In today’s data-driven world, mastering advanced SQL techniques is crucial for data professionals. SQL is not just a language; it’s the backbone of data analysis and reporting, used by 69% of professional developers and 75% of Fortune 500 companies. As businesses increasingly rely on SQL for efficient data manipulation and extraction, understanding advanced transaction techniques becomes essential. This blog focuses on enhancing your skills in SQL transaction management, setting the stage for a deep dive into complex concepts that will elevate your expertise in handling large-scale data with precision and efficiency.
Understanding SQL Transactions
In the realm of database management, SQL transactions are pivotal for ensuring data integrity and consistency. They allow multiple operations to be executed as a single unit of work, providing a safety net against errors and system failures. Let’s delve into the core aspects of SQL transactions and why they are indispensable in modern data environments.
Definition and Purpose
What are SQL Transactions?
A SQL transaction is a sequence of operations performed on a database that is treated as a single logical unit. These operations are encapsulated within a BEGIN TRANSACTION
and COMMIT
or ROLLBACK
statement. The primary purpose of a transaction is to ensure that all operations within it are completed successfully. If any operation fails, the entire transaction can be rolled back, leaving the database in its original state. This mechanism is crucial for maintaining the accuracy and reliability of data, especially in complex systems where multiple users may access and modify data concurrently.
Importance of Transactions in Database Management
Transactions are essential in database management for several reasons:
- Data Integrity: By treating a series of operations as a single unit, transactions ensure that the database remains consistent even in the event of a failure.
- Concurrency Control: Transactions help manage simultaneous data modifications by multiple users, preventing conflicts and ensuring data accuracy.
- Error Recovery: In case of an error, transactions allow for a rollback, undoing any changes made during the transaction and preserving the integrity of the database.
These features make SQL transactions a cornerstone in industries like finance, e-commerce, and inventory management, where data consistency and reliability are paramount.
ACID Properties
The robustness of SQL transactions is underpinned by the ACID properties, which guarantee their reliability and effectiveness.
Atomicity
Atomicity ensures that all operations within a transaction are completed successfully or not at all. This means that if one part of the transaction fails, the entire transaction is aborted, and the database remains unchanged. This property is vital for maintaining data integrity, as it prevents partial updates that could lead to data corruption.
Consistency
Consistency ensures that a transaction takes the database from one valid state to another. It guarantees that any data written to the database must adhere to all predefined rules, such as constraints, cascades, and triggers. This property is crucial for maintaining the correctness of data across transactions.
Isolation
Isolation ensures that concurrent transactions do not interfere with each other. It defines how and when the changes made by one transaction become visible to other transactions. This property is critical for maintaining data accuracy in environments with high levels of concurrency, as it prevents issues such as dirty reads and lost updates.
Durability
Durability guarantees that once a transaction has been committed, it will remain so, even in the event of a system failure. This means that the results of a committed transaction are permanently recorded in the database, ensuring data persistence and reliability.
By adhering to these ACID properties, SQL transactions provide a robust framework for managing data operations, ensuring that databases remain accurate, consistent, and reliable.
Advanced SQL Transaction Techniques with TiDB
As data professionals, understanding advanced SQL transaction techniques is paramount for managing complex data environments efficiently. TiDB database, with its robust distributed architecture, offers a suite of advanced transaction features that ensure high availability, strong consistency, and seamless scalability. Let’s explore some of these techniques and how they can be implemented to enhance your data management capabilities.
Nested Transactions
Definition and Use Cases
Nested transactions are transactions within transactions, allowing for more granular control over complex operations. They are particularly useful in scenarios where a series of related operations need to be executed as part of a larger transaction. For instance, in a financial application, updating multiple accounts simultaneously while ensuring that each update is successful before committing the entire transaction can benefit from nested transactions.
Implementing Nested Transactions in SQL
Implementing nested transactions in SQL involves encapsulating smaller transactions within a larger one. While traditional SQL databases may not natively support nested transactions, TiDB database provides mechanisms to simulate this behavior using savepoints. By setting savepoints, you can roll back to specific points within a transaction without affecting the entire operation. This flexibility is crucial for maintaining data integrity and ensuring that partial failures do not compromise the entire dataset.
