
Mastering SQL schema creation is crucial for ensuring efficient and robust database performance. A well-designed SQL schema acts as a blueprint, enforcing rules and relationships that maintain data integrity without additional application code. This proficiency not only prevents performance bottlenecks but also empowers professionals to extract valuable insights and drive informed business decisions. In real-world scenarios, the ability to design effective schemas is indispensable, as it supports complex systems and enhances the overall functionality of databases like the TiDB database, which excels in handling large-scale data with ease.
Prerequisites for SQL Schema Creation
Before diving into the intricacies of SQL schema creation, it’s essential to establish a solid foundation. This section outlines the prerequisites necessary to effectively create and manage SQL schemas, focusing on understanding the SQL Server environment and acquiring basic SQL knowledge.
Understanding SQL Server Environment
To proficiently create and manage SQL schemas, familiarity with the SQL Server environment is crucial. This involves understanding the installation and setup processes, as well as becoming comfortable with the tools used for database management.
Installation and Setup
Setting up a SQL Server environment begins with installing Microsoft SQL Server 2019. This version offers robust features that support efficient schema creation and management. The installation process is straightforward, typically involving downloading the installer from Microsoft’s official website, running the setup, and following the on-screen instructions to configure the server according to your needs. Ensuring that your system meets the necessary requirements for SQL Server 2019 is vital for a smooth installation experience.
Familiarity with SQL Server Management Studio (SSMS)
Once the server is installed, the next step is to become acquainted with SQL Server Management Studio (SSMS), a powerful tool for managing SQL Server instances. SSMS provides a user-friendly interface for executing queries, designing databases, and managing security settings. Version 18.9.2 is particularly recommended for its enhanced features and improved performance. Familiarizing yourself with SSMS will enable you to efficiently navigate through various tasks involved in schema creation, such as defining tables, setting permissions, and optimizing queries.
Basic SQL Knowledge
A fundamental understanding of SQL is indispensable for anyone looking to master schema creation. This includes knowledge of key SQL commands and a grasp of essential database concepts.
Key SQL Commands
Proficiency in SQL commands forms the backbone of effective schema management. Key commands include CREATE
, ALTER
, and DROP
, which are used to define, modify, and delete database objects, respectively. Additionally, understanding how to use SELECT
for querying data, INSERT
for adding new records, UPDATE
for modifying existing data, and DELETE
for removing records is essential. Mastery of these commands allows for precise control over database operations, ensuring that schemas are both functional and efficient.
Understanding Database Concepts
Beyond commands, a solid grasp of database concepts is necessary. This includes understanding the role of schemas as logical containers for database objects like tables, views, and indexes. Recognizing the importance of data integrity, relationships, and constraints is also critical. These concepts ensure that data is organized logically, making it easier to maintain and query. By comprehending these foundational elements, you can design schemas that not only meet current requirements but are also scalable and adaptable to future needs.
Creating a SQL Schema with TiDB
Creating a SQL schema in the TiDB database is a streamlined process that leverages its compatibility with MySQL, allowing for efficient management of large-scale data. This section provides a detailed guide to creating a SQL schema in TiDB, followed by a practical example to illustrate the process.
Step-by-Step Guide
Accessing TiDB
To begin creating a SQL schema, you first need to access the TiDB database environment. This can be done through the TiDB Cloud Console or by connecting directly to a TiDB cluster using a MySQL-compatible client such as MySQL Workbench or the command line interface. Ensure that you have the necessary permissions to create schemas within the database.
Navigating to Schema Creation
Once connected, navigate to the schema creation interface. In the TiDB Cloud Console, this involves selecting the appropriate database and accessing the schema management section. If using a command line interface, you can start by selecting the database where you want to create the schema using the USE
command.
Defining Schema Properties
Defining the properties of your SQL schema is a crucial step. This includes specifying the schema name and defining the tables, columns, and data types that will be part of the schema. In TiDB, you can use standard SQL commands to define these properties. For instance, the CREATE TABLE
command allows you to specify column names, data types, and constraints such as primary keys and foreign keys, ensuring data integrity and efficient retrieval.
Practical Example
Creating a Sample Schema
Let’s walk through a practical example of creating a sample SQL schema in TiDB. Suppose we are designing a schema for an e-commerce application. We would start by creating a database and then define tables for products, customers, and orders.
