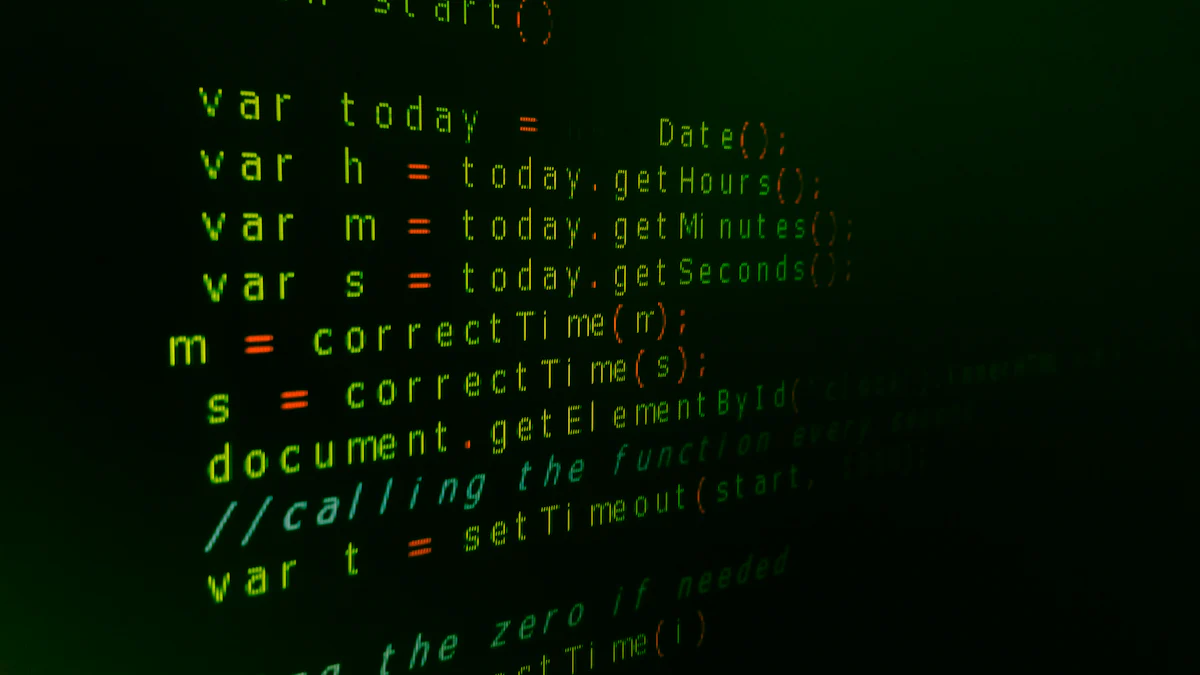
In the realm of software development, efficient memory management is crucial, and Golang garbage collection plays a pivotal role in this process. As developers strive to build high-performance applications, they often encounter challenges with inefficient garbage collection, which can lead to increased latency and resource consumption. Optimizing garbage collection not only enhances application performance but also ensures smoother operation and scalability. By understanding and addressing these challenges, developers can harness the full potential of the Golang ecosystem, paving the way for robust and efficient software solutions.