BEGIN TRANSACTION;
-- Perform initial operations
SAVEPOINT sp1;
-- Perform nested operations
ROLLBACK TO SAVEPOINT sp1; -- Rollback if necessary
COMMIT;
Savepoints
What are Savepoints?
Savepoints are markers within a transaction that allow for partial rollbacks. They provide a way to undo specific parts of a transaction without discarding all changes made. This feature is invaluable in complex SQL transaction scenarios where certain operations might fail, but others should still be committed.
Practical Applications of Savepoints
The practical applications of savepoints are vast. In e-commerce systems, for example, a transaction might involve updating inventory, processing payments, and sending notifications. If the notification fails, a savepoint allows you to roll back just that part of the transaction, retrying it without affecting the inventory and payment updates.
BEGIN TRANSACTION;
-- Update inventory
SAVEPOINT sp1;
-- Process payment
SAVEPOINT sp2;
-- Send notification
ROLLBACK TO SAVEPOINT sp2; -- Retry notification if it fails
COMMIT;
Distributed Transactions
Overview of Distributed Transactions
Distributed transactions span multiple networked databases, ensuring data consistency across different nodes. TiDB database supports distributed transactions natively, leveraging its optimistic and pessimistic transaction models to handle complex workloads efficiently. This capability is essential for applications that require data to be synchronized across various geographical locations.
Challenges and Solutions
Managing distributed transactions comes with challenges such as network latency, data consistency, and fault tolerance. TiDB database addresses these issues through its Multi-Raft protocol, which ensures that transactions are committed only when data is written to the majority of replicas. This approach guarantees strong consistency and high availability, even in the face of network partitions or node failures.
Practical Examples and SQL Code Snippets
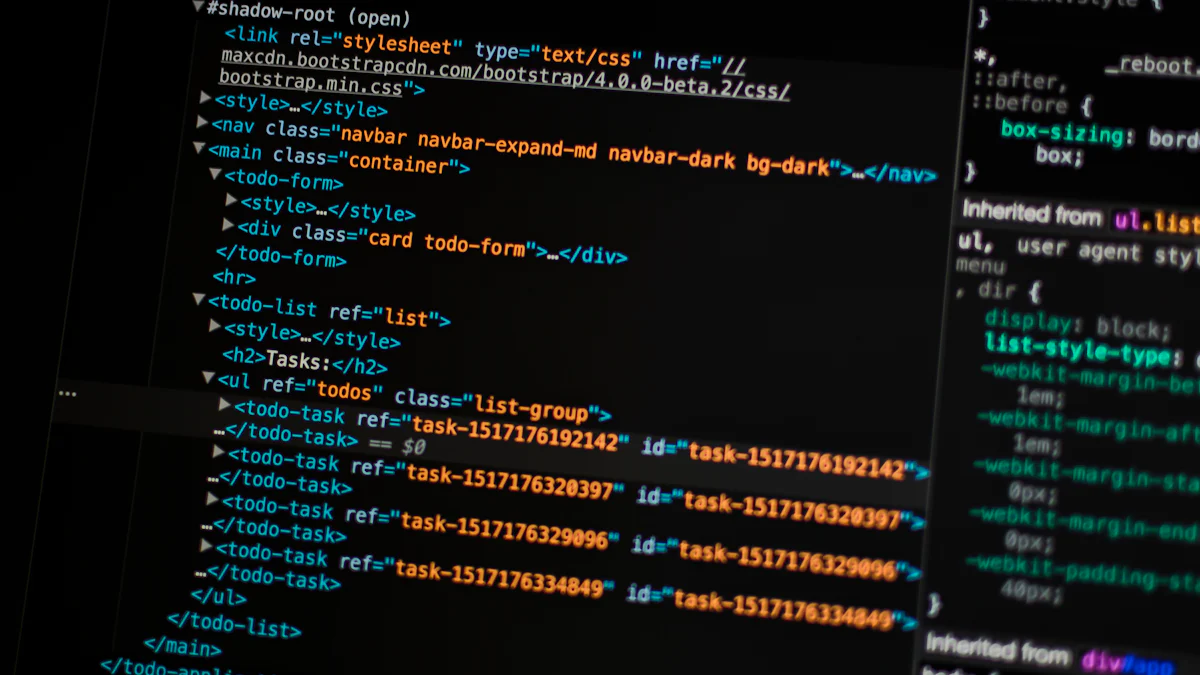
In this section, we delve into practical implementations of advanced SQL transaction techniques using the TiDB database. These examples are designed to provide you with hands-on experience and insights into effectively managing complex transactional scenarios.
