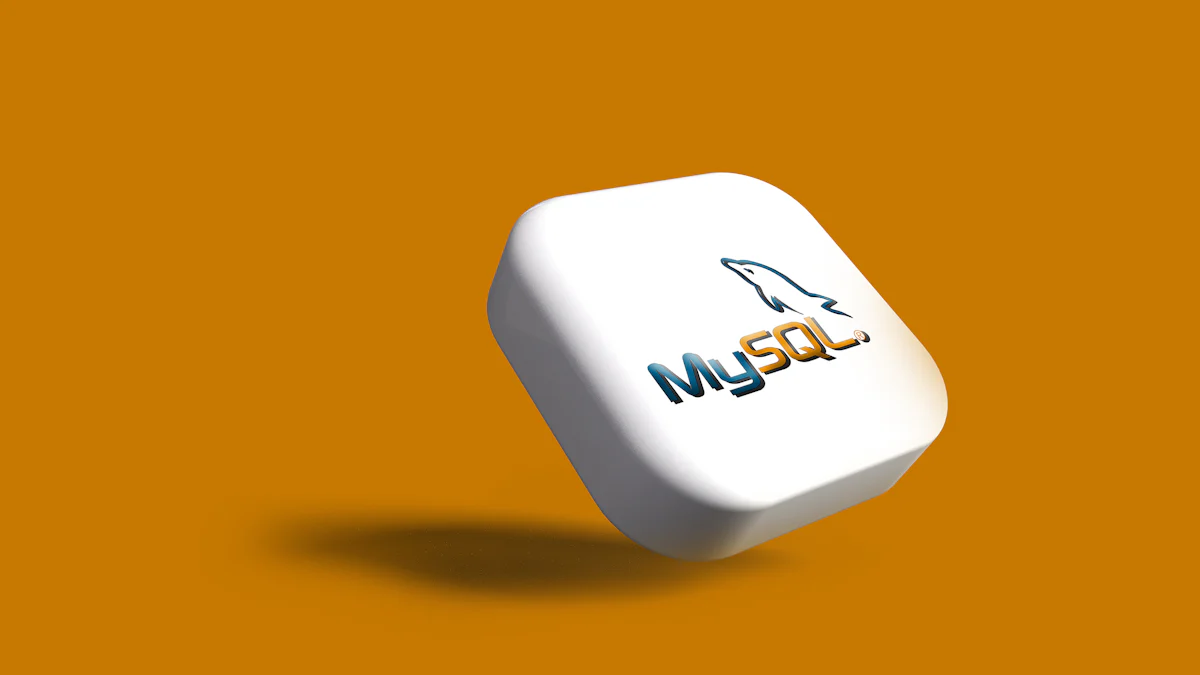
Understanding and avoiding common MySQL errors is crucial for maintaining optimal database performance and reliability. These errors can lead to significant issues, such as Out of Memory errors, which can disrupt operations and degrade user experience. By following best practices for error prevention, you can ensure a more stable and efficient database environment. Let’s dive into how you can safeguard your MySQL databases from these pitfalls.
Common MySQL Errors and Their Solutions
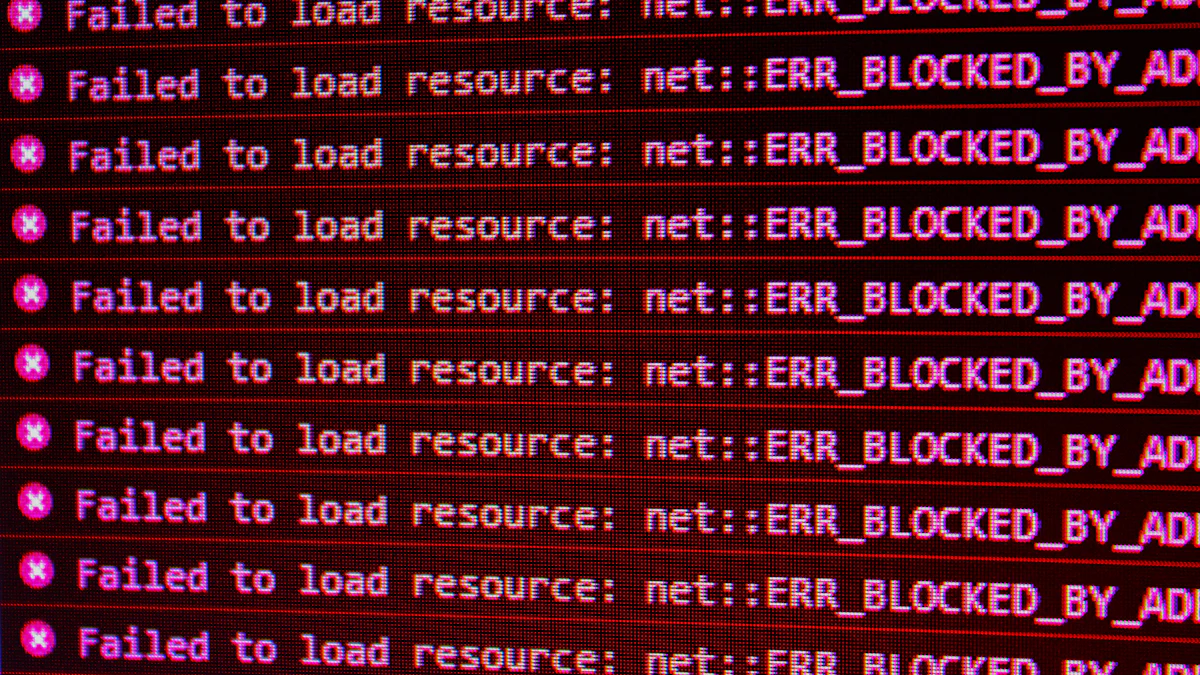
Common Connection Errors
Incorrect Username or Password
One of the most frequent issues users encounter is incorrect login credentials. This error typically manifests as an “Access denied” message. To resolve this, double-check the username and password you’re using to connect to the MySQL server. Ensure that there are no typos and that the credentials match those stored in the MySQL user table.
