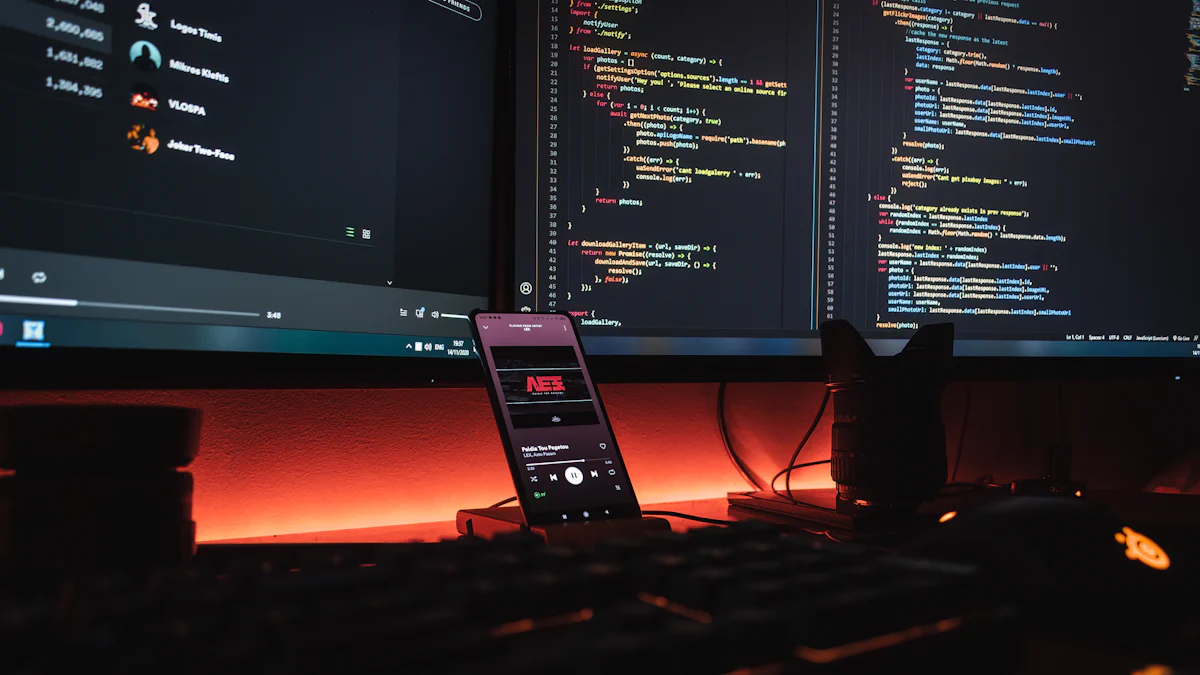
In the constantly changing realm of software development, effective dependency management plays a vital role in constructing resilient applications. The emergence of Golang modules has transformed this procedure in contemporary Go development. Introduced in version 1.11, Golang modules have evolved into the primary system for handling dependencies, streamlining tasks such as installation and version control. Recent statistics reveal that 87% of Go developers exclusively depend on this system, demonstrating its extensive acceptance. Through a simplified approach, Golang modules tackle past intricacies, empowering developers to concentrate on innovation rather than dependency challenges.
Understanding Golang Modules
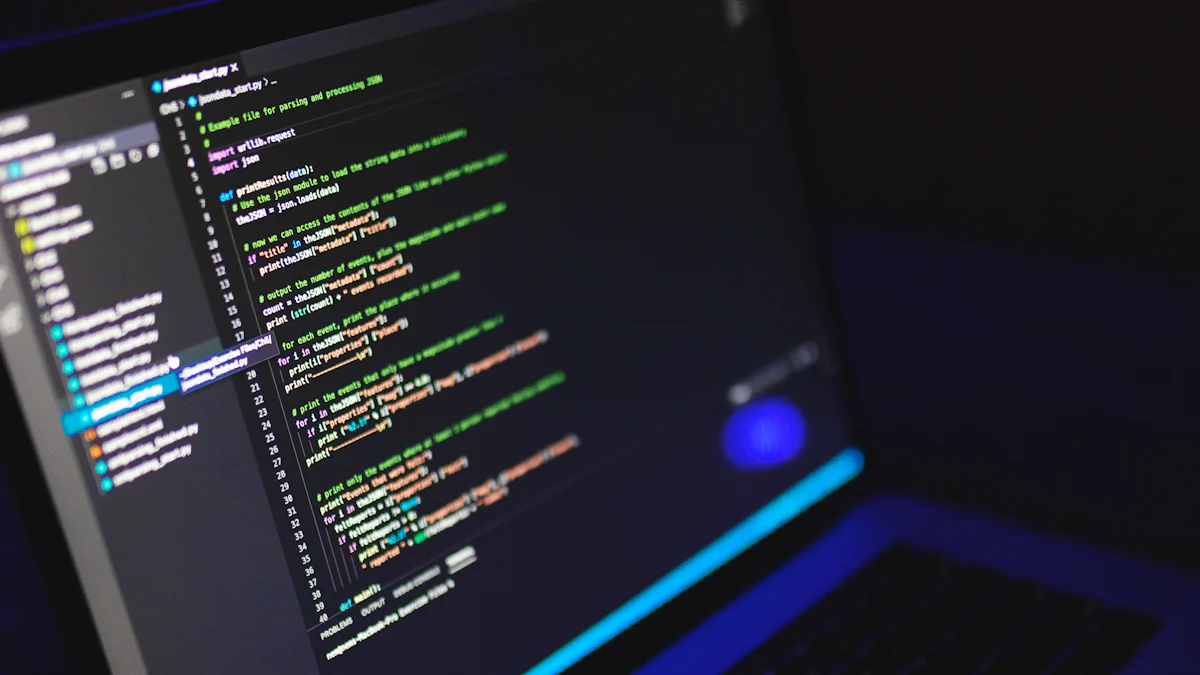
In the ever-evolving world of software development, managing dependencies efficiently is crucial for building robust applications. Golang modules have emerged as a pivotal tool in this regard, offering developers a streamlined approach to handling dependencies. Let’s delve deeper into what makes Golang modules an essential component of modern Go development.
What are Golang Modules?
Definition and Purpose
Golang modules are a collection of Go packages stored in a directory with a go.mod
file at its root. This file defines the module’s path, its dependencies, and the versions of those dependencies. The primary purpose of a golang module is to provide a clear and explicit way to manage dependencies, ensuring that projects are built with the correct versions of external packages. By doing so, it eliminates the ambiguity and potential conflicts that can arise from dependency management.
Historical Context and Evolution
Introduced in Go 1.11, golang modules marked a significant shift from the previous GOPATH
system. Before modules, developers relied heavily on vendoring, which often led to complex and cumbersome dependency trees. With the introduction of golang modules, developers gained the ability to specify exact versions of dependencies, thereby enhancing project reliability. The evolution continued with Go 1.13, which further refined module functionality, making it easier for developers to maintain and organize their projects.
Key Features of Golang Modules
Versioning and Compatibility
One of the standout features of golang modules is their robust versioning system. By adhering to semantic versioning, modules ensure backward compatibility and prevent breaking changes. This system allows developers to confidently upgrade dependencies, knowing that their applications will remain stable. The go.mod
file plays a crucial role here, explicitly listing the required versions of each dependency, thus maintaining consistency across different environments.
Dependency Resolution
Golang modules excel in simplifying dependency resolution. The go get
command automates the process of fetching and updating dependencies, ensuring that the correct versions are used. This automation reduces the manual effort required in managing dependencies, allowing developers to focus more on coding and less on configuration. Furthermore, the go.sum
file acts as a checksum for dependencies, adding an extra layer of security by verifying the integrity of downloaded packages.
Setting Up Golang Modules
Initializing a Module
Setting up a golang module is straightforward. By running the go mod init <module-name>
command, developers can initialize a new module. This command creates a go.mod
file, which serves as the blueprint for the module’s dependencies. It’s essential to place this file at the root of the project to ensure seamless dependency management.
Managing Module Files
Once a module is initialized, managing its files becomes a critical task. The go.mod
file should be regularly updated to reflect any changes in dependencies. Developers can use the go mod tidy
command to clean up unused dependencies, ensuring that the go.mod
file remains concise and accurate. Additionally, the go mod verify
command can be employed to check the integrity of the module’s dependencies, safeguarding against potential security vulnerabilities.
By understanding and leveraging the capabilities of golang modules, developers can significantly enhance their workflow, leading to more efficient and reliable applications. As the backbone of dependency management in Go, these modules empower developers to build with confidence, knowing that their projects are supported by a robust and flexible system.
Working with Dependencies in Golang Modules
Efficiently managing dependencies is a cornerstone of successful software development, and golang modules provide a robust framework to achieve this. This section delves into the practical aspects of adding, updating, and resolving dependencies within the golang module system, ensuring your projects remain stable and up-to-date.
Adding and Removing Dependencies
Using go get
Command
The go get
command is a powerful tool for adding dependencies to your golang module. By executing go get <package-path>
, you can easily fetch and install the desired package along with its dependencies. This command not only adds the package to your project but also updates the go.mod
file to reflect the new dependency, ensuring that your project remains consistent across different environments.
For instance, if you’re integrating the TiDB database into your Go application, you would use:
go get github.com/pingcap/tidb
This command retrieves the latest version of the TiDB database package, making it ready for use in your project.
