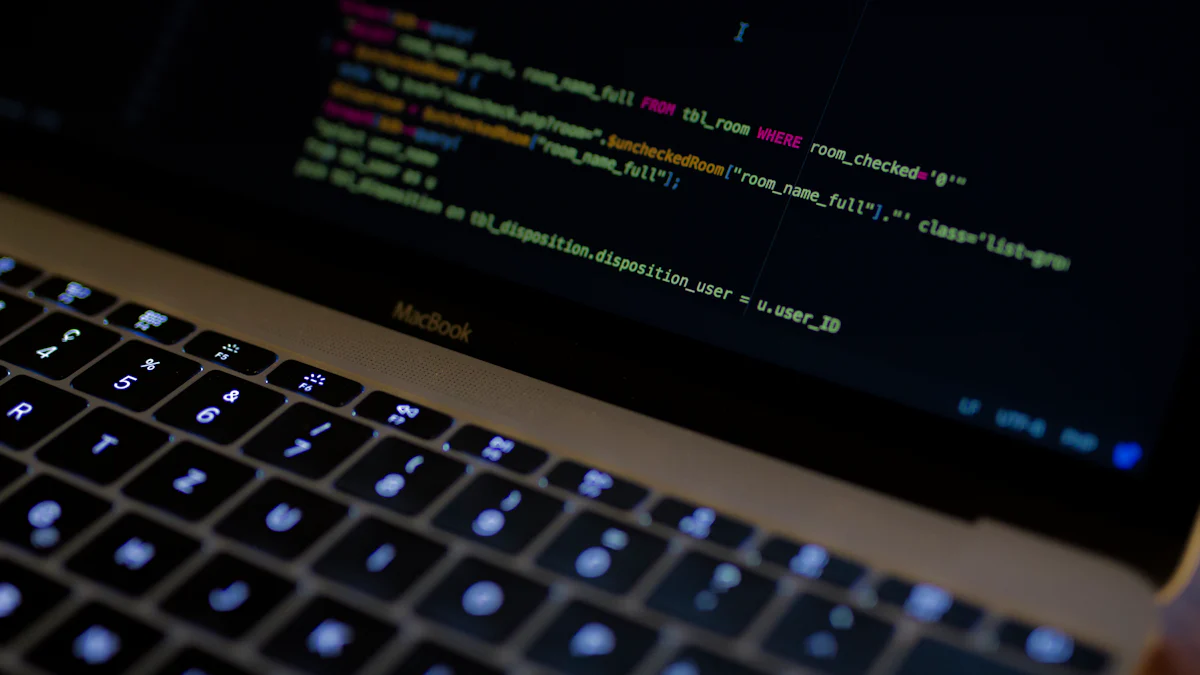
Managing columns in SQL databases is crucial for maintaining optimal performance and data organization. Proper column management can significantly impact database performance, as indexing and query optimization rely heavily on the strategic arrangement of columns. This blog focuses on the essential operations of sql add column drop column, which are fundamental for evolving database schemas and optimizing performance. These operations have practical applications in real-time reporting, data analysis, and ensuring efficient data retrieval.
Understanding SQL Columns
What are SQL Columns?
Definition and Role in Databases
SQL columns are fundamental components of a database table, representing the attributes or fields of the data stored within. Each column in a table is defined by a specific data type, such as INT
, VARCHAR
, or DATE
, which dictates the kind of data that can be stored in that column. The primary role of columns is to organize data into a structured format, enabling efficient storage, retrieval, and manipulation.
Columns interact with rows, where each row represents a single record in the table. This structure allows for systematic data organization, ensuring that related data points are stored together. For instance, in a customer database, columns might include CustomerID
, Name
, Email
, and DateOfBirth
, each holding specific pieces of information about the customers.
Importance of Column Management
Effective column management is crucial for several reasons:
- Data Integrity: Properly defined columns ensure that data entered into the database adheres to the expected format and constraints, maintaining the accuracy and reliability of the data.
- Performance Optimization: Columns play a significant role in query performance. Well-managed columns, especially those indexed appropriately, can expedite query responses and enhance overall database performance.