-- Create a new database
CREATE DATABASE ecommerce;
-- Use the newly created database
USE ecommerce;
-- Create a table for products
CREATE TABLE products (
product_id INT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
price DECIMAL(10, 2),
stock INT
);
-- Create a table for customers
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
first_name VARCHAR(100),
last_name VARCHAR(100),
email VARCHAR(255) UNIQUE
);
-- Create a table for orders
CREATE TABLE orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
FOREIGN KEY (customer_id) REFERENCES customers(customer_id)
);
This example demonstrates how to set up a basic SQL schema with tables that include primary keys and foreign key relationships, which are essential for maintaining data consistency and integrity.
Assigning Permissions
After creating the schema, it’s important to assign appropriate permissions to ensure secure access. TiDB supports granular access control, allowing you to grant specific privileges to users. For instance, you can allow a user to only read data from the products
table while granting another user full access to manage the orders
table.
-- Grant select permission on products table to a user
GRANT SELECT ON products TO 'read_user'@'localhost';
-- Grant all permissions on orders table to an admin user
GRANT ALL PRIVILEGES ON orders TO 'admin_user'@'localhost';
By carefully managing permissions, you can protect sensitive data and ensure that users have the access they need to perform their roles effectively.
Creating a SQL schema in TiDB not only enhances data organization but also optimizes performance, making it a powerful tool for businesses handling complex datasets. The flexibility and scalability of the TiDB database make it an ideal choice for modern applications requiring robust data management solutions.
Creating a Schema using T-SQL
Creating a SQL schema using T-SQL (Transact-SQL) is a fundamental skill for database professionals. T-SQL, an extension of SQL used in Microsoft SQL Server, allows you to define and manipulate data with precision. This section will guide you through the process of creating a schema using T-SQL, providing practical insights and examples.
Step-by-Step Guide
Writing T-SQL Commands
To begin crafting a SQL schema, it’s essential to understand the syntax and structure of T-SQL commands. A typical schema creation involves defining tables, columns, data types, and constraints. Here’s a basic example of how to write T-SQL commands for schema creation:
-- Create a new schema
CREATE SCHEMA Sales;
-- Create a table within the schema
CREATE TABLE Sales.Products (
ProductID INT PRIMARY KEY,
ProductName NVARCHAR(100) NOT NULL,
Price DECIMAL(10, 2),
StockQuantity INT CHECK (StockQuantity >= 0)
);
In this example, the CREATE SCHEMA
command establishes a new schema named Sales
. Within this schema, a table Products
is created with several columns, including constraints like PRIMARY KEY
and CHECK
to ensure data integrity.
Executing Commands in SQL Server
Once you’ve written your T-SQL commands, the next step is execution. This can be done using SQL Server Management Studio (SSMS) or any other SQL Server-compatible client. Simply open a new query window, paste your T-SQL script, and execute it. Successful execution will result in the creation of your defined schema and its associated objects.
Practical Example
Creating a Sample Schema with T-SQL
Let’s delve into a more comprehensive example that illustrates creating a SQL schema for a library management system. This example will highlight the use of T-SQL to define multiple tables and relationships:
-- Create a new schema
CREATE SCHEMA Library;
-- Create a table for books
CREATE TABLE Library.Books (
BookID INT PRIMARY KEY,
Title NVARCHAR(255) NOT NULL,
Author NVARCHAR(255),
PublishedYear INT
);
-- Create a table for members
CREATE TABLE Library.Members (
MemberID INT PRIMARY KEY,
FirstName NVARCHAR(100),
LastName NVARCHAR(100),
Email NVARCHAR(255) UNIQUE
);
-- Create a table for loans
CREATE TABLE Library.Loans (
LoanID INT PRIMARY KEY,
BookID INT,
MemberID INT,
LoanDate DATE,
ReturnDate DATE,
FOREIGN KEY (BookID) REFERENCES Library.Books(BookID),
FOREIGN KEY (MemberID) REFERENCES Library.Members(MemberID)
);
This example demonstrates the creation of a Library
schema with tables for Books
, Members
, and Loans
. It showcases the use of foreign keys to establish relationships between tables, ensuring referential integrity.
Modifying Schema Properties
After creating a schema, modifications may be necessary to adapt to evolving requirements. T-SQL provides flexibility for altering schema properties. For instance, you might need to add a new column or modify an existing one:
-- Add a new column to the Books table
ALTER TABLE Library.Books
ADD Genre NVARCHAR(100);
-- Modify the data type of the PublishedYear column
ALTER TABLE Library.Books
ALTER COLUMN PublishedYear SMALLINT;
These commands illustrate how to extend the functionality of your schema by adding new attributes or adjusting existing ones, ensuring that your database remains aligned with business needs.