Updating and Removing Dependencies
Keeping your dependencies current is crucial for maintaining security and performance. To update a dependency, simply run go get -u <package-path>
. This command updates the specified package to the latest version, while also updating the go.mod
file accordingly.
Removing a dependency is equally straightforward. After deleting the import statements from your code, you can run go mod tidy
to clean up unused dependencies from the go.mod
file. This ensures that your project remains lean and free from unnecessary packages.
Handling Version Conflicts
Understanding Semantic Versioning
Golang modules leverage semantic versioning to manage dependencies effectively. This versioning system uses a three-part number format: major.minor.patch
. Understanding this format is essential for resolving conflicts, as it helps you determine compatibility between different versions of a package.
- Major: Incompatible API changes.
- Minor: Backward-compatible functionality.
- Patch: Backward-compatible bug fixes.
By adhering to this system, golang modules ensure that developers can upgrade dependencies without fear of breaking their applications.
Resolving Conflicts
Version conflicts can arise when different packages require incompatible versions of the same dependency. Golang modules address this issue by allowing developers to specify precise versions in the go.mod
file. If a conflict occurs, you can manually edit the go.mod
file to specify a compatible version or use the replace
directive to substitute one version for another.
For example, if two packages require different versions of a library, you might add a line like this to your go.mod
file:
replace example.com/old => example.com/new v1.2.3
This directive tells the golang module system to use the specified version, resolving the conflict and ensuring that your project builds correctly.
By mastering these techniques, developers can harness the full potential of golang modules, creating applications that are both resilient and scalable. The ability to manage dependencies with precision and ease is a testament to the power and flexibility of golang modules, making them an indispensable tool in modern Go development.
Advanced Golang Module Techniques
As developers delve deeper into the capabilities of the golang module system, they uncover advanced techniques that enhance flexibility and control over their projects. This section explores the strategic use of replace and exclude directives, as well as managing private modules and configuring proxy servers, to optimize dependency management.
Using Replace and Exclude Directives
Purpose and Use Cases
The replace
and exclude
directives in a golang module offer powerful ways to manage dependencies. The replace
directive allows developers to substitute one module version with another, which is particularly useful when dealing with version conflicts or testing local changes without altering the original package. On the other hand, the exclude
directive prevents specific versions of a module from being used, ensuring that incompatible or undesirable versions do not affect your project.
These directives are invaluable in scenarios where you need precise control over the versions of dependencies, such as when integrating cutting-edge features from a development branch or avoiding known issues in certain versions.
Examples of Usage
To illustrate, suppose you’re working on a project that relies on a specific version of a library, but a newer version introduces breaking changes. You can use the replace
directive in your go.mod
file like this:
replace example.com/old => example.com/old v1.2.3
This line ensures that your golang module uses version v1.2.3
, even if a newer version is available.
Similarly, if a particular version of a dependency is causing issues, you can exclude it:
exclude example.com/problematic v1.4.0
This directive prevents version v1.4.0
from being used, safeguarding your project from potential disruptions.
Private Modules and Proxy Servers
Accessing Private Repositories
In many professional environments, accessing private repositories is essential for maintaining proprietary codebases. Golang modules facilitate this by allowing developers to configure authentication credentials for private repositories. By setting up environment variables such as GOPRIVATE
, you can ensure that your golang module can securely access private dependencies without exposing sensitive information.
For instance, to access a private repository hosted on GitHub, you might set the following environment variable:
export GOPRIVATE=github.com/yourcompany/*
This configuration instructs the golang module system to treat any repository under github.com/yourcompany
as private, streamlining access while maintaining security.
Configuring Proxy Servers
Proxy servers play a crucial role in optimizing dependency management, especially in environments with restricted internet access or when caching frequently used packages. By configuring a proxy server, you can enhance the performance and reliability of your golang module operations.
To set up a proxy server, you can define the GOPROXY
environment variable. For example:
export GOPROXY=https://proxy.golang.org,direct
This setup directs the golang module system to use the specified proxy server, falling back to direct access if the proxy is unavailable. This approach not only speeds up dependency resolution but also provides a layer of redundancy.
By mastering these advanced techniques, developers can wield the full power of the golang module system, tailoring it to meet the unique demands of their projects. Whether resolving complex dependency issues or optimizing access to private resources, these strategies ensure that your applications remain robust and adaptable.
Best Practices for Golang Module Management
In the realm of Go development, maintaining a clean and efficient module management system is crucial for ensuring project stability and scalability. By adhering to best practices, developers can streamline their workflows and enhance the reliability of their applications.
Maintaining a Clean go.mod
File
A well-maintained go.mod
file is the cornerstone of effective dependency management in Go projects. Here are some strategies to keep it organized:
Regular Updates and Pruning
Regularly updating your dependencies is essential for leveraging the latest features and security patches. The go get -u
command facilitates this by fetching the newest versions of packages. However, it’s equally important to prune unused dependencies to prevent bloat and potential conflicts. The go mod tidy
command is invaluable here, as it cleans up unnecessary entries in the go.mod
file, ensuring that only actively used packages are listed.
Keeping Track of Changes
Maintaining a record of changes in your dependencies is vital for troubleshooting and auditing purposes. Consider using version control systems like Git to track modifications to your go.mod
file. This practice not only aids in identifying the source of issues but also provides a historical context for dependency updates, making it easier to revert changes if necessary.
Expert Insight: Bartlomiej, a specialist in Go Modules, emphasizes the importance of adopting Go modules for their innovative features, such as advanced security and reliable installations. He suggests, “If you don’t use it, I recommend switching to it as there are many innovations that work on top of Go modules.”
Leveraging Go Tools for Optimization
Go provides a suite of tools designed to optimize module management, enhancing both efficiency and security.
Using go mod tidy
The go mod tidy
command is a powerful tool for optimizing your module files. It automatically removes unused dependencies and adds any missing ones, ensuring that your go.mod
file accurately reflects the current state of your project. This not only keeps your project lean but also reduces the risk of dependency-related issues.
Employing go mod verify
Security is a paramount concern in software development, and the go mod verify
command plays a critical role in safeguarding your projects. By verifying the checksums of your dependencies against those recorded in the go.sum
file, this command ensures that no unauthorized changes have been made to the packages you rely on. This verification process adds an extra layer of security, protecting your application from potential vulnerabilities.
By implementing these best practices, developers can harness the full potential of Go modules, creating robust and scalable applications. The combination of regular maintenance and strategic use of Go tools ensures that your projects remain efficient and secure, allowing you to focus on innovation and development.
Integrating Golang Modules with PingCAP’s TiDB
Integrating the TiDB database with golang modules opens up a realm of possibilities for developers seeking enhanced scalability and performance in their applications. This section explores the benefits and practical implementation of using TiDB with golang modules, providing insights into how this combination can revolutionize your development process.
Benefits of Using TiDB with Golang Modules
Enhanced Scalability and Performance
The TiDB database is renowned for its ability to scale horizontally, making it an ideal choice for applications that require robust performance under heavy loads. By leveraging golang modules, developers can effortlessly manage dependencies and integrate the TiDB database into their projects. This seamless integration ensures that applications can handle increased traffic without compromising on speed or reliability. The modular nature of golang modules allows developers to specify precise versions of the TiDB database, ensuring compatibility and stability across different environments.
Real-time Data Processing
In today’s fast-paced digital landscape, real-time data processing is crucial for gaining actionable insights and maintaining a competitive edge. The TiDB database excels in this area by supporting Hybrid Transactional and Analytical Processing (HTAP) workloads. When combined with golang modules, developers can efficiently manage dependencies and ensure that their applications are equipped to process data in real-time. This capability is particularly beneficial for businesses that rely on timely data analysis to drive decision-making and optimize operations.
Implementing TiDB in Golang Projects
Setting up TiDB with Go
Integrating the TiDB database into a Go project using golang modules is a straightforward process. Begin by initializing a golang module in your project directory:
go mod init <module-name>
Next, use the go get
command to add the TiDB database package to your project:
go get github.com/pingcap/tidb
This command fetches the latest version of the TiDB database package and updates your go.mod
file accordingly. By utilizing golang modules, you can ensure that your project remains consistent and up-to-date with the latest features and improvements from the TiDB database.
Case Studies and Examples
Numerous organizations have successfully integrated the TiDB database with golang modules to enhance their applications. For instance, a leading e-commerce platform leveraged this combination to handle peak traffic during major sales events, achieving seamless scalability and improved user experience. Another example is a financial services company that utilized the real-time data processing capabilities of the TiDB database, powered by golang modules, to deliver instant transaction insights to their clients.
These case studies highlight the transformative impact of integrating the TiDB database with golang modules, showcasing how developers can harness this powerful synergy to build scalable, high-performance applications.
By embracing the integration of golang modules with the TiDB database, developers can unlock new levels of efficiency and innovation in their projects. This powerful combination not only simplifies dependency management but also empowers developers to build applications that are ready to meet the demands of modern business environments.
Mastering Go Modules is pivotal for developers aiming to streamline dependency management and enhance application reliability. By embracing these modules, you can leverage innovations like advanced security features and reliable installations, as highlighted by Bartlomiej:
“If you don’t use it, I recommend switching to it as there are many innovations that work on top of Go modules.”
We encourage you to apply these techniques in your projects, moving away from outdated practices like vendoring. Explore further resources and community support to deepen your understanding and optimize your development workflow.