, access, and manipulation of data through a structured query language (SQL).
Why Use MySQLdb in Python?
Benefits of Integrating MySQLdb with Python
Integrating MySQLdb with Python offers several advantages that enhance both development efficiency and application performance:
- Seamless Integration: MySQLdb enables Python programs to interact with MySQL databases effortlessly, allowing developers to execute SQL commands and manage database transactions without leaving the Python environment.
- Performance Optimization: By utilizing the MySQL C API, MySQLdb ensures fast and efficient database operations, which is crucial for applications that require real-time data processing and analysis.
- Flexibility and Scalability: The combination of Python’s versatility and MySQLdb’s robust database capabilities allows developers to build applications that can scale with growing data demands while maintaining high performance.
Common Use Cases
MySQLdb Python is widely used across various industries and applications due to its reliability and ease of use. Some common use cases include:
- Web Development: Many web applications rely on MySQLdb Python to handle user data, manage sessions, and store content dynamically.
- Data Analysis: Data scientists and analysts use MySQLdb to extract and manipulate large datasets stored in MySQL databases, enabling them to perform complex analyses and generate insights.
- Enterprise Applications: Organizations utilize MySQLdb Python to manage their business-critical data, ensuring secure and efficient data handling across different departments and systems.
By understanding the significance of MySQLdb and its integration with Python, developers can harness the full potential of their MySQL databases, driving innovation and efficiency in their projects.
Common Installation Errors in MySQLdb Python
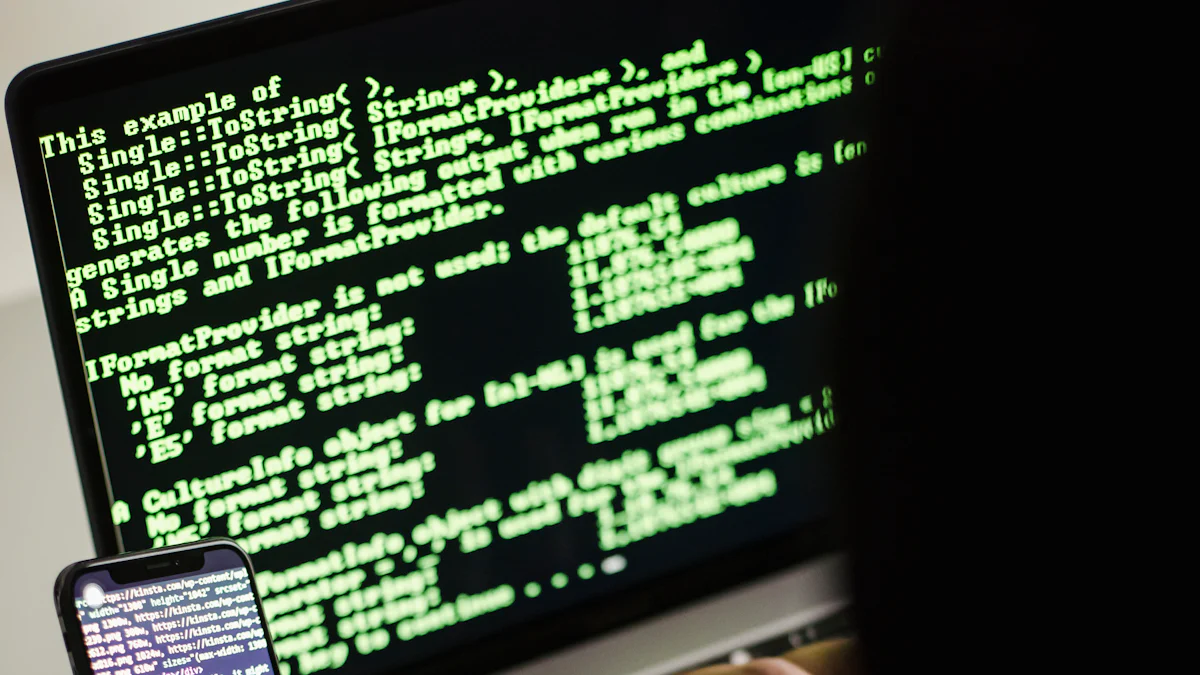
When working with MySQLdb Python, developers often encounter a range of installation errors that can disrupt their workflow. Understanding these errors and knowing how to resolve them is crucial for maintaining productivity and ensuring seamless database interactions.