Example of Nested Transactions
Step-by-step Implementation
Nested transactions offer a nuanced approach to handling complex operations by allowing transactions within transactions. This method is particularly useful when dealing with multiple related operations that need to be executed as part of a larger transaction. Here’s a step-by-step guide to implementing nested transactions in the TiDB database:
Begin the Main Transaction: Start by initiating the main transaction using the
BEGIN TRANSACTION
command.BEGIN TRANSACTION;
Set Savepoints: Use savepoints to mark specific points within the transaction. This allows for partial rollbacks if needed.
SAVEPOINT sp1;
Execute Nested Operations: Perform the necessary operations within the transaction. If an operation fails, roll back to the designated savepoint.
-- Execute some operations
ROLLBACK TO SAVEPOINT sp1; -- Rollback if an error occursCommit the Transaction: Once all operations are successfully executed, commit the transaction to finalize the changes.
COMMIT;
Common Pitfalls and How to Avoid Them
While implementing nested transactions, it’s crucial to be aware of potential pitfalls:
- Overuse of Savepoints: Excessive use of savepoints can lead to performance degradation. Use them judiciously to maintain efficiency.
- Complex Error Handling: Ensure robust error handling mechanisms are in place to manage failures effectively without compromising data integrity.
Using Savepoints in Real-world Scenarios
Code Snippets and Explanations
Savepoints provide a mechanism to manage complex transactions by allowing partial rollbacks. Here’s how they can be applied in real-world scenarios:
Inventory Management: In an e-commerce platform, updating inventory, processing payments, and sending notifications can be managed using savepoints.
BEGIN TRANSACTION;
-- Update inventory
SAVEPOINT sp1;
-- Process payment
SAVEPOINT sp2;
-- Send notification
ROLLBACK TO SAVEPOINT sp2; -- Retry notification if it fails
COMMIT;
This approach ensures that if any part of the process fails, only that segment is retried without affecting the entire transaction.
Best Practices
To maximize the effectiveness of savepoints:
- Strategic Placement: Place savepoints at critical junctures where failures are likely to occur.
- Minimal Usage: Use savepoints sparingly to avoid unnecessary complexity and maintain transaction performance.
Handling Distributed Transactions
Example Code and Explanation
Distributed transactions are essential for maintaining consistency across multiple databases. The TiDB database supports distributed transactions natively, ensuring high availability and strong consistency. Here’s an example of handling distributed transactions:
Initiate the Transaction: Begin the distributed transaction across multiple nodes.
BEGIN TRANSACTION;
Perform Operations: Execute the necessary operations on each node, ensuring data consistency.
-- Operation on Node 1
-- Operation on Node 2Commit the Transaction: Use the Multi-Raft protocol to ensure that the transaction is committed only when data is written to the majority of replicas.
COMMIT;
Tips for Efficient Management
Efficient management of distributed transactions involves:
- Network Optimization: Minimize latency by optimizing network configurations and ensuring reliable connections between nodes.
- Consistency Checks: Regularly perform consistency checks to ensure data integrity across all nodes.
By mastering these advanced SQL transaction techniques, you can enhance your ability to manage complex data environments effectively, leveraging the robust capabilities of the TiDB database.
In mastering advanced SQL transaction techniques, data professionals unlock the potential to manage complex data environments with precision and efficiency. These techniques, such as nested transactions, savepoints, and distributed transactions, are not just theoretical concepts but practical tools that enhance data integrity and consistency. As highlighted by industry experts, developing skills in these areas enables professionals to analyze large datasets effectively, leading to quicker insights and informed decision-making. Embrace these techniques in your daily work with the TiDB database to transform your data management capabilities and drive impactful results.