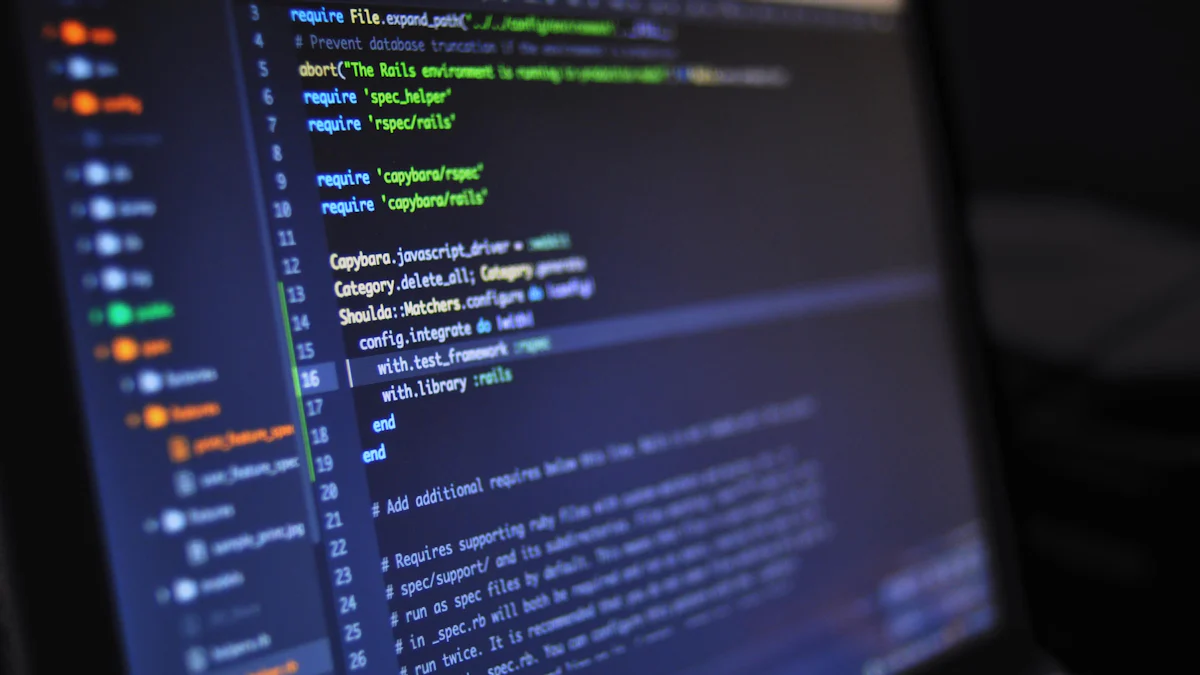
Temporary tables in SQL are a create temp table sql powerful tool for developers and database administrators. These tables provide an efficient way to store data needed for short-term tasks, saving the hassle of creating permanent tables for transient operations. Whether you’re staging data for transformation, performing intermediate calculations, or handling large batches of data in real-time, temporary tables can significantly streamline your workflow. By allowing you to store and reuse intermediate query results, they help avoid redundant computations and improve overall performance.
Understanding Temporary Tables
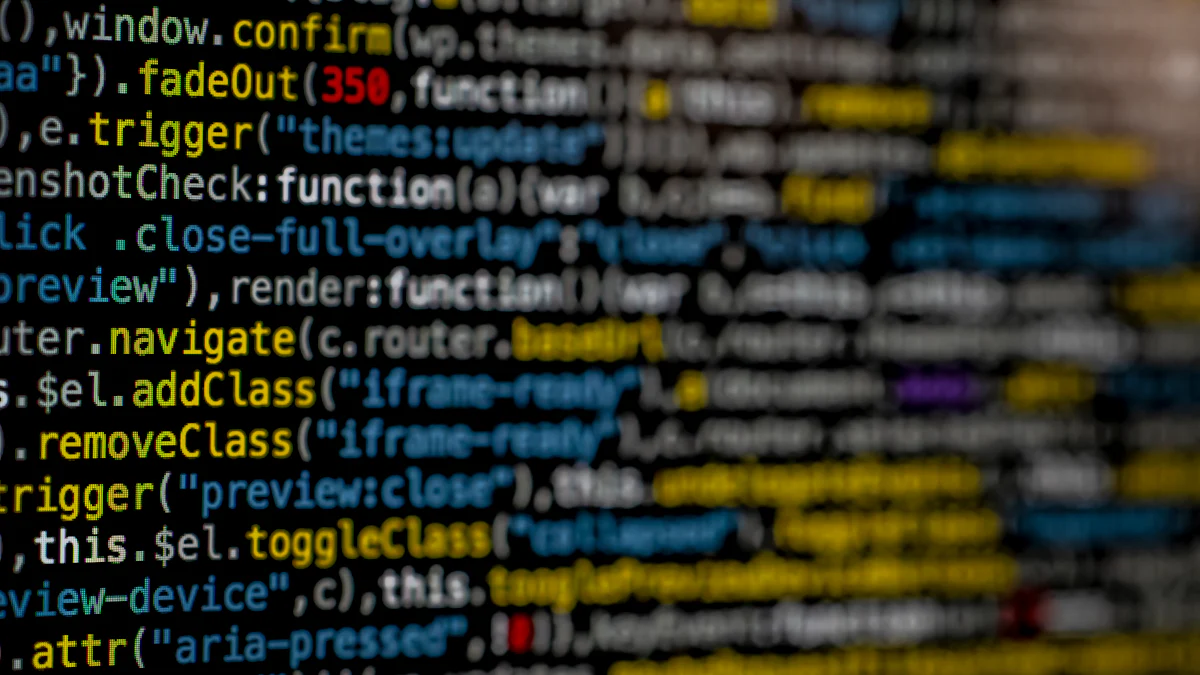
What are Temporary Tables?