Creating a SQL schema using T-SQL empowers database professionals to design robust and efficient data architectures. By leveraging T-SQL’s capabilities, you can ensure that your schemas are well-structured and capable of supporting complex data requirements.
Best Practices for SQL Schema Creation
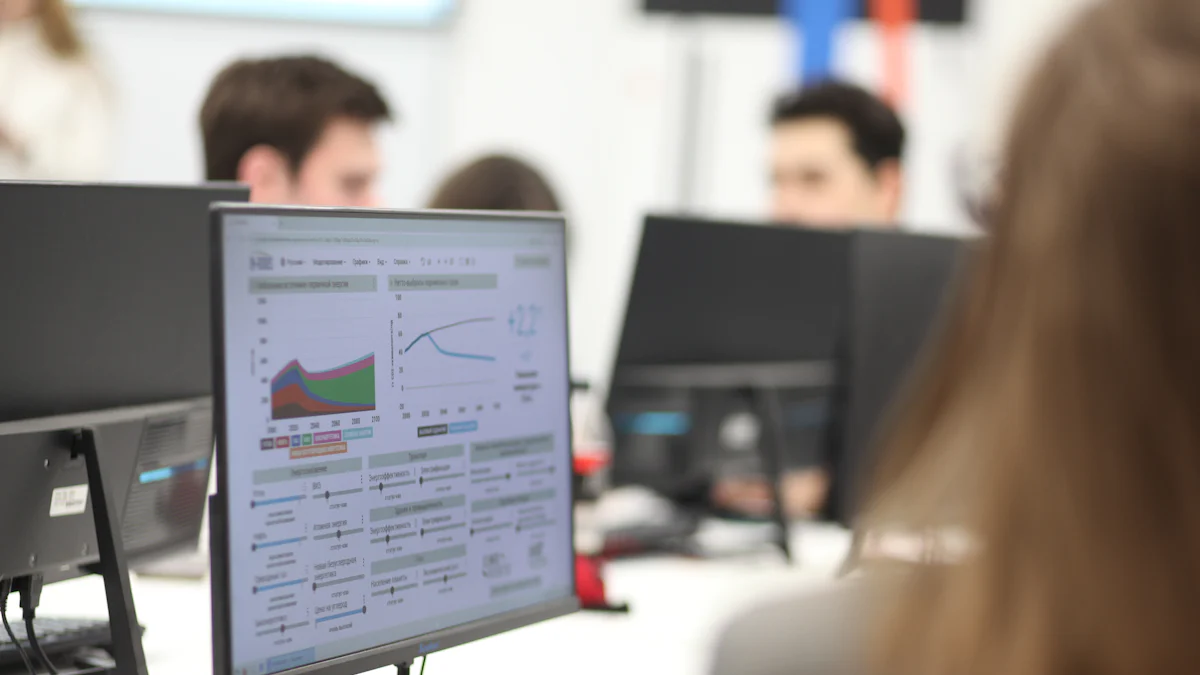
Creating a robust and efficient SQL schema is a fundamental aspect of database management. By adhering to best practices, you can ensure data integrity, enhance security, and optimize performance. This section delves into essential guidelines for naming conventions and security considerations in SQL schema creation.
Naming Conventions
Consistency and Clarity
Naming conventions play a pivotal role in maintaining a clear and organized database structure. Consistent naming helps developers and database administrators understand the purpose and function of each database object at a glance. Here are some key points to consider:
-
Use Descriptive Names: Ensure that table and column names clearly describe their content or purpose. For instance, instead of using generic names like
tbl1
orcolA
, opt for descriptive alternatives such asCustomerOrders
orOrderDate
. -
Follow a Standard Format: Adopt a standard naming format across your database. This could involve using prefixes to denote object types (e.g.,
tbl_
for tables,vw_
for views) or employing camelCase or snake_case for multi-word identifiers. -
Avoid Reserved Keywords: Refrain from using SQL reserved keywords as object names to prevent conflicts and errors during query execution.
-
Be Consistent Across the Database: Consistency in naming conventions aids in understanding and managing the database more efficiently. This practice also facilitates collaboration among team members by providing a common framework for database object identification.
By implementing these naming conventions, you create a logical and intuitive structure that simplifies database operations and reduces the likelihood of errors.
Security Considerations
Managing User Permissions
Security is a paramount concern in database management, and controlling user permissions is a critical aspect of safeguarding your data. Properly managing permissions ensures that only authorized users have access to sensitive information and can perform specific actions within the database.
-
Principle of Least Privilege: Grant users the minimum level of access necessary to perform their tasks. This reduces the risk of accidental or malicious data manipulation and enhances overall security.
-
Role-Based Access Control (RBAC): Implement RBAC to streamline permission management. By assigning roles with predefined permissions to users, you can efficiently manage access rights and ensure consistency across the organization.
-
Regularly Review and Audit Permissions: Periodically review user permissions to ensure they align with current roles and responsibilities. Conducting audits helps identify and rectify any unauthorized access or outdated permissions.
-
Use Information Schema Views: Leverage information schema views to gain insights into the metadata of database objects. These views provide valuable information about user permissions, object dependencies, and schema structures, aiding in effective security management.
By adhering to these security best practices, you can protect your database from unauthorized access and maintain the integrity and confidentiality of your data.
Incorporating these best practices into your SQL schema creation process not only enhances the efficiency and security of your database but also lays a solid foundation for future scalability and adaptability. By prioritizing consistency, clarity, and security, you ensure that your database remains robust and reliable in the face of evolving business needs.
Troubleshooting Common Issues
Creating and managing SQL schemas can be a complex endeavor, often accompanied by challenges that require thoughtful troubleshooting. Understanding common issues and their solutions is essential for maintaining a robust database environment. This section delves into error handling and performance optimization, providing insights and practical tips to enhance your schema design.
Error Handling
Common Errors and Solutions
In the realm of SQL schema creation, errors are inevitable. However, with the right approach, they can be effectively managed and resolved. Here are some common errors you might encounter and their corresponding solutions:
-
Syntax Errors: These occur when there is a mistake in the SQL command syntax. To resolve this, carefully review your SQL statements for typos or misplaced keywords. Utilizing tools like SQL Server Management Studio (SSMS) can help identify syntax errors quickly.
-
Constraint Violations: When data does not adhere to the defined constraints, such as primary keys or foreign keys, errors arise. Ensure that the data being inserted or updated complies with the schema’s constraints. Implementing checks and validations within your application logic can prevent these violations.
-
Permission Denied: This error occurs when a user attempts to perform an action without the necessary permissions. Regularly audit and update user permissions to align with their roles and responsibilities. Using role-based access control (RBAC) can streamline permission management.
-
Deadlocks: These happen when two or more transactions block each other, leading to a standstill. To mitigate deadlocks, ensure that transactions acquire locks in a consistent order and keep transaction durations short.
“The real pain with schema design is you may not realize the cost of your mistakes until it’s too late to fix them.” – A common sentiment among developers, highlighting the importance of proactive error handling.
Performance Optimization
Tips for Efficient Schema Design
Optimizing schema performance is crucial for ensuring that your database operates smoothly and efficiently. Here are some strategies to enhance your schema design:
-
Indexing: Proper indexing can significantly improve query performance by reducing the amount of data scanned. Identify frequently queried columns and create indexes accordingly. However, be mindful of over-indexing, which can lead to increased storage and maintenance overhead.
-
Normalization: Normalize your database to eliminate redundancy and ensure data integrity. However, balance normalization with performance needs, as overly normalized schemas can lead to complex queries and slower performance.
-
Partitioning: For large tables, consider partitioning to distribute data across multiple storage units. This can enhance query performance by allowing parallel processing and reducing I/O contention.
-
Caching: Implement caching mechanisms to store frequently accessed data in memory. This reduces the need to repeatedly query the database, improving response times.
-
Monitoring and Tuning: Regularly monitor database performance using tools like SQL Profiler or Performance Monitor. Analyze query execution plans to identify bottlenecks and optimize them through query rewriting or index adjustments.
By incorporating these practices into your schema design process, you can create a resilient and high-performing database environment. Remember, a well-optimized schema not only enhances performance but also supports scalability and adaptability, ensuring your database remains robust in the face of evolving business demands.
In mastering SQL schema creation, we’ve explored the essential building blocks that ensure robust database performance. From understanding prerequisites to implementing best practices, each step is crucial for crafting efficient schemas. Remember, the design of your database schema should be meticulously planned before construction begins, as it forms the backbone of your data architecture. We encourage you to continue exploring and practicing these concepts, as real-world application will deepen your expertise. Ultimately, mastering SQL schema creation empowers you to harness the full potential of databases like the TiDB database, driving innovation and informed decision-making in your projects.