Host Not Allowed to Connect
Another common connection error occurs when the host you’re trying to connect from is not permitted by the MySQL server. This can be due to security settings that restrict access to specific IP addresses. To fix this, you need to update the MySQL user table to allow connections from the desired host. You can do this by running:
GRANT ALL PRIVILEGES ON *.* TO 'username'@'host' IDENTIFIED BY 'password';
FLUSH PRIVILEGES;
Too Many Connections
MySQL has a default limit on the number of simultaneous connections it can handle. When this limit is reached, new connection attempts will fail with a “Too many connections” error. To avoid this, you can increase the max_connections
setting in your MySQL configuration file (my.cnf
or my.ini
). Additionally, ensure that your application is efficiently managing connections, closing them when they are no longer needed.
Query Errors
Syntax Errors
Syntax errors are among the most common MySQL errors developers face. These occur when the SQL query is not written correctly. To prevent syntax errors, always double-check your queries for typos and ensure they follow the correct SQL syntax. Using tools like MySQL Workbench can help you validate your queries before executing them.
Data Type Mismatches
Data type mismatches happen when the data being inserted or queried does not match the column’s data type. For example, trying to insert a string into an integer column will result in an error. To avoid this, always ensure that the data types in your queries match those defined in your database schema.
Missing Tables or Columns
This error occurs when a query references a table or column that does not exist in the database. It often results from typos or changes in the database schema that have not been reflected in the application code. Regularly synchronize your database schema with your application code and use descriptive names to minimize these errors.
Performance Issues
Slow Queries
Slow queries can significantly impact the performance of your MySQL database. To identify and optimize slow queries, use the MySQL slow query log. This log records queries that take longer than a specified time to execute. Once identified, you can optimize these queries by adding indexes, rewriting the query, or breaking it into smaller, more manageable parts.
Indexing Problems
Improper indexing can lead to performance bottlenecks. While indexes can speed up data retrieval, too many indexes can slow down write operations. To strike a balance, only index columns that are frequently used in search conditions or join operations. Regularly review and optimize your indexes to maintain optimal performance.
Locking and Deadlocks
Locking and deadlocks occur when multiple transactions compete for the same resources, leading to a standstill. To minimize these issues, keep transactions short and avoid holding locks for extended periods. Use the SHOW ENGINE INNODB STATUS
command to diagnose and resolve deadlocks. Additionally, consider using the TiDB database, which offers better concurrency control and reduces the likelihood of deadlocks.
Data Integrity Errors
Maintaining data integrity is essential for ensuring the accuracy and consistency of your database. Let’s explore some common MySQL errors related to data integrity and how to address them effectively.
Foreign Key Constraints
Foreign key constraints are crucial for maintaining referential integrity between tables. However, they can also lead to errors if not managed correctly. A common issue arises when attempting to insert or update a record that violates a foreign key constraint. This typically results in an error message indicating that the foreign key constraint has failed.
To avoid this, ensure that the referenced key exists in the parent table before inserting or updating records in the child table. Additionally, consider using cascading options like ON DELETE CASCADE
or ON UPDATE CASCADE
to automatically update or delete related records, thereby maintaining data integrity without manual intervention.
ALTER TABLE child_table
ADD CONSTRAINT fk_example FOREIGN KEY (parent_id)
REFERENCES parent_table(id)
ON DELETE CASCADE
ON UPDATE CASCADE;
Duplicate Entries
Duplicate entries can compromise the uniqueness of data in your database, leading to inconsistencies and potential conflicts. This error often occurs when attempting to insert a record with a primary key or unique index that already exists.
To prevent duplicate entries, use the INSERT IGNORE
or ON DUPLICATE KEY UPDATE
clauses in your SQL statements. These clauses allow you to handle duplicates gracefully by either ignoring the insertion or updating the existing record.
INSERT INTO table_name (id, column1, column2)
VALUES (1, 'value1', 'value2')
ON DUPLICATE KEY UPDATE column1 = 'value1', column2 = 'value2';
Data Corruption
Data corruption is one of the most severe issues you can encounter in a MySQL database. It can result from hardware failures, software bugs, or improper shutdowns. Corrupted data can lead to inaccurate query results and even system crashes.
To mitigate the risk of data corruption, follow these best practices:
- Regular Backups: Perform regular backups of your database to ensure you can restore data in case of corruption.
- Use Reliable Storage: Invest in high-quality storage solutions to minimize the risk of hardware-induced corruption.
- Monitor System Health: Regularly monitor the health of your database and server to detect and address potential issues early.
In case of data corruption, tools like mysqlcheck
and innodb_force_recovery
can help diagnose and repair corrupted tables.
mysqlcheck -u root -p --auto-repair --check --optimize database_name
By understanding and addressing these common MySQL errors related to data integrity, you can maintain a robust and reliable database environment. Leveraging the advanced features of the TiDB database can further enhance your ability to manage data integrity effectively, offering better concurrency control and reducing the likelihood of errors.
Leveraging TiDB to Avoid Common MySQL Errors
When it comes to avoiding common MySQL errors, leveraging the advanced features of the TiDB database can be a game-changer. TiDB offers compatibility with MySQL while providing enhanced capabilities that address many of the typical pitfalls encountered in MySQL environments.
TiDB’s Compatibility with MySQL
Managing MySQL Compatibility Limitations
TiDB is designed to be highly compatible with MySQL, making it easier for users to migrate and integrate their existing applications. However, there are some differences to be aware of:
- ENUM Elements: Unlike MySQL, TiDB does not allow modification of ENUM elements but permits appending new ones. To avoid application inflexibility, it’s advisable to steer clear of ENUM types.
- Changing Column Types: Directly changing column types is not supported in TiDB. Instead, you should recreate the column with the desired type.
- Case Sensitivity and Collations: MySQL’s default collations are case-insensitive, whereas TiDB’s are case-sensitive. If needed, enable general collations during cluster initialization to align with MySQL behavior.
By understanding these limitations and planning accordingly, you can ensure a smoother transition and avoid common MySQL errors related to compatibility.
TiDB’s Advanced Features
Horizontal Scalability
One of the standout features of the TiDB database is its horizontal scalability. Unlike traditional MySQL databases that may struggle with scaling, TiDB allows you to add more nodes to your cluster seamlessly. This capability ensures that your database can handle increased loads without compromising performance. For instance, if you’re dealing with rapidly growing data, such as log storage, TiDB’s horizontal scalability is ideal for maintaining efficiency and avoiding performance bottlenecks.
Strong Consistency and High Availability
TiDB provides strong consistency and high availability, which are crucial for maintaining data integrity and minimizing downtime. The database uses a Raft-based consensus algorithm to ensure that all nodes in the cluster have consistent data. This reduces the risk of data corruption and other integrity issues commonly seen in MySQL, such as error 1032, which signals missing records during data manipulation. Additionally, TiDB’s high availability features, like automatic failover, ensure that your database remains operational even in the event of node failures.
Best Practices for Migrating from MySQL to TiDB
Refactoring Data Structures and Code
Migrating from MySQL to TiDB involves more than just data transfer; it requires thoughtful refactoring of data structures and code to optimize performance. Here are some best practices:
- Merge Sharded Tables: If you previously used sharding in MySQL, consider merging tables into a single table in TiDB. This reduces complexity and improves query performance.
- Introduce Redis for High-Frequency Queries: For queries that require frequent access, use Redis to offload read-only traffic from the database. This can significantly enhance performance and reduce the load on your TiDB cluster.
Choosing Between Sharding and No Sharding
Deciding whether to shard your database in TiDB depends on your specific use case:
- Unpredictable Database Capacity: For databases with rapidly growing data, TiDB’s horizontal scalability makes sharding unnecessary. The database can efficiently handle large volumes of data without the need for manual sharding.
- Multi-Dimensional Complex Queries: If your application requires complex queries across multiple dimensions, using TiDB without sharding can simplify your architecture and improve query performance.
- Uneven Data Distribution: In scenarios like social applications where data distribution can be uneven, TiDB’s even distribution of hot data among TiKV Regions prevents performance bottlenecks.
By following these best practices and leveraging TiDB’s advanced features, you can avoid common MySQL errors and create a robust, scalable, and high-performing database environment.
Avoiding common MySQL errors is essential for maintaining a robust and efficient database environment. By implementing the best practices discussed, you can significantly reduce the risk of encountering these issues. We encourage you to explore additional resources to deepen your understanding and further enhance your database management skills. Share your experiences and solutions with the community to help others navigate similar challenges. Together, we can achieve more reliable and performant database systems.
See Also
Identifying Sluggish Queries: Effective Methods to Enhance MySQL Speed
Effortless MySQL Database Backup Techniques
Transitioning Away from MySQL: 5 Vital Factors for Scalability and Efficiency
Mastery of MySQL Integer Data Types for Peak Performance
Transforming MySQL through Vector Similarity Search Innovation