Temporary tables in SQL are special types of tables that exist only for the duration of a session or a specific transaction. They are created on-the-fly and are typically used to perform complex calculations, store intermediate results, or manipulate subsets of data during the execution of a query or a series of queries.
Definition and Characteristics
Temporary tables are not permanently stored in the database. Instead, they provide a flexible and efficient way to process and manipulate data without affecting the original source. These tables are session-specific, meaning they are automatically dropped when the session ends, ensuring that they do not persist beyond their intended use.
Key characteristics of temporary tables include:
- Ephemeral Nature: They exist only for the duration of a session or transaction.
- Isolation: Data stored in temporary tables is isolated to the session that created them.
- Automatic Cleanup: They are automatically dropped when the session ends or the transaction completes.
Types of Temporary Tables (Local vs. Global)
There are two main types of temporary tables in SQL: local temporary tables and global temporary tables.
-
Local Temporary Tables: These are prefixed with a single
#
(e.g.,#TempTable
). They are visible only within the session that created them and are automatically dropped when the session ends. -
Global Temporary Tables: These are prefixed with
##
(e.g.,##GlobalTempTable
). They are visible to all sessions and are dropped when the last session referencing the table ends.
Benefits of Using Temporary Tables
Temporary tables offer several advantages that make them invaluable in various scenarios.
Performance Improvement
One of the primary benefits of using temporary tables is performance improvement. By storing intermediate results, temporary tables can reduce the complexity and execution time of complex queries. This is particularly useful in scenarios where subqueries might drag performance. For instance, creating summary tables for an OLAP database can be more efficient with temporary tables.
Simplifying Complex Queries
Temporary tables provide a convenient way to break down complex problems into smaller, more manageable steps. They allow for the separation of data processing stages, which can enhance code readability and simplify query logic. For example, you can store intermediate calculations in a temporary table and then use that table in subsequent queries, making the overall process easier to understand and maintain.
Data Isolation and Security
Temporary tables ensure that data is isolated to the session that created them. This isolation provides an added layer of security, as the data in temporary tables is not accessible by other sessions. This feature is particularly useful in environments where multiple users or applications are accessing the database simultaneously, as it prevents accidental data leakage or conflicts.
How to Create Temporary Tables in SQL
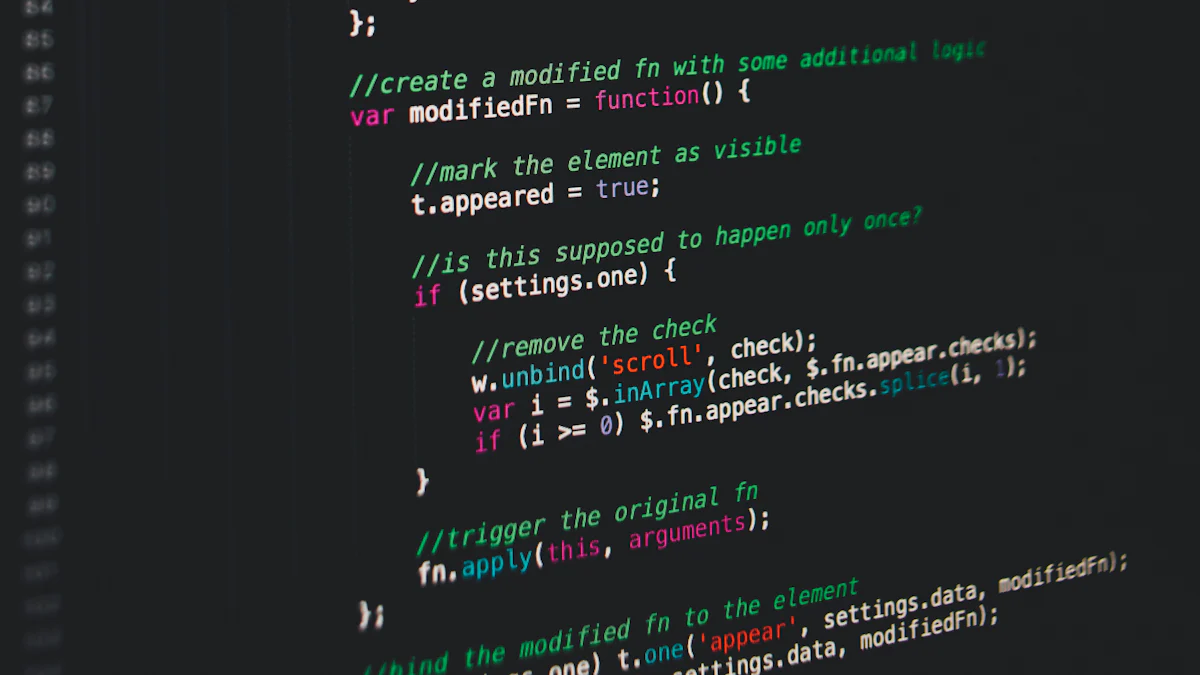
Creating temporary tables in SQL is a straightforward process that can significantly enhance your database operations. This section will guide you through the syntax and provide practical examples to help you understand how to effectively use temporary tables.
Syntax and Basic Examples
Understanding the syntax for creating temporary tables is essential. Let’s break it down into two parts: creating local temporary tables and global temporary tables.
Creating Local Temporary Tables
Local temporary tables are session-specific and are created using a single #
prefix. Here’s the basic syntax:
CREATE TEMPORARY TABLE #LocalTempTable (
column1 datatype,
column2 datatype,
...
);
For example, to create a local temporary table to store user data, you might use:
CREATE TEMPORARY TABLE #UserData (
UserID INT,
UserName VARCHAR(50),
UserEmail VARCHAR(100)
);
This table will be available only within the session that created it and will be automatically dropped when the session ends.
Creating Global Temporary Tables
Global temporary tables are accessible across all sessions and are created using a double ##
prefix. Here’s the syntax:
CREATE TEMPORARY TABLE ##GlobalTempTable (
column1 datatype,
column2 datatype,
...
);
For instance, to create a global temporary table for storing product data, you could use:
CREATE TEMPORARY TABLE ##ProductData (
ProductID INT,
ProductName VARCHAR(50),
ProductPrice DECIMAL(10, 2)
);
This table will remain available until the last session referencing it ends.
Practical Examples
To illustrate the power of temporary tables, let’s look at some practical examples.
Example 1: Storing Intermediate Results
Imagine you’re working on a complex query that involves multiple calculations. Instead of performing all calculations in a single query, you can break it down using temporary tables.
First, create a temporary table to store intermediate results:
CREATE TEMPORARY TABLE #SalesSummary AS
SELECT
SalesPersonID,
SUM(SalesAmount) AS TotalSales
FROM
Sales
GROUP BY
SalesPersonID;
Now, you can use this temporary table in subsequent queries:
SELECT
s.SalesPersonID,
s.TotalSales,
p.SalesPersonName
FROM
#SalesSummary s
JOIN
SalesPersons p ON s.SalesPersonID = p.SalesPersonID;
This approach simplifies your queries and improves readability.
Example 2: Simplifying Joins and Subqueries
Temporary tables can also simplify complex joins and subqueries. For example, if you need to join multiple large tables, you can use temporary tables to store intermediate join results.
First, create a temporary table to store the initial join results:
CREATE TEMPORARY TABLE #InitialJoin AS
SELECT
o.OrderID,
o.CustomerID,
c.CustomerName
FROM
Orders o
JOIN
Customers c ON o.CustomerID = c.CustomerID;
Then, use this temporary table to perform further operations:
SELECT
ij.OrderID,
ij.CustomerName,
p.ProductName
FROM
#InitialJoin ij
JOIN
OrderDetails od ON ij.OrderID = od.OrderID
JOIN
Products p ON od.ProductID = p.ProductID;
By breaking down the query into smaller steps, you make it easier to manage and optimize.
Managing Temporary Tables in TiDB
Managing temporary tables in TiDB database is crucial for efficient data handling and ensuring optimal performance. This section will guide you through inserting, updating, deleting, and dropping temporary tables, providing practical insights and examples.
Inserting Data into Temporary Tables
Inserting data into temporary tables can be done using two primary methods: INSERT INTO SELECT
and INSERT VALUES
.
Using INSERT INTO SELECT
The INSERT INTO SELECT
statement allows you to populate a temporary table with data from an existing table or query result. This method is particularly useful when you need to create temp table sql with data derived from complex queries.
-- Create a temporary table to store sales data
CREATE TEMPORARY TABLE #SalesData (
SalesPersonID INT,
TotalSales DECIMAL(10, 2)
);
-- Insert data into the temporary table from an existing table
INSERT INTO #SalesData (SalesPersonID, TotalSales)
SELECT SalesPersonID, SUM(SalesAmount)
FROM Sales
GROUP BY SalesPersonID;
This approach helps in aggregating data efficiently and storing intermediate results for further processing.
Using INSERT VALUES
The INSERT VALUES
statement is used to insert specific rows of data into a temporary table. This method is ideal for inserting a small number of records manually.
-- Create a temporary table to store product details
CREATE TEMPORARY TABLE #ProductDetails (
ProductID INT,
ProductName VARCHAR(50),
ProductPrice DECIMAL(10, 2)
);
-- Insert individual rows into the temporary table
INSERT INTO #ProductDetails (ProductID, ProductName, ProductPrice)
VALUES (1, 'Laptop', 999.99),
(2, 'Smartphone', 499.99),
(3, 'Tablet', 299.99);
Using INSERT VALUES
is straightforward and effective for quick data entry tasks.
Updating and Deleting Data
Temporary tables often require updates or deletions to maintain data accuracy and relevance.
Updating Data in Temporary Tables
Updating data in a temporary table follows the same syntax as updating a regular table. This is essential when you need to modify existing records based on new information.
-- Update the price of a product in the temporary table
UPDATE #ProductDetails
SET ProductPrice = 899.99
WHERE ProductID = 1;
This ensures that your temporary table reflects the most current data.
Deleting Data from Temporary Tables
Deleting data from a temporary table is also similar to deleting data from a permanent table. This operation is useful for removing outdated or irrelevant records.
-- Delete a product from the temporary table
DELETE FROM #ProductDetails
WHERE ProductID = 3;
Regularly cleaning up temporary tables helps in managing resources effectively.
Dropping Temporary Tables
Dropping temporary tables can be done automatically or manually, depending on your requirements.
Automatic Dropping
One of the key features of temporary tables is their automatic cleanup. When the session that created the temporary table ends, the table is automatically dropped, freeing up resources.
-- No explicit command needed; the table is dropped automatically when the session ends
This feature ensures that temporary tables do not persist beyond their intended use, maintaining database efficiency.
Manual Dropping
In some cases, you may want to drop a temporary table manually before the session ends. This can be done using the DROP TABLE
statement.
-- Manually drop the temporary table
DROP TABLE IF EXISTS #ProductDetails;
Manually dropping temporary tables is useful when you want to free up resources immediately or when the table is no longer needed within the session.
Best Practices and Common Pitfalls
Best Practices
Naming Conventions
Adopting clear and consistent naming conventions for temporary tables can greatly enhance the readability and maintainability of your SQL code. Here are some tips:
- Prefixing: Use a specific prefix like
#temp_
for local temporary tables and##temp_
for global temporary tables. This makes it immediately clear that the table is temporary. - Descriptive Names: Ensure the table name describes its purpose or the data it holds. For example,
#temp_SalesSummary
is more informative than#temp1
. - Avoiding Conflicts: Since temporary tables are session-specific, naming conflicts are rare, but it’s still good practice to use unique names within your session to avoid confusion.
CREATE TEMPORARY TABLE #temp_UserData (
UserID INT,
UserName VARCHAR(50),
UserEmail VARCHAR(100)
);
Resource Management
Efficient resource management is crucial when working with temporary tables to prevent unnecessary strain on the database server:
- Limit Scope: Only create temporary tables when absolutely necessary and drop them as soon as they are no longer needed. This helps free up resources promptly.
- Indexing: If you perform frequent lookups or joins on a temporary table, consider adding indexes to improve query performance. However, be mindful of the overhead associated with maintaining these indexes.
- Monitoring Usage: Regularly monitor the usage of temporary tables to ensure they are not consuming excessive resources. Tools and queries that track session activity can be useful here.
-- Drop the temporary table once it's no longