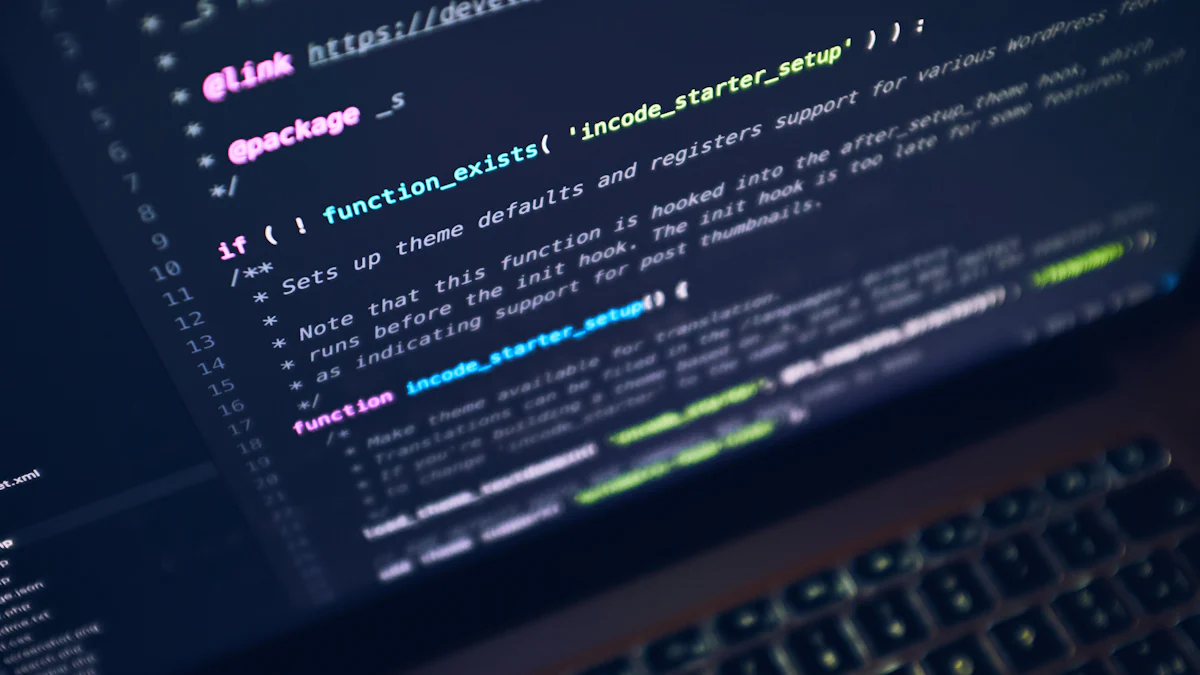
Efficiently updating rows in SQL is crucial for maintaining optimal database performance and resource management. The right sql command to update
can significantly enhance query optimization, ensuring that data distribution statistics remain accurate. TiDB database plays a pivotal role in this realm by offering advanced capabilities that streamline the update process. Its distributed architecture not only supports large-scale data operations but also ensures high availability and consistency, making it an invaluable tool for businesses seeking to optimize their database performance.
Understanding SQL Command to Update
In the realm of database management, understanding the nuances of the sql command to update
is essential for ensuring both performance and accuracy. This section delves into the foundational aspects of SQL update commands and highlights the significance of efficient updates.
Basics of SQL Command to Update
Syntax and Usage
The SQL UPDATE statement is a powerful tool used to modify existing records within a database table. Its basic syntax involves specifying the table to be updated, the columns to be modified, and the conditions under which the update should occur. Here’s a simple example:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
This command allows you to update one or multiple columns in a single query. The inclusion of a WHERE
clause is crucial as it defines the specific rows that need modification. Without it, the update would apply to all rows in the table, potentially leading to unintended data changes.
Common Pitfalls
While the sql command to update
is straightforward, several common pitfalls can affect its efficiency:
- Neglecting the WHERE Clause: Always double-check the
WHERE
clause to ensure only intended rows are modified. An incorrect or missing condition can lead to widespread data errors. - Bulk Updates: For large datasets, using bulk-update techniques is recommended to avoid holding locks for too long or causing conflicts. This approach optimizes performance by minimizing the transaction size.
- Temporary Tables: Storing exact data to update in a temporary table can speed up the update process, especially when dealing with complex conditions or large volumes of data.
Importance of Efficiency in Updates
Performance Implications
Efficient updates are vital for maintaining optimal database performance. An optimized sql command to update
reduces the time taken for transactions, thereby enhancing overall system responsiveness. For instance, using an UPDATE JOIN
can streamline operations by combining data from multiple tables, but it’s crucial to optimize these joins to prevent performance bottlenecks.
Resource Management
Efficiently managing resources is another critical aspect of updating rows in SQL. By ensuring that updates are executed swiftly and accurately, you minimize the load on the database server, freeing up resources for other operations. This is particularly important in high-concurrency environments where multiple transactions occur simultaneously.
Overview of TiDB’s Capabilities
In the ever-evolving landscape of database management, TiDB database stands out with its robust capabilities that cater to the demands of modern data-driven applications. Let’s explore how TiDB enhances efficiency and reliability in SQL updates.
Horizontal Scalability
Benefits for Large Datasets
One of the standout features of TiDB database is its horizontal scalability. This capability allows businesses to seamlessly expand their database infrastructure by adding more nodes, rather than overhauling existing systems. For large datasets, this means:
- Efficient Data Handling: As data volumes grow, TiDB ensures that performance remains consistent by distributing the load across multiple nodes. This prevents bottlenecks and maintains swift data access and updates.
- Cost-Effective Scaling: Instead of investing in costly hardware upgrades, organizations can scale out their databases incrementally, optimizing resource allocation and reducing overhead costs.
Load Balancing
Load balancing is integral to maintaining a responsive and reliable database environment. TiDB excels in this area by:
- Dynamic Distribution: It intelligently distributes queries and transactions across available nodes, ensuring that no single node becomes a point of failure or slowdown.
- Enhanced Performance: By balancing the workload, TiDB minimizes latency and maximizes throughput, even during peak usage times.
High Availability
Ensuring Data Consistency
Data consistency is paramount in any database system, and TiDB database guarantees this through its high availability architecture:
- Multiple Replicas: TiDB employs multiple replicas of data across different nodes, ensuring that even if one node fails, the data remains accessible and consistent.
- Strong Consistency Protocols: Utilizing protocols like Multi-Raft, TiDB ensures that all replicas are synchronized, providing a consistent view of the data at all times.
Fault Tolerance
In today’s fast-paced digital world, downtime is not an option. TiDB’s fault tolerance mechanisms ensure:
- Automatic Failover: In the event of a node failure, TiDB automatically reroutes traffic to healthy nodes, minimizing disruption and maintaining service continuity.
- Resilient Architecture: Its distributed nature means that the impact of hardware failures is mitigated, allowing businesses to focus on growth rather than maintenance.
By leveraging these capabilities, TiDB database empowers organizations to handle complex data operations with ease, ensuring that their systems are both scalable and resilient.
Key Features of TiDB for Efficient Updates
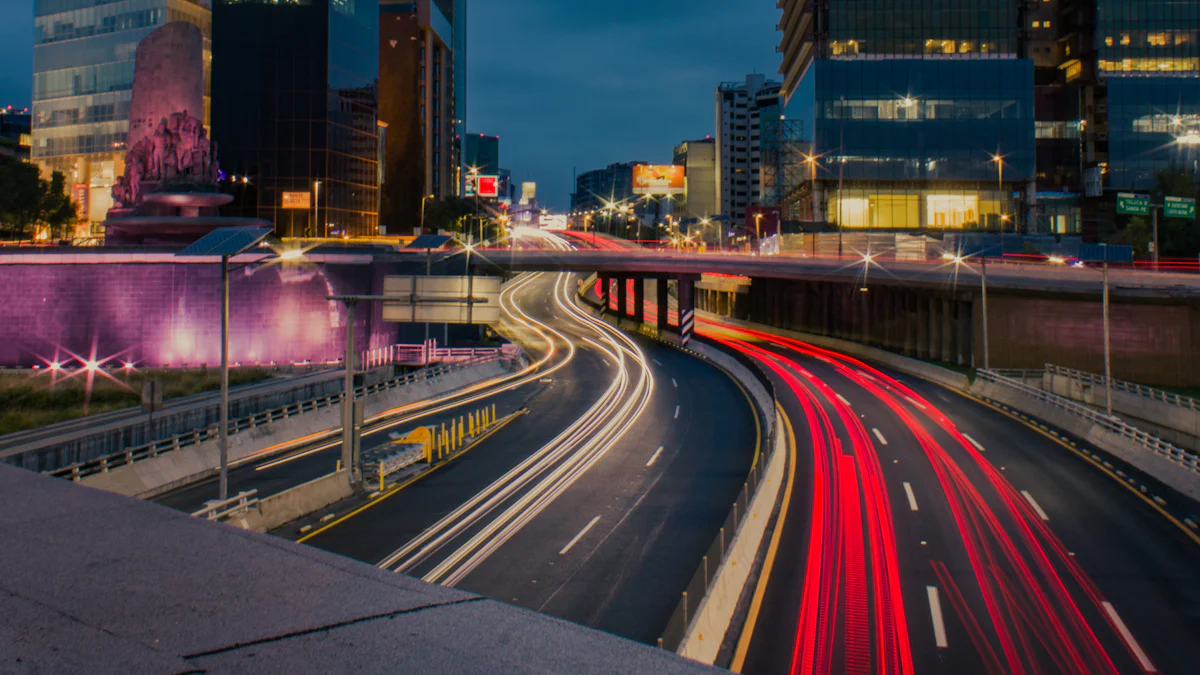
In the dynamic world of data management, the ability to perform updates efficiently is crucial. The TiDB database offers a suite of features designed to optimize the sql command to update
process, ensuring that your data operations are both swift and reliable.
Bulk Updates
Techniques and Strategies
When dealing with large datasets, bulk updates become essential. The TiDB database supports various techniques to streamline these operations. One effective strategy is to break down large updates into smaller, manageable batches. This approach minimizes lock contention and reduces the risk of transaction conflicts. Additionally, leveraging temporary tables to store intermediate results can significantly enhance the speed and efficiency of bulk updates.
Performance Benefits
The performance benefits of using bulk updates in the TiDB database are substantial. By processing updates in batches, you can achieve faster execution times and lower resource consumption. This not only improves the overall throughput of your database but also ensures that other operations continue to run smoothly without being hindered by long-running update transactions.
Transaction Management
ACID Properties
Transaction management is at the heart of any robust database system, and the TiDB database excels in this area by adhering to ACID properties. These properties—Atomicity, Consistency, Isolation, and Durability—ensure that all updates are processed reliably. Atomicity guarantees that each transaction is treated as a single unit, while consistency ensures that the database remains in a valid state before and after the update. Isolation prevents concurrent transactions from interfering with each other, and durability ensures that once a transaction is committed, it remains so even in the event of a system failure.
Concurrency Control
Concurrency control is vital for maintaining database performance, especially in high-traffic environments. The TiDB database employs sophisticated concurrency control mechanisms to manage simultaneous transactions effectively. By utilizing both optimistic and pessimistic locking strategies, TiDB ensures that updates are executed without unnecessary delays, allowing multiple users to interact with the database concurrently without compromising data integrity.
Specific SQL Statements
‘INSERT ON DUPLICATE KEY UPDATE’
The INSERT ON DUPLICATE KEY UPDATE
statement is a powerful tool within the TiDB database for handling situations where you need to insert new records or update existing ones based on unique key constraints. This command simplifies the process of managing duplicate entries and ensures that your data remains consistent and up-to-date without the need for complex conditional logic.
Use Cases and Examples
Consider a scenario where you need to update user ratings in a database. Using the INSERT ON DUPLICATE KEY UPDATE
statement, you can efficiently manage these updates:
INSERT INTO ratings (book_id, user_id, rating) VALUES (1000, 1, 5)
ON DUPLICATE KEY UPDATE rating = 5;
This example demonstrates how the TiDB database can handle both insertion and update operations in a single, streamlined command, reducing the complexity and improving the efficiency of your data management tasks.
By leveraging these key features, the TiDB database empowers organizations to execute the sql command to update
with unmatched efficiency, ensuring that their systems remain responsive and reliable even under heavy load.
