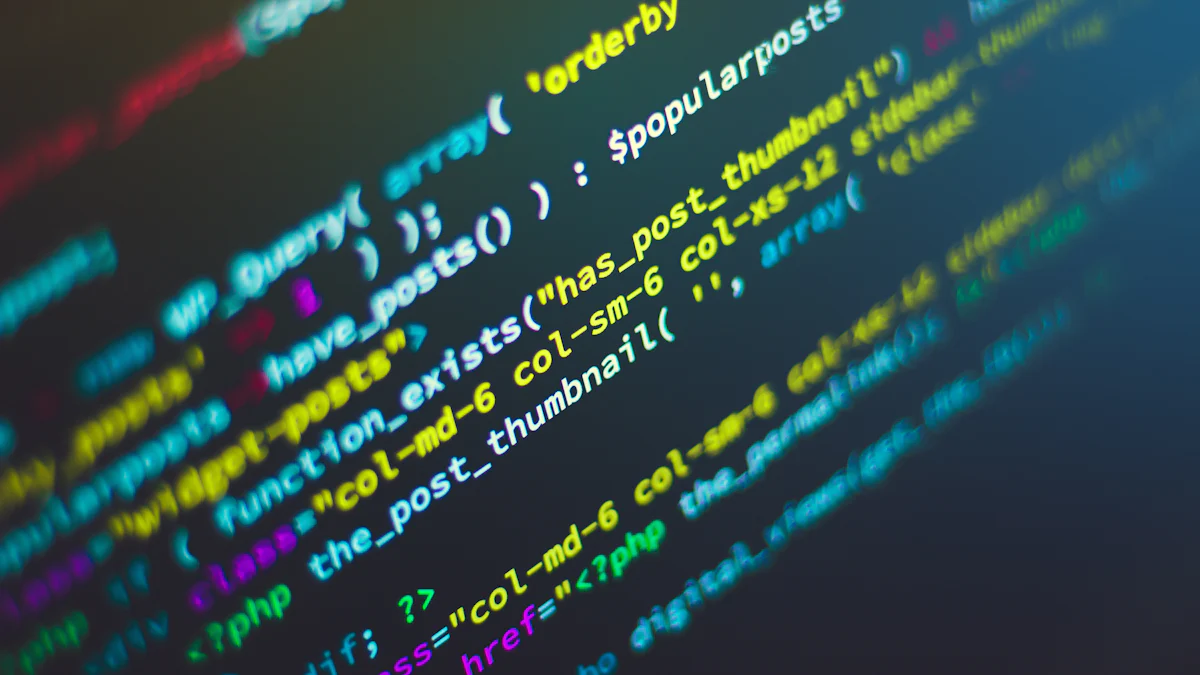
Efficient SQL operations are crucial for maintaining high-performance databases. The select update in sql commands are fundamental in SQL, enabling data retrieval and modification. However, without proper optimization, these operations can lead to slow query execution, high resource consumption, and potential data loss. This blog aims to provide practical strategies for optimizing select update in sql commands, ensuring faster data retrieval and efficient updates, ultimately enhancing the performance of your SQL queries.
Understanding the Basics of SELECT and UPDATE
To effectively optimize SQL queries, it’s essential to grasp the fundamentals of the SELECT
and UPDATE
commands. These commands are the backbone of data retrieval and modification in SQL databases, and understanding their syntax and common use cases is crucial for any database professional.
Overview of SELECT Command
The SELECT
command is used to retrieve data from one or more tables in a database. It allows you to specify which columns to fetch and apply various filters to narrow down the results.
Syntax and Usage
The basic syntax of the SELECT
command is straightforward:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
- SELECT: Specifies the columns to retrieve.
- FROM: Indicates the table from which to fetch the data.
- WHERE: Adds conditions to filter the results.
For example, to retrieve the names and ages of all users from a users
table where the age is greater than 30, you would use:
SELECT name, age
FROM users
WHERE age > 30;
Common Use Cases
The SELECT
command is versatile and can be used in various scenarios:
- Fetching specific columns: Retrieve only the necessary columns to reduce data transfer and improve performance.
- Filtering data: Use the
WHERE
clause to filter records based on specific conditions. - Joining tables: Combine data from multiple tables using
JOIN
clauses. - Aggregating data: Use aggregate functions like
SUM
,COUNT
,AVG
, etc., to summarize data.