- Scalability: As databases grow, the ability to add or drop columns without disrupting operations is vital for scalability. This flexibility supports evolving business needs and data requirements.
- Data Analysis: Organized columns facilitate efficient data analysis and reporting, allowing for more accurate insights and decision-making.
Common Scenarios for Adding and Dropping Columns
Database Schema Evolution
In the dynamic landscape of database management, schemas often need to evolve to accommodate new requirements. Adding columns can introduce new data attributes without redesigning the entire database. Conversely, dropping obsolete columns can streamline the schema, reducing complexity and potential maintenance overhead.
For example, a retail business might start tracking customer preferences by adding a Preferences
column to their Customers
table. Over time, if this data becomes redundant or is tracked elsewhere, the column can be dropped to simplify the schema.
Performance Optimization
Columns are integral to performance optimization strategies. Indexing columns with high cardinality—columns with a large number of unique values—can significantly enhance query performance. Additionally, maintaining up-to-date statistics on these columns helps the database engine make informed decisions about query execution plans.
When optimizing joins, indexing the columns used in join conditions can drastically reduce the time required to locate and match records. For instance, indexing the CustomerID
column in both Orders
and Customers
tables can speed up queries that join these tables on CustomerID
.
Moreover, adding or dropping columns can directly impact the efficiency of data retrieval and storage. For instance, adding a column to store precomputed values can reduce the computational load during query execution, while dropping unused columns can free up storage space and reduce the size of the database.
Steps to Add Columns in SQL
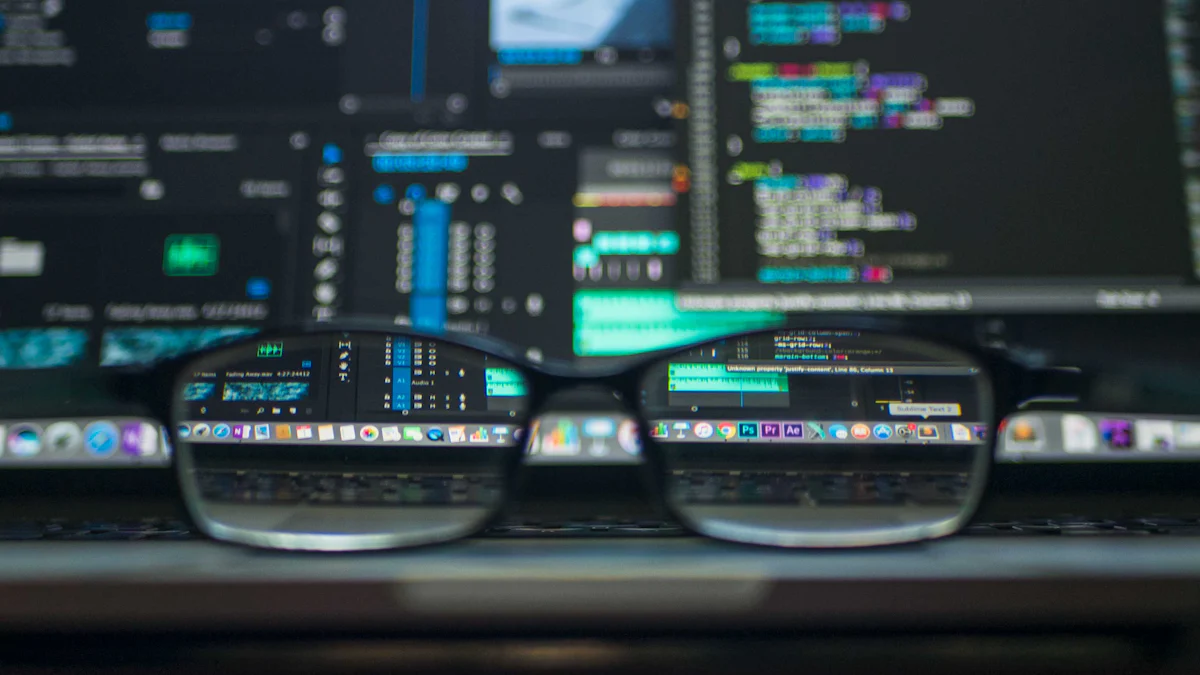
Basic Syntax for Adding Columns
Using the ALTER TABLE Statement
To add a column to an existing table, the ALTER TABLE
statement is employed. This command allows you to modify the structure of a table without disrupting its current data. The basic syntax for adding a column is straightforward:
ALTER TABLE table_name ADD COLUMN column_name column_type [column_options];
Here, table_name
is the name of the table to be modified, column_name
is the name of the new column, and column_type
specifies the data type of the new column. Optional parameters like column_options
can include constraints such as NOT NULL
, DEFAULT
, or UNIQUE
.
Example: Adding a Single Column
Consider a scenario where you need to add a column named age
of type INT
to a table called employees
. The SQL statement would look like this:
ALTER TABLE employees ADD COLUMN age INT;
This command will add the age
column to the employees
table, allowing you to store integer values representing the ages of employees.
Adding Multiple Columns
Syntax and Considerations
Adding multiple columns in a single operation can be more efficient than adding them one by one. The syntax for adding multiple columns is an extension of the single-column addition:
ALTER TABLE table_name
ADD COLUMN column1_name column1_type [column1_options],
ADD COLUMN column2_name column2_type [column2_options];
When adding multiple columns, it’s crucial to consider the order of columns and any dependencies between them. Proper planning ensures that the database schema remains logical and efficient.
Example: Adding Multiple Columns
Suppose you want to add two columns, department
(a VARCHAR
type) and hire_date
(a DATE
type), to the employees
table. The SQL statement would be:
ALTER TABLE employees
ADD COLUMN department VARCHAR(50),
ADD COLUMN hire_date DATE;
This command adds both department
and hire_date
columns to the employees
table in a single operation, streamlining the process and reducing potential downtime.
Adding Columns with Constraints
Types of Constraints (NOT NULL, UNIQUE, etc.)
Constraints are rules applied to columns to enforce data integrity and consistency. Common constraints include:
- NOT NULL: Ensures that the column cannot contain
NULL
values. - UNIQUE: Ensures that all values in the column are unique.
- PRIMARY KEY: A combination of
NOT NULL
andUNIQUE
, uniquely identifying each row in the table. - DEFAULT: Sets a default value for the column if no value is specified during insertion.
Applying these constraints when adding columns helps maintain the integrity and reliability of your data.
Example: Adding a Column with a NOT NULL Constraint
Let’s add a salary
column to the employees
table with a NOT NULL
constraint to ensure that every employee has a salary recorded. The SQL statement would be:
ALTER TABLE employees
ADD COLUMN salary DECIMAL(10, 2) NOT NULL;
This command adds the salary
column with a DECIMAL
data type, ensuring that it cannot contain NULL
values. By enforcing the NOT NULL
constraint, you guarantee that every record in the employees
table includes a valid salary entry.
Steps to Drop Columns in SQL
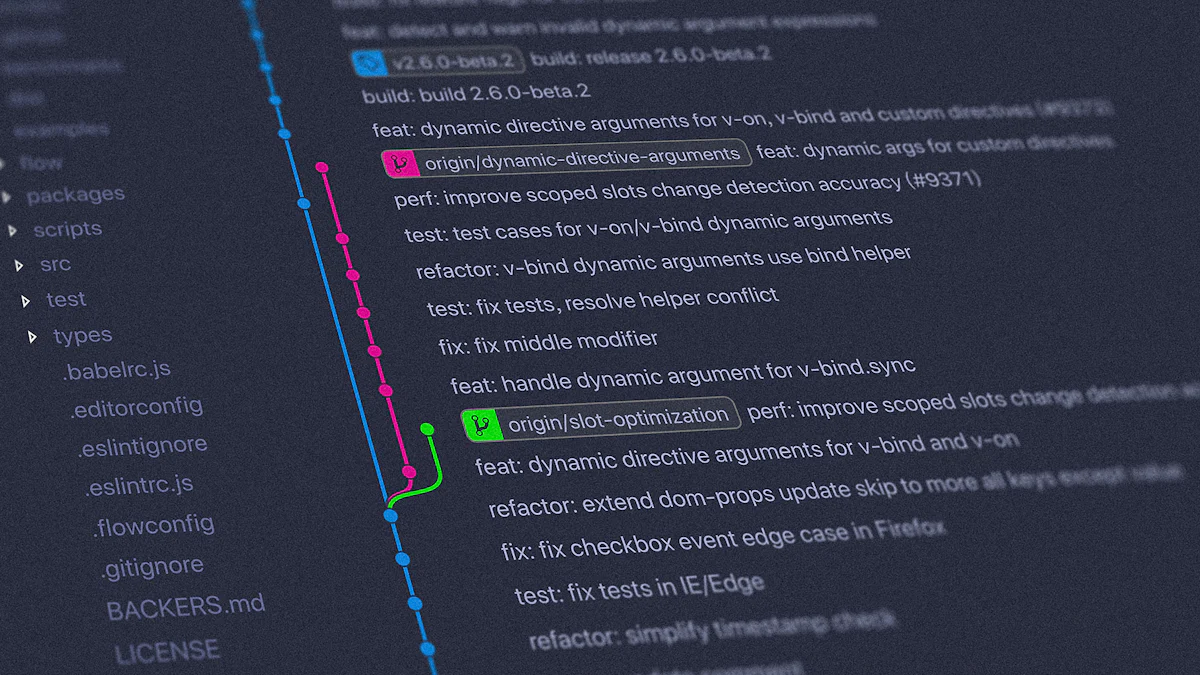
Efficiently managing the structure of your database involves not only adding columns but also knowing how to remove them when they are no longer needed. Dropping columns can help streamline your database schema, improve performance, and reduce storage requirements.
Basic Syntax for Dropping Columns
Using the ALTER TABLE Statement
To drop a column from an existing table, the ALTER TABLE
statement is used. This command allows you to modify the table structure by removing unnecessary columns without affecting the remaining data. The basic syntax for dropping a column is as follows:
ALTER TABLE table_name DROP COLUMN column_name;
Here, table_name
is the name of the table from which you want to remove the column, and column_name
is the name of the column to be dropped.
Example: Dropping a Single Column
Consider a scenario where you need to drop a column named age
from a table called employees
. The SQL statement would look like this:
ALTER TABLE employees DROP COLUMN age;
This command will remove the age
column from the employees
table, effectively eliminating any data stored in that column.
Dropping Multiple Columns
Syntax and Considerations
Dropping multiple columns in a single operation can be more efficient than dropping them one by one. The syntax for dropping multiple columns is an extension of the single-column drop:
ALTER TABLE table_name
DROP COLUMN column1_name,
DROP COLUMN column2_name;
When dropping multiple columns, it’s essential to consider the impact on the database schema and any dependencies that may exist. Proper planning ensures that the removal of columns does not disrupt the integrity or functionality of the database.
Example: Dropping Multiple Columns
Suppose you want to drop two columns, department
and hire_date
, from the employees
table. The SQL statement would be:
ALTER TABLE employees
DROP COLUMN department,
DROP COLUMN hire_date;
This command removes both department
and hire_date
columns from the employees
table in a single operation, streamlining the process and minimizing potential downtime.
Handling Dependencies and Constraints
Identifying Dependent Objects
Before dropping a column, it’s crucial to identify any dependent objects, such as indexes, foreign keys, or constraints, that rely on the column. Dropping a column without addressing these dependencies can lead to errors and data integrity issues. Tools and queries can help identify these dependencies, ensuring a smooth transition.
For instance, in TiDB database, you can use the SHOW CREATE TABLE
statement to review the table’s structure and identify any dependencies:
SHOW CREATE TABLE employees;
This command provides a detailed view of the table’s schema, including any constraints or indexes associated with the columns.
Example: Dropping a Column with Dependencies
Let’s consider a scenario where the salary
column in the employees
table is part of an index. Before dropping the column, you need to remove the index:
ALTER TABLE employees DROP INDEX salary_index;
ALTER TABLE employees DROP COLUMN salary;
By first removing the index, you ensure that dropping the salary
column does not cause any issues. This careful approach maintains the integrity and performance of your database.
By mastering the steps to add and drop columns, you can effectively manage your database schema, ensuring it evolves to meet changing requirements while maintaining optimal performance. Whether you’re using the sql add column drop column
operations for schema evolution or performance optimization, understanding these processes is crucial for efficient database management.