Best Practices for Updating Multiple Rows
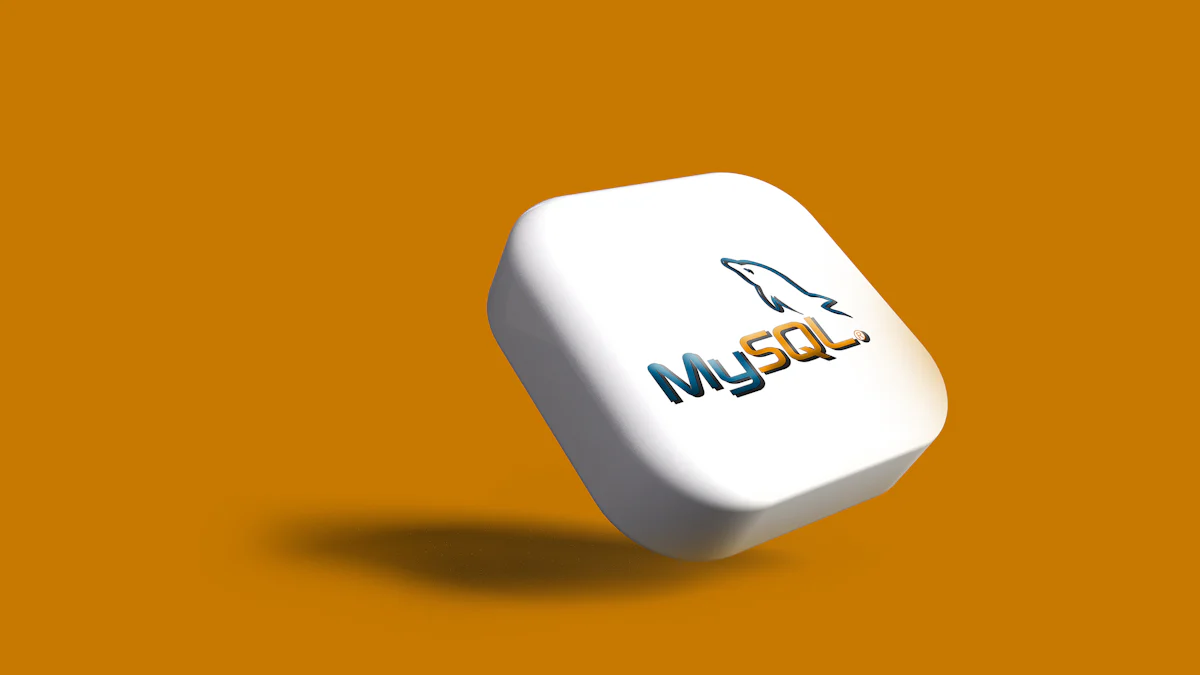
In the realm of database management, efficiently updating multiple rows is a critical skill that can significantly enhance performance and maintain data integrity. Here, we delve into practical examples and common pitfalls to avoid when using the sql command to update
.
Practical Examples
Code Snippets
Implementing efficient updates often involves crafting precise SQL commands. Below are some code snippets demonstrating effective techniques:
-
Bulk Update with CASE Expression: This method allows you to update multiple rows with different values in a single query, optimizing performance.
UPDATE employees SET salary = CASE WHEN department = 'HR' THEN salary * 1.05 WHEN department = 'IT' THEN salary * 1.10 ELSE salary END WHERE department IN ('HR', 'IT');
-
Using Joins for Updates: Sometimes, updates require data from another table. An
UPDATE JOIN
can be used to achieve this efficiently.UPDATE orders INNER JOIN customers ON orders.customer_id = customers.id SET orders.discount = 0.10 WHERE customers.loyalty_level = 'Gold';
These snippets illustrate how the sql command to update
can be tailored to handle complex scenarios, ensuring both efficiency and accuracy.
Step-by-Step Guide
For those new to SQL or seeking to refine their skills, here’s a step-by-step guide to executing a bulk update:
-
Identify the Target Rows: Use a
SELECT
statement to confirm which rows need updating. This prevents unintended changes.SELECT * FROM products WHERE category = 'Electronics';
-
Craft the Update Statement: Incorporate a
WHERE
clause to specify the exact rows for modification.UPDATE products SET price = price * 0.90 WHERE category = 'Electronics';
-
Test in a Safe Environment: Before applying the update to a live database, test it in a development or staging environment to ensure it behaves as expected.
-
Execute and Verify: Run the update and verify the changes with a follow-up
SELECT
query.SELECT * FROM products WHERE category = 'Electronics';
By following these steps, you ensure that your updates are both effective and safe, minimizing the risk of errors.
Common Mistakes to Avoid
Inefficient Queries
One of the most common pitfalls in using the sql command to update
is crafting inefficient queries that can slow down the database. Here are some tips to avoid this:
-
Avoid Full Table Scans: Always use a
WHERE
clause to limit the scope of your update. Without it, you risk updating every row in the table, which can be resource-intensive. -
Optimize Index Usage: Ensure that your queries leverage existing indexes to speed up the update process. If necessary, create new indexes to support frequent update operations.
Locking Issues
Locking issues can arise when updates hold locks on rows for extended periods, leading to performance bottlenecks. To mitigate this:
-
Batch Updates: Break large updates into smaller batches to reduce lock duration and improve concurrency.
-
Use Pessimistic Locking Sparingly: While pessimistic locking can prevent conflicts, it can also lead to deadlocks if not managed carefully. Consider optimistic locking where appropriate.
By understanding and avoiding these common mistakes, you can enhance the efficiency of your SQL updates, ensuring that your database remains responsive and reliable.
Use Cases Highlighting TiDB’s Advantages
In the ever-evolving world of database management, real-world applications and success stories provide invaluable insights into the capabilities of the TiDB database. By examining these scenarios, we can better understand how TiDB enhances performance and efficiency across various industries.
Real-World Scenarios
Industry Applications
The TiDB database has been embraced by numerous industries for its robust features and scalability. For instance, in the financial sector, where data consistency and reliability are paramount, TiDB’s high availability and strong consistency protocols ensure seamless transaction processing even during peak loads. This makes it an ideal choice for financial institutions that require rapid data updates without compromising accuracy.
In the e-commerce industry, TiDB’s horizontal scalability allows businesses to handle massive datasets efficiently. As online shopping platforms experience fluctuating traffic, especially during sales events, TiDB’s load balancing capabilities distribute queries evenly, maintaining optimal performance and user experience.
Customer Success Stories
One notable success story comes from Pinterest, a leading social media platform. After rigorous internal testing of various datastores, Pinterest selected the TiDB database due to its sustained performance and developer velocity. The decision was further validated through testing with shadow traffic, where TiDB demonstrated exceptional reliability and performance characteristics. This case study underscores TiDB’s ability to support high-demand environments with ease, making it a preferred choice for companies seeking to enhance their data infrastructure.
Reinforcing Benefits
Performance Gains
The performance gains achieved with the TiDB database are significant. By leveraging its distributed architecture, organizations can execute complex sql command to update
operations swiftly, reducing latency and improving system responsiveness. This is particularly beneficial for businesses that rely on real-time data processing and analytics, as TiDB ensures that updates are processed efficiently, keeping data current and actionable.
Cost Efficiency
Cost efficiency is another compelling advantage of the TiDB database. Its cloud-native design allows for flexible scaling, enabling businesses to expand their database infrastructure incrementally rather than investing in costly hardware upgrades. This approach not only optimizes resource allocation but also reduces operational expenses, providing a cost-effective solution for managing large-scale data operations.
By exploring these use cases and benefits, it’s clear that the TiDB database offers a powerful combination of performance, reliability, and cost efficiency, making it an invaluable asset for organizations across diverse sectors.
Efficient updates in SQL are not just a technical necessity but a cornerstone for maintaining robust database performance. By leveraging the advanced capabilities of the TiDB database, you can ensure that your data operations remain swift and reliable. TiDB’s distributed architecture, combined with its support for bulk updates and transaction management, offers unparalleled performance gains and cost efficiency. As you implement these practices, remember to double-check your WHERE
clauses and consider using temporary tables for complex updates. Embrace these strategies to optimize your database management and drive your business forward with confidence.