Error: “No module named MySQLdb”
Explanation of the error
The “No module named MySQLdb” error typically occurs when Python cannot locate the MySQLdb module within your environment. This issue is common when the module has not been installed correctly or if there is a mismatch between the Python version and the installed MySQLdb package.
Steps to resolve the issue
To resolve this error, follow these steps:
-
Verify Installation: Ensure that MySQLdb Python is installed. You can do this by running the following command in your terminal:
pip list | grep mysqlclient
If
mysqlclient
is not listed, proceed to install it. -
Install MySQLdb Python: Use pip to install the MySQLdb Python package:
pip install mysqlclient
-
Check Python Version Compatibility: Ensure that you are using a compatible version of Python. MySQLdb Python (mysqlclient) supports Python 3.x. If you are using Python 2.x, consider upgrading to Python 3.x or using an alternative like
MySQL Connector/Python
. -
Virtual Environment: If issues persist, try setting up a virtual environment to isolate dependencies:
python -m venv myenv source myenv/bin/activate pip install mysqlclient
Error: “MySQLdb not found”
Possible causes
This error indicates that the MySQLdb module is missing from your Python path. It can occur due to incomplete installations, incorrect paths, or conflicts with other packages.
Troubleshooting steps
To troubleshoot and resolve this error:
-
Reinstall MySQLdb Python: Uninstall any existing installations and reinstall:
pip uninstall mysqlclient pip install mysqlclient
-
Check System Path: Ensure that your Python path includes the directory where MySQLdb is installed. You can modify the
PYTHONPATH
environment variable if necessary. -
Dependencies: Verify that all necessary dependencies are installed. On Ubuntu, you might need:
sudo apt-get install python3-dev default-libmysqlclient-dev build-essential
-
Manual Installation: If pip fails, consider downloading the package manually and installing it using:
python setup.py install
Error: “Cannot connect to MySQL server”
Understanding the error
This error arises when MySQLdb Python cannot establish a connection to the MySQL server. It may be due to incorrect connection parameters, server unavailability, or network issues.
Solutions to fix the connection issue
To fix this connection issue:
-
Verify Connection Parameters: Double-check your host, port, username, password, and database name. Ensure they match the server settings.
-
Server Status: Confirm that your MySQL server is running and accessible. Use:
systemctl status mysql
-
Network Configuration: Ensure that your firewall or network settings allow connections to the MySQL server’s port (default is 3306).
-
SSL Configuration: If SSL is required, ensure that your connection string includes the necessary SSL parameters. For example:
db_conf = { "host": "your_tidb_host", "port": 4000, "user": "your_user", "password": "your_password", "database": "your_database", "ssl_mode": "VERIFY_IDENTITY", "ssl": {"ca": "path_to_ca_certificate"} } connection = MySQLdb.connect(**db_conf)
By addressing these common installation errors, developers can ensure a smoother experience with MySQLdb Python, allowing them to focus on leveraging the full potential of their MySQL databases for robust application development.
Best Practices for Successful Installation of MySQLdb Python
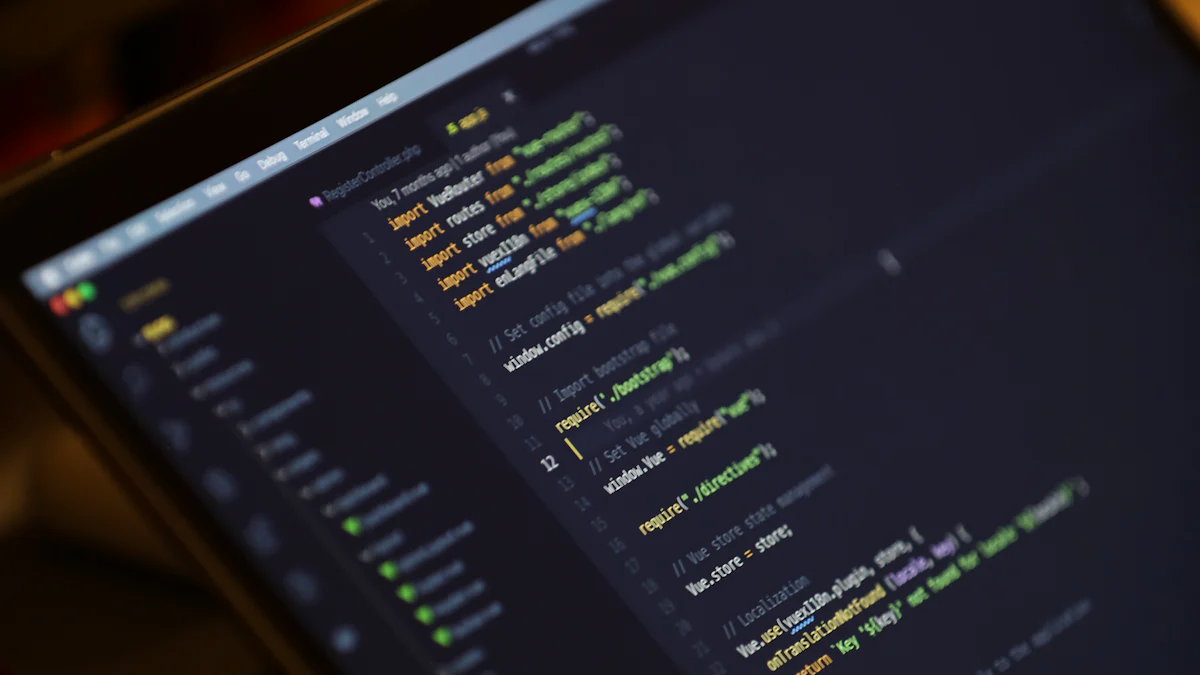
Ensuring a smooth installation of MySQLdb Python is crucial for developers aiming to leverage the full potential of MySQL databases in their applications. By following best practices, you can avoid common pitfalls and streamline the setup process.
Preparing Your Environment
System Requirements
Before diving into the installation, it’s essential to verify that your system meets the necessary requirements for running MySQLdb Python. This involves:
- Operating System: Ensure compatibility with your OS. MySQLdb Python is widely supported on Linux, macOS, and Windows.
- Python Version: MySQLdb Python (mysqlclient) supports Python 3.x. It’s advisable to use the latest stable release of Python to benefit from improved performance and security features.
- MySQL Server: Ensure that a compatible version of MySQL server is installed and running on your system.
Installing Necessary Dependencies
Dependencies play a vital role in the successful installation of MySQLdb Python. Here’s how to set them up:
- Linux Systems: Use the package manager to install development libraries:
sudo apt-get install python3-dev default-libmysqlclient-dev build-essential
- macOS: You may need to install Xcode command line tools and Homebrew, then run:
brew install mysql-client
- Windows: Ensure that Visual C++ Build Tools are installed, as they are required for compiling the package.
Step-by-Step Installation Guide
Using pip for Installation
With your environment prepared, you can proceed to install MySQLdb Python using pip, the recommended package manager for Python:
- Open Terminal or Command Prompt: Navigate to your project directory or a virtual environment.
- Install mysqlclient: Execute the following command:
pip install mysqlclient
This command fetches the latest version of MySQLdb Python from the Python Package Index (PyPI).
Verifying the Installation
After installation, it’s important to confirm that MySQLdb Python is correctly set up:
- Check Installed Packages: Run the following command to verify the presence of mysqlclient:
pip list | grep mysqlclient
- Test Import: Open a Python shell and attempt to import MySQLdb:
import MySQLdb
If no errors occur, the installation is successful.
Testing Your Installation
Running a Sample Script
To ensure everything is functioning as expected, run a simple script that connects to your MySQL database:
import MySQLdb
def test_connection():
try:
connection = MySQLdb.connect(
host="localhost",
user="your_user",
passwd="your_password",
db="your_database"
)
print("Connection successful!")
except MySQLdb.Error as e:
print(f"Error connecting to MySQLdb: {e}")
test_connection()
Replace your_user
, your_password
, and your_database
with your actual database credentials. If the script prints “Connection successful!”, your setup is complete.
Debugging Common Issues
Should you encounter issues during testing, consider these troubleshooting tips:
- Check Credentials: Verify that the username, password, and database name are correct.
- Firewall and Network: Ensure that your firewall settings allow connections to the MySQL server’s port.
- Error Messages: Pay attention to any error messages for clues on what might be wrong, such as missing dependencies or incorrect configurations.
By adhering to these best practices, you can effectively install and configure MySQLdb Python, ensuring a robust foundation for your database-driven applications.
Leveraging TiDB with MySQLdb Python
Integrating TiDB database with MySQLdb Python opens up a world of possibilities for developers seeking a robust, scalable, and MySQL-compatible solution. This section explores the benefits of using TiDB and provides practical guidance on integrating it with MySQLdb Python.
Benefits of Using TiDB
MySQL Compatibility
One of the standout features of the TiDB database is its seamless compatibility with MySQL. This compatibility ensures that developers can migrate their existing MySQL applications to TiDB without significant code changes. By embracing the MySQL ecosystem, TiDB allows for a smoother transition, making it an attractive option for those looking to enhance their database capabilities while maintaining familiar MySQL syntax and operations.
Scalability and High Availability
TiDB’s distributed architecture offers unparalleled horizontal scalability, allowing databases to grow alongside your application’s demands. This means you can add more nodes to your cluster without downtime, ensuring that your application remains responsive even as data volumes increase. Additionally, TiDB’s distributed architecture provides high availability through its multi-replica setup, ensuring that your data is always accessible and secure, even in the event of hardware failures.
Integrating MySQLdb with TiDB
Connection Setup
To integrate MySQLdb Python with the TiDB database, you’ll need to set up a connection that leverages TiDB’s MySQL compatibility. Here’s a simple example of how to establish this connection:
import MySQLdb
def connect_to_tidb():
try:
connection = MySQLdb.connect(
host="your_tidb_host",
port=4000, # Default TiDB port
user="your_user",
passwd="your_password",
db="your_database"
)
print("Connected to TiDB successfully!")
except MySQLdb.Error as e:
print(f"Connection error: {e}")
connect_to_tidb()
Replace your_tidb_host
, your_user
, your_password
, and your_database
with your actual TiDB credentials. This setup allows you to leverage MySQLdb Python to interact with TiDB just as you would with a traditional MySQL server.
Example Use Cases
The integration of MySQLdb Python with TiDB opens up several compelling use cases:
-
Real-Time Analytics: With TiDB’s unique mixed workload processing layer, you can perform real-time analytics on transactional data without affecting performance. This is particularly beneficial for applications requiring immediate insights from large datasets.
-
Scalable Web Applications: For web applications experiencing rapid growth, TiDB’s scalability ensures that your database infrastructure can expand seamlessly. MySQLdb Python facilitates easy interaction with TiDB, allowing developers to focus on building features rather than managing database limitations.
-
Enterprise Data Management: TiDB’s high availability and ACID transaction support make it ideal for enterprise applications that require reliable and consistent data management. MySQLdb Python serves as a bridge, enabling efficient data operations and management.
By leveraging the strengths of TiDB with MySQLdb Python, developers can build powerful, scalable, and resilient applications that meet modern data demands.
In this blog, we explored the intricacies of installing MySQLdb Python, addressing common errors and providing practical solutions. By following these troubleshooting steps, developers can ensure a seamless integration with their MySQL databases, enhancing application performance and reliability. We encourage you to apply these insights to your projects and invite you to share your experiences or pose questions. Engaging with the community not only enriches your understanding but also contributes to collective learning. Let’s continue to innovate and overcome technical challenges together.