Understanding Golang’s Garbage Collector
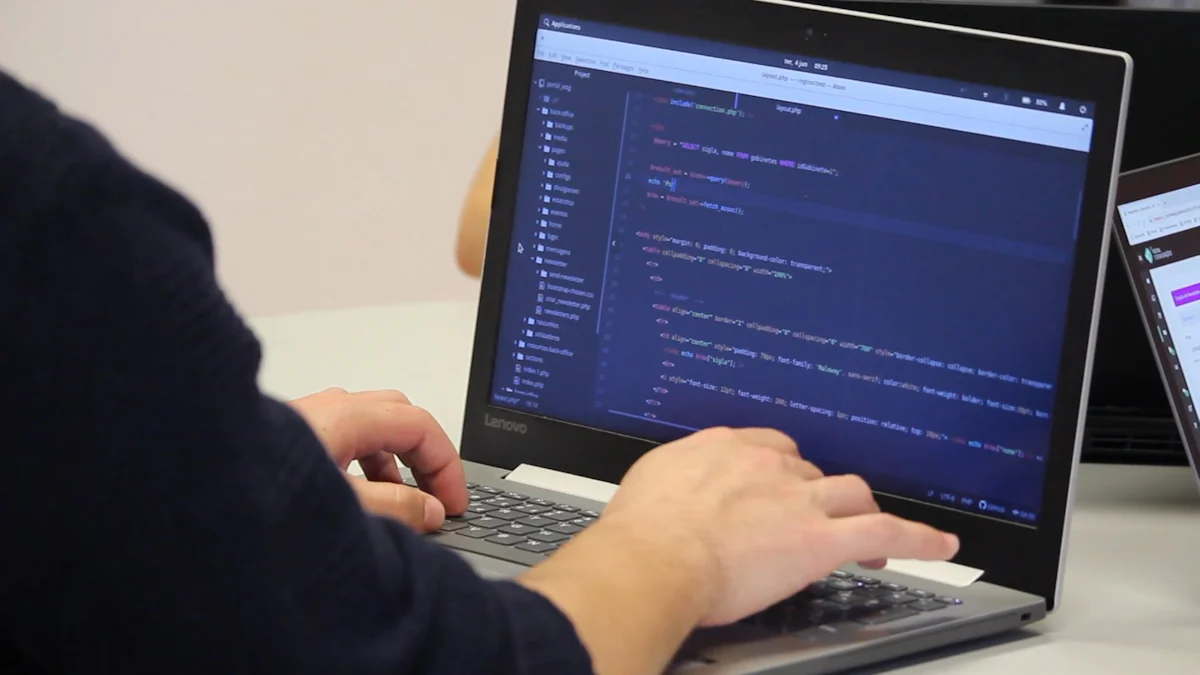
In the world of Go programming, understanding the intricacies of golang garbage collection is essential for creating efficient and high-performance applications. The garbage collector in Go is designed to manage memory automatically, freeing up developers to focus on building robust software without worrying about manual memory management.
Basics of Garbage Collection
What is Garbage Collection?
Garbage collection is a form of automatic memory management. The garbage collector attempts to reclaim memory occupied by objects that are no longer in use by the program. This process is crucial in preventing memory leaks, which can degrade application performance over time. In essence, garbage collection helps ensure that your application uses memory efficiently, reducing the risk of running out of memory or experiencing unexpected crashes.
How Golang Implements Garbage Collection
Golang employs a concurrent and parallel garbage collection strategy, which minimizes disruptions to program execution. This means that while your application is running, the garbage collector works in the background to identify and clean up unused memory. This approach allows for smoother performance, as the garbage collector does not need to pause the entire program to perform its tasks. Developers can also fine-tune garbage collection parameters and utilize memory profiling tools to optimize performance further.
Types of Garbage Collectors in Golang
Mark-and-Sweep Algorithm
The mark-and-sweep algorithm is one of the foundational techniques used in golang garbage collection. In this method, the garbage collector traverses the object graph, marking all reachable objects. Once marking is complete, it sweeps through the memory to reclaim space occupied by unmarked objects. This process is efficient but can introduce pauses in program execution if not managed correctly. However, Go’s implementation is designed to minimize these pauses, ensuring that applications remain responsive.
Concurrent Garbage Collection
Golang’s concurrent garbage collection is an advancement over traditional methods, aiming to reduce pause times significantly. By performing garbage collection concurrently with the program’s execution, Go ensures that the application remains responsive and efficient. This technique involves dividing the garbage collection process into smaller tasks that can be executed alongside the main program, reducing the impact on performance. The concurrent approach is particularly beneficial for applications with real-time requirements, where minimizing latency is critical.
Strategies for Efficient Golang Garbage Collection
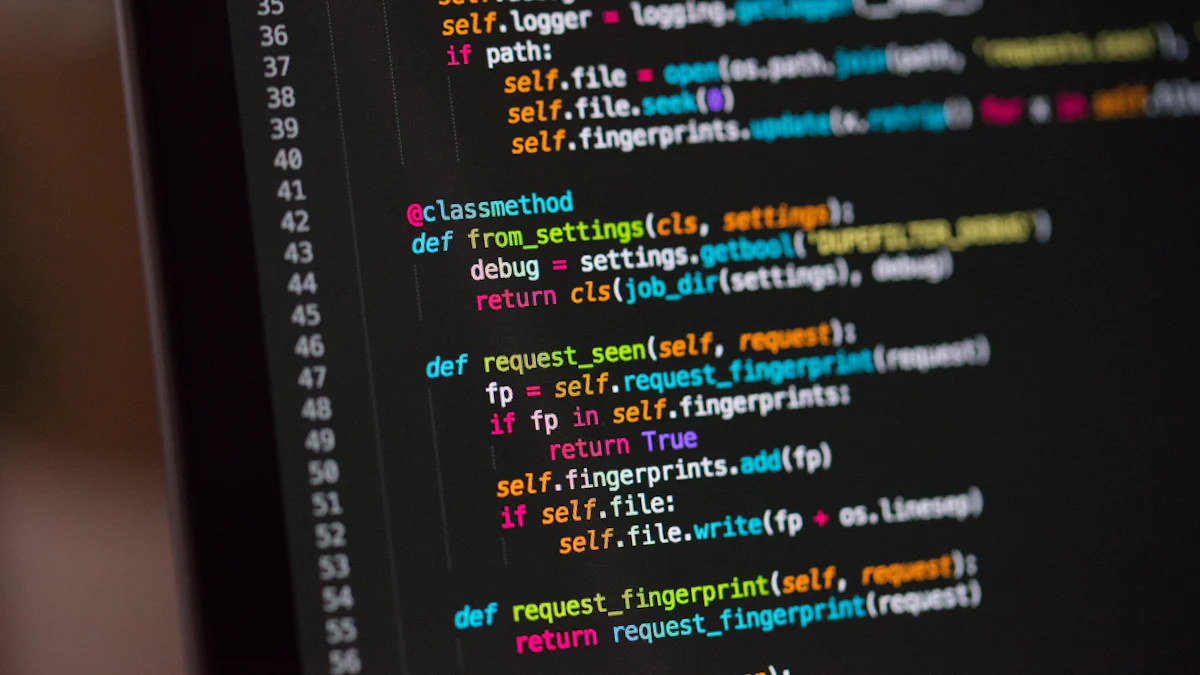
In the quest to optimize golang garbage collection, employing strategic memory management practices is essential. By understanding and implementing these strategies, developers can significantly enhance application performance and reliability.
Memory Management Best Practices
Avoiding Memory Leaks
Memory leaks occur when allocated memory is not released after it is no longer needed, leading to increased memory usage over time. In Golang, avoiding memory leaks is crucial for efficient garbage collection. Here are some best practices:
- Use defer statements: Properly utilize
defer
to ensure resources like files and network connections are closed promptly. - Limit global variables: Minimize the use of global variables that can inadvertently hold references to objects, preventing them from being garbage collected.
- Regular audits: Conduct regular code audits to identify and rectify potential memory leaks.
By adhering to these practices, developers can reduce the burden on the golang garbage collection process, allowing it to operate more efficiently.
Efficient Use of Pointers
Pointers in Go provide powerful capabilities but must be used judiciously to avoid unnecessary memory retention:
- Avoid unnecessary pointers: Only use pointers when necessary, as they can extend the lifetime of objects, keeping them alive longer than needed.
- Understand pointer semantics: Be mindful of how pointers interact with data structures, ensuring they do not inadvertently prevent garbage collection.
Efficient pointer usage not only aids in reducing memory footprint but also enhances the overall performance of golang garbage collection.
Tuning the Golang Garbage Collector
Fine-tuning the garbage collector can lead to significant performance improvements in Go applications. Here are some techniques to consider:
Adjusting GOGC Environment Variable
The GOGC
environment variable controls the garbage collection target percentage, influencing how often garbage collection occurs:
- Default setting: The default value is 100, meaning garbage collection is triggered when the heap size doubles.
- Tuning for performance: Adjust
GOGC
based on application needs. Lower values increase collection frequency, reducing memory usage but potentially impacting CPU performance, while higher values decrease frequency, increasing memory usage.
Experimenting with GOGC
settings allows developers to find a balance that suits their application’s performance requirements.
Profiling and Monitoring
Profiling and monitoring are indispensable for understanding and optimizing golang garbage collection:
- Use Go’s profiling tools: Tools like
pprof
provide insights into memory usage patterns and garbage collection behavior. - Monitor metrics: Regularly monitor garbage collection metrics to identify bottlenecks and areas for improvement.
By leveraging these tools, developers can gain a deeper understanding of how their applications interact with the garbage collector, enabling targeted optimizations.
Incorporating these strategies into your development workflow can lead to more efficient golang garbage collection, ultimately resulting in faster, more reliable applications.
Advanced Techniques
As developers delve deeper into optimizing golang garbage collection, advanced techniques such as custom allocators and real-world case studies provide valuable insights and practical solutions. These methods can significantly enhance performance, especially in complex applications requiring fine-tuned memory management.
Custom Allocators
Custom memory allocators offer a powerful way to manage memory more precisely in Go applications. By taking control over memory allocation and deallocation, developers can optimize performance and reduce the workload on the garbage collector.
When to Use Custom Allocators
Custom allocators are particularly beneficial in scenarios where:
- High-performance requirements: Applications that demand low latency and high throughput can benefit from custom allocators, as they allow for more predictable memory management.
- Specialized memory patterns: If your application has unique memory usage patterns, a custom allocator can be tailored to handle these efficiently, reducing overhead.
- Reducing garbage collection pressure: By managing memory manually, you can minimize the frequency and impact of golang garbage collection cycles, leading to smoother application performance.
However, it’s important to weigh the benefits against the complexity introduced by custom allocators. They require a deep understanding of memory management and can increase the risk of memory leaks if not implemented correctly.
Implementing Custom Allocators
Implementing a custom allocator in Go involves several steps:
- Identify memory patterns: Analyze your application’s memory usage to determine if a custom allocator is necessary and what type of allocator would be most effective.
- Design the allocator: Create a design that suits your application’s needs, considering factors like allocation size, frequency, and lifespan.
- Integrate with existing code: Carefully incorporate the custom allocator into your application, ensuring it interacts seamlessly with existing code and golang garbage collection mechanisms.
- Test and optimize: Rigorously test the allocator to ensure it functions correctly and optimizes performance as expected. Continuous monitoring and adjustments may be needed to maintain efficiency.
By following these steps, developers can harness the power of custom allocators to enhance their application’s performance and reduce reliance on golang garbage collection.
Real-World Examples
Examining real-world examples provides practical insights into how advanced techniques can be applied effectively. Let’s explore how PingCAP and other industry leaders have optimized golang garbage collection.
Case Study: PingCAP’s Approach
PingCAP, renowned for its TiDB database, has implemented innovative strategies to optimize memory management and enhance performance. By leveraging custom allocators and fine-tuning golang garbage collection parameters, PingCAP has achieved remarkable results:
- Improved scalability: The TiDB database supports large-scale data operations with minimal latency, thanks to efficient memory management practices.
- Enhanced reliability: By reducing the frequency of garbage collection cycles, PingCAP ensures consistent performance even under heavy workloads.
These strategies have enabled PingCAP to deliver a robust and scalable solution that meets the demands of modern applications.
Lessons Learned from Industry Leaders
Other industry leaders have also shared valuable lessons in optimizing golang garbage collection:
- Continuous monitoring: Regularly monitor garbage collection metrics to identify potential bottlenecks and areas for improvement.
- Iterative optimization: Adopt an iterative approach to optimization, making incremental changes and measuring their impact on performance.
- Community collaboration: Engage with the Go community to share experiences and learn from others’ successes and challenges.
By learning from these examples, developers can apply proven strategies to optimize golang garbage collection in their own applications, leading to enhanced performance and reliability.
In summary, optimizing golang garbage collection is essential for building high-performance applications. By implementing strategies like memory management best practices and tuning the garbage collector, developers can significantly enhance application efficiency. We encourage you to experiment with these techniques and monitor your application’s performance closely. Your insights and experiences are invaluable—feel free to share them with the community as we collectively strive for excellence in Go development.