Best Practices and Considerations
When managing your database schema, it’s essential to follow best practices to ensure that changes are implemented smoothly and efficiently. This section outlines key considerations for planning, testing, and optimizing schema changes.
Planning Schema Changes
Impact Analysis
Before making any modifications to your database schema, conducting a thorough impact analysis is crucial. This involves understanding how the changes will affect existing applications, queries, and overall database performance. Key steps include:
- Reviewing Dependencies: Identify any objects, such as views, stored procedures, or triggers, that depend on the columns being added or dropped.
- Assessing Performance Impact: Evaluate how the changes might affect query performance, especially for large datasets or frequently accessed tables.
- Consulting Stakeholders: Communicate with developers, data analysts, and other stakeholders to ensure that the changes align with business requirements and do not disrupt ongoing operations.
Backup Strategies
Implementing a robust backup strategy is essential to safeguard your data before making any schema changes. This ensures that you can quickly restore your database to its previous state in case of any issues. Recommended practices include:
- Full Backups: Perform a full backup of the database before making any significant schema changes.
- Incremental Backups: Use incremental backups to capture changes made since the last full backup, minimizing downtime and storage requirements.
- Automated Backups: Schedule automated backups to ensure regular data protection without manual intervention.
Testing Changes
Using Staging Environments
Testing schema changes in a staging environment before applying them to the production database is a best practice that helps identify potential issues early. A staging environment replicates the production setup, allowing you to:
- Validate Changes: Ensure that the schema modifications work as expected and do not introduce errors.
- Test Performance: Assess the impact of the changes on query performance and overall database efficiency.
- Simulate Workloads: Run typical workloads and queries to verify that the changes do not negatively affect application functionality.
Example: Testing Column Additions and Deletions
Consider a scenario where you need to add a new column and drop an existing one in the employees
table. In the staging environment, you would:
- Add the New Column:
ALTER TABLE employees ADD COLUMN department VARCHAR(50);
- Verify the Addition: Check that the new column has been added correctly and test inserting data into it.
- Drop the Existing Column:
ALTER TABLE employees DROP COLUMN age;
- Verify the Deletion: Ensure that the column has been removed and that no dependent objects are affected.
By following these steps, you can confidently apply the changes to the production database, knowing that they have been thoroughly tested.
Performance Implications
Index Management
Indexes play a critical role in database performance, especially when adding or dropping columns. Proper index management ensures that your database remains efficient and responsive. Key considerations include:
- Updating Indexes: When adding a new column that will be frequently queried, consider creating an index to improve query performance.
- Removing Unused Indexes: Dropping a column may render some indexes obsolete. Remove these indexes to free up resources and maintain optimal performance.
- Monitoring Index Usage: Regularly monitor index usage to identify and address any performance bottlenecks.
Query Optimization
Optimizing queries is essential for maintaining high performance, particularly after making schema changes. Effective query optimization involves:
- Analyzing Query Plans: Use tools like
EXPLAIN
to analyze query execution plans and identify areas for improvement. - Refactoring Queries: Simplify complex queries and ensure that they leverage indexes effectively.
- Maintaining Statistics: Keep database statistics up-to-date to help the query optimizer make informed decisions.
By adhering to these best practices and considerations, you can ensure that your database schema evolves smoothly, maintaining both performance and data integrity. Whether you’re performing sql add column drop column
operations or more complex schema modifications, careful planning and testing are key to successful database management.
Mastering column management in SQL is a critical skill for any database professional. By understanding and practicing the steps to add and drop columns, you can ensure your database schema evolves smoothly and efficiently. This knowledge not only enhances data integrity and performance but also prepares you for more advanced topics in SQL. We encourage you to apply these techniques in your projects and explore further learning opportunities to deepen your expertise in database management.
See Also
Transforming MySQL Database Communication through Text-to-SQL and LLMs
The Importance of Database Schema in SQL Data Handling
Guiding SQL Partitioning for Optimizing Databases
Transitioning Away from MySQL: 5 Vital Factors for Scalability and Performance
Achieving Accuracy in SQL: Proficiency with the Decimal Data Type