Overview of UPDATE Command
The UPDATE
command is used to modify existing records in a table. It’s essential for maintaining and updating data without the need to delete and reinsert records.
Syntax and Usage
The basic syntax of the UPDATE
command is as follows:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
- UPDATE: Specifies the table to update.
- SET: Defines the columns and their new values.
- WHERE: Adds conditions to specify which records to update.
For instance, to update the age of a user with an ID of 1 in the users
table, you would use:
UPDATE users
SET age = 35
WHERE id = 1;
Common Use Cases
The UPDATE
command is commonly used in the following scenarios:
- Modifying specific records: Update only the records that meet certain conditions.
- Bulk updates: Change multiple records at once by specifying broader conditions.
- Data correction: Fix incorrect data entries without deleting and re-inserting records.
- Incremental updates: Adjust values incrementally, such as increasing prices by a percentage.
Understanding these fundamental aspects of the SELECT
and UPDATE
commands lays the groundwork for optimizing SQL queries. By mastering their syntax and use cases, you can ensure efficient data retrieval and modification, ultimately enhancing the performance of your SQL operations.
Strategies for Optimizing SELECT Queries
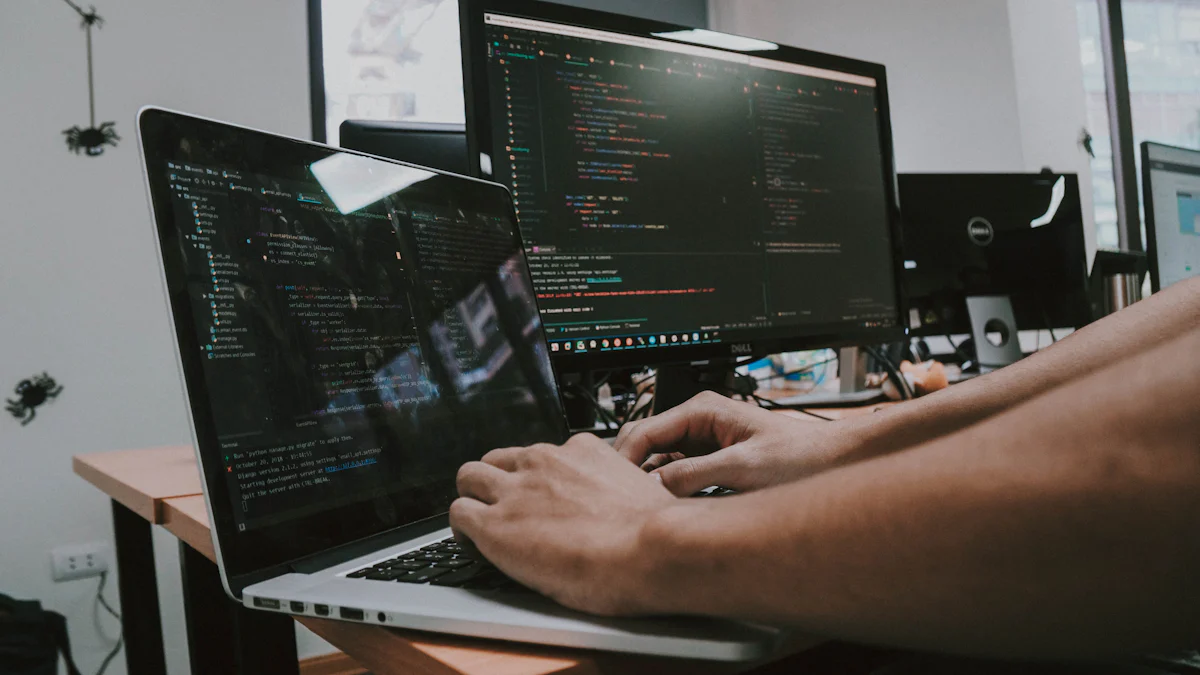
Optimizing SELECT
queries is crucial for enhancing the performance of your SQL database. By implementing effective strategies, you can significantly reduce query execution time and resource consumption. Here are some key techniques to optimize your SELECT
queries.
Indexing
Indexes are essential for speeding up data retrieval operations. They work by creating a data structure that allows the database to quickly locate the rows that match a given condition.
Types of Indexes
-
Single-Column Indexes: These indexes are created on a single column. They are useful when queries frequently filter or sort by that column.
CREATE INDEX idx_user_age ON users(age);
-
Composite Indexes: These are indexes on multiple columns. They are beneficial when queries filter or sort by multiple columns simultaneously.
CREATE INDEX idx_user_name_age ON users(name, age);
-
Unique Indexes: These ensure that all values in the indexed column are unique. They are often used for primary keys.
CREATE UNIQUE INDEX idx_user_email ON users(email);
When to Use Indexes
- Frequent Searches: Use indexes on columns that are frequently searched or filtered.
- Join Operations: Index columns that are commonly used in join conditions.
- Sorting: Index columns that are often used in
ORDER BY
clauses to speed up sorting operations. - High Cardinality: Index columns with a high number of unique values to improve query performance.
Query Optimization Techniques
Optimizing the way you write your queries can have a significant impact on performance. Here are some techniques to consider:
Using EXPLAIN Plan
The EXPLAIN
plan provides insights into how the database executes a query. It helps identify bottlenecks and areas for improvement.
EXPLAIN SELECT name, age FROM users WHERE age > 30;
By analyzing the output, you can understand whether indexes are being used effectively and if there are any full table scans that need to be addressed.
Avoiding SELECT *
Using SELECT *
retrieves all columns from a table, which can be inefficient. Instead, specify only the columns you need.
-- Inefficient
SELECT * FROM users WHERE age > 30;
-- Efficient
SELECT name, age FROM users WHERE age > 30;
Filtering and Sorting Data Efficiently
-
Use WHERE Clause: Apply filters early in the query to reduce the number of rows processed.
SELECT name, age FROM users WHERE age > 30 ORDER BY age;
-
Avoid HAVING Clause: Use the
WHERE
clause instead ofHAVING
whenever possible, asHAVING
is applied after aggregation, making it less efficient.-- Less efficient SELECT age, COUNT(*) FROM users GROUP BY age HAVING age > 30; -- More efficient SELECT age, COUNT(*) FROM users WHERE age > 30 GROUP BY age;
Handling Large Datasets
When dealing with large datasets, special techniques are required to maintain performance.
Pagination
Pagination helps manage large result sets by breaking them into smaller, more manageable chunks. This improves both performance and user experience.
SELECT name, age FROM users WHERE age > 30 ORDER BY age LIMIT 10 OFFSET 20;
Partitioning
Partitioning divides a table into smaller, more manageable pieces, improving query performance and maintenance.
-
Range Partitioning: Divides the table based on a range of values.
CREATE TABLE users ( id INT, name VARCHAR(50), age INT ) PARTITION BY RANGE (age) ( PARTITION p0 VALUES LESS THAN (20), PARTITION p1 VALUES LESS THAN (30), PARTITION p2 VALUES LESS THAN (40) );
-
Hash Partitioning: Distributes rows across partitions based on a hash function.
CREATE TABLE users ( id INT, name VARCHAR(50), age INT ) PARTITION BY HASH(id) PARTITIONS 4;
By implementing these strategies, you can optimize your SELECT
queries, ensuring faster data retrieval and more efficient use of resources. This not only enhances the performance of your SQL database but also improves the overall user experience.
Strategies for Optimizing UPDATE Statements
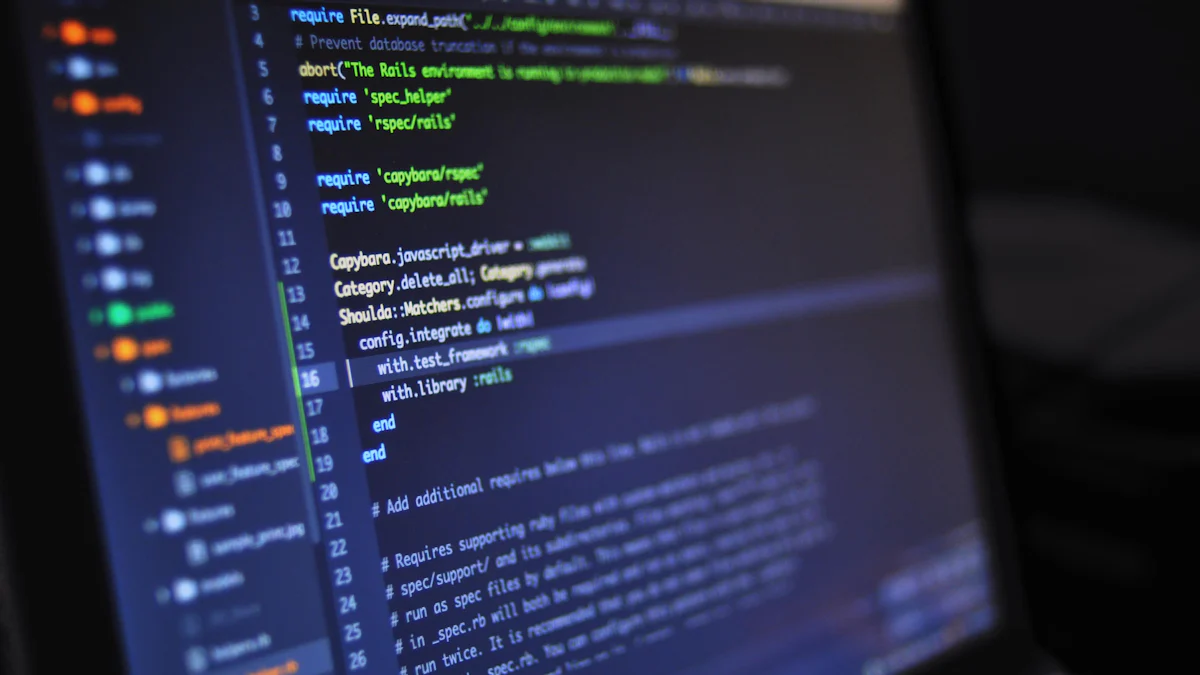
Optimizing UPDATE
statements is crucial for maintaining the performance and integrity of your SQL database. Here are some effective strategies to ensure your updates are efficient and minimize potential issues.
Minimizing Lock Contention
Lock contention occurs when multiple transactions compete for the same resources, leading to delays and potential deadlocks. Minimizing lock contention is essential for maintaining smooth database operations.
Using Transactions Wisely
Transactions are powerful tools for ensuring data consistency, but they can also lead to lock contention if not used wisely. Here are some best practices:
-
Keep Transactions Short: Long-running transactions hold locks for extended periods, increasing the likelihood of contention. Aim to keep transactions as short as possible.
BEGIN; UPDATE users SET age = 35 WHERE id = 1; COMMIT;
-
Use Appropriate Isolation Levels: Choose an isolation level that balances consistency and performance. For instance,
READ COMMITTED
can reduce lock contention compared toSERIALIZABLE
.SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
-
Avoid User Interaction Within Transactions: Ensure that transactions do not wait for user input, as this can prolong lock duration.
Batch Updates
Batch updates can significantly reduce lock contention by updating records in smaller chunks rather than all at once. This approach minimizes the time locks are held and reduces the risk of conflicts.
-
Batch Size: Determine an optimal batch size that balances performance and resource usage. Too large a batch can still cause contention, while too small may lead to inefficiency.
UPDATE users SET age = age + 1 WHERE id BETWEEN 1 AND 1000;
-
Iterative Updates: Use loops or scripts to perform updates iteratively, ensuring each batch completes before starting the next.
DECLARE @batchSize INT = 1000; DECLARE @start INT = 1; WHILE @start <= (SELECT MAX(id) FROM users) BEGIN UPDATE users SET age = age + 1 WHERE id BETWEEN @start AND @start + @batchSize - 1; SET @start = @start + @batchSize; END;
Efficient Use of WHERE Clauses
The WHERE
clause is critical in UPDATE
statements to ensure only the intended records are modified. Efficient use of WHERE
clauses can greatly enhance performance.
Indexing Columns in WHERE Clauses
Indexes play a vital role in speeding up the search process within the WHERE
clause. By indexing columns used in WHERE
clauses, you can significantly reduce the time required to locate the records to be updated.
-
Single-Column Indexes: Create indexes on individual columns frequently used in
WHERE
clauses.CREATE INDEX idx_user_id ON users(id);
-
Composite Indexes: Use composite indexes for queries involving multiple columns.
CREATE INDEX idx_user_age_status ON users(age, status);
Avoiding Full Table Scans
Full table scans can be detrimental to performance, especially in large tables. Here are some tips to avoid them:
-
Use Specific Conditions: Ensure your
WHERE
clause is specific enough to leverage indexes and avoid scanning the entire table.-- Inefficient UPDATE users SET status = 'active' WHERE age > 30; -- Efficient UPDATE users SET status = 'active' WHERE age > 30 AND status = 'inactive';
-
Analyze Execution Plans: Use the
EXPLAIN
plan to understand how your query is executed and identify any full table scans.EXPLAIN UPDATE users SET status = 'active' WHERE age > 30 AND status = 'inactive';
Handling Large Updates
Large updates can be challenging due to their impact on performance and potential for lock contention. Here are strategies to handle them effectively.
Breaking Down Large Updates
Breaking down large updates into smaller, more manageable chunks can help maintain performance and reduce the risk of lock contention.
-
Chunking: Divide the update operation into smaller chunks and process each chunk separately.
DECLARE @batchSize INT = 1000; DECLARE @start INT = 1; WHILE @start <= (SELECT MAX(id) FROM users) BEGIN UPDATE users SET age = age + 1 WHERE id BETWEEN @start AND @start + @batchSize - 1; SET @start = @start + @batchSize; END;
Using Temporary Tables
Temporary tables can be used to stage data before performing large updates, reducing the load on the main table and minimizing lock contention.
-
Staging Data: Insert data into a temporary table, perform necessary updates, and then merge back into the main table.
CREATE TEMPORARY TABLE temp_users AS SELECT * FROM users WHERE age > 30; UPDATE temp_users SET age = age + 1; UPDATE users u JOIN temp_users t ON u.id = t.id SET u.age = t.age;
By implementing these strategies, you can optimize your UPDATE
statements, ensuring efficient data modification while minimizing the risk of lock contention and performance degradation. These techniques will help maintain the overall health and responsiveness of your SQL database.
Advanced Techniques and Best Practices
As you become more adept at optimizing SQL queries, incorporating advanced techniques and adhering to best practices can further enhance the performance and reliability of your database operations. This section delves into sophisticated strategies for analyzing query performance and maintaining your database.
Analyzing Query Performance
Understanding how your queries perform is crucial for identifying bottlenecks and areas for improvement. Here are some effective methods for analyzing query performance.
Using Performance Monitoring Tools
Performance monitoring tools are indispensable for tracking the efficiency of your SQL queries. These tools provide real-time insights into query execution times, resource usage, and potential issues. Some popular performance monitoring tools include:
- New Relic: Offers comprehensive monitoring for databases, including query performance metrics and alerts.
- SolarWinds Database Performance Analyzer: Provides detailed analysis of query performance, helping you identify slow-running queries and their root causes.
- pgAdmin: A powerful open-source tool for PostgreSQL that includes query performance monitoring features.
Using these tools, you can gain valuable insights into how your queries are performing and take proactive measures to optimize them.
Interpreting Query Execution Plans
Query execution plans are a roadmap of how the database engine executes a query. By interpreting these plans, you can identify inefficiencies and optimize your queries accordingly.
To generate an execution plan, use the EXPLAIN
command:
EXPLAIN SELECT name, age FROM users WHERE age > 30;
The output will provide details on how the query is executed, including:
- Scan Type: Indicates whether the query uses an index scan, sequential scan, or other methods.
- Cost Estimates: Provides an estimate of the resources required to execute the query.
- Rows: Shows the number of rows processed at each step.
By analyzing these details, you can determine if your query is using indexes effectively or if there are any full table scans that need to be addressed.
Regular Maintenance
Regular maintenance is essential for ensuring the long-term performance and health of your SQL database. Here are some key maintenance tasks to consider.
Updating Statistics
Statistics play a crucial role in query optimization by providing the database engine with information about the distribution of data within tables. Keeping these statistics up-to-date ensures that the query optimizer can make informed decisions.
To update statistics, use the following command:
ANALYZE users;
This command updates the statistics for the users
table, helping the query optimizer generate more efficient execution plans.
Rebuilding Indexes
Over time, indexes can become fragmented, leading to degraded performance. Rebuilding indexes can help restore their efficiency and improve query performance.
To rebuild an index, use the following command:
ALTER INDEX idx_user_age REBUILD;
This command rebuilds the idx_user_age
index, ensuring it remains efficient and effective for query operations.
Additional Tips for SQL Performance Tuning
In addition to the techniques mentioned above, here are some additional tips for optimizing SQL queries:
- Avoid SELECT DISTINCT: Use specific filters instead of
SELECT DISTINCT
to reduce unnecessary processing. - Use INNER JOINs: Prefer
INNER JOIN
overWHERE
or cross joins for better performance. - Limit Wildcards: Use wildcards at the end of a phrase only to improve search efficiency.
- Run Queries During Off-Peak Hours: Schedule intensive queries during off-peak hours to minimize impact on performance.
By incorporating these advanced techniques and best practices, you can ensure that your SQL queries are optimized for performance and reliability. Regularly analyzing query performance and maintaining your database will help you stay ahead of potential issues and keep your database running smoothly.
SELECT and UPDATE in SQL with TiDB
Leveraging TiDB for SELECT and UPDATE
TiDB, an advanced open-source distributed SQL database, offers unique advantages for executing SELECT
and UPDATE
operations efficiently. Leveraging TiDB can significantly enhance your database performance and scalability.
Benefits of Using TiDB
- Horizontal Scalability: TiDB allows you to scale out by adding more nodes to the cluster, ensuring that your
SELECT
andUPDATE
operations remain performant even as your dataset grows. - High Availability: With built-in replication and automatic failover, TiDB ensures that your database remains available, minimizing downtime and maintaining data integrity during
SELECT
andUPDATE
operations. - Strong Consistency: TiDB provides ACID compliance, ensuring that your
UPDATE
operations are reliable and yourSELECT
queries return consistent results. - HTAP Capabilities: TiDB supports Hybrid Transactional and Analytical Processing (HTAP), enabling you to run real-time analytics on fresh transactional data without impacting the performance of your
SELECT
andUPDATE
operations.
Real-World Use Cases
- E-commerce Platforms: TiDB is used by e-commerce platforms to handle high volumes of
SELECT
andUPDATE
operations, such as retrieving product details and updating inventory levels in real-time. - Financial Services: Financial institutions leverage TiDB for its strong consistency and high availability, ensuring accurate
SELECT
queries for reporting and reliableUPDATE
operations for transaction processing. - Gaming Industry: Companies like CAPCOM utilize TiDB to manage player data, perform real-time analytics, and ensure seamless gameplay experiences through efficient
SELECT
andUPDATE
operations.
Best Practices for SELECT and UPDATE in TiDB
To maximize the benefits of TiDB, it’s essential to follow best practices tailored to its unique architecture.
Optimizing SELECT Queries in TiDB
-
Use Appropriate Indexes: Just like in traditional SQL databases, indexing is crucial. Ensure that columns frequently used in
SELECT
queries are indexed to speed up data retrieval. -
Leverage Partitioning: TiDB supports table partitioning, which can help manage large datasets more efficiently. Partition your tables based on query patterns to improve
SELECT
query performance. -
Utilize TiDB’s EXPLAIN Statement: Use the
EXPLAIN
statement to analyze yourSELECT
queries. This helps you understand how TiDB executes your queries and identify potential bottlenecks.EXPLAIN SELECT name, age FROM users WHERE age > 30;
-
Optimize Join Operations: When performing joins, ensure that the join conditions are indexed. This reduces the amount of data TiDB needs to scan, speeding up your
SELECT
queries.
Optimizing UPDATE Statements in TiDB
-
Batch Updates: Similar to other SQL databases, performing updates in batches can reduce lock contention and improve performance. Use smaller batches to minimize the impact on the system.
UPDATE users SET status = 'active' WHERE age > 30 LIMIT 1000;
-
Use Transactions Wisely: Keep transactions short to avoid long-held locks. This is especially important in a distributed environment like TiDB, where network latency can add to transaction duration.
BEGIN; UPDATE users SET age = 35 WHERE id = 1; COMMIT;
-
Monitor Performance: Regularly monitor the performance of your
UPDATE
statements using TiDB’s built-in tools and adjust your strategies accordingly. This helps in identifying and resolving performance issues proactively.
By following these best practices, you can optimize your SELECT
and UPDATE
operations in TiDB, ensuring efficient data retrieval and modification. This not only enhances the performance of your database but also improves the overall user experience.
In summary, optimizing SELECT
and UPDATE
operations in SQL is essential for maintaining high-performance databases. Continuous optimization ensures your queries run efficiently, reducing execution time and resource consumption. By applying these strategies, you can significantly enhance your SQL performance.
At PingCAP, we are committed to innovation and customer satisfaction, continually improving our offerings to meet your evolving needs. Leverage these techniques with TiDB database to achieve robust, scalable, and efficient database operations.
See Also
Enhancing SQL Query Performance Through Optimization Techniques
Leveraging SQL EXPLAIN for Improved Query Performance
Identifying Slow Queries: Effective Methods to Enhance MySQL Performance
Comparing Primary Key and Foreign Key for Optimal Data Management in Databases
Applying Similar Techniques to Manage Vectors and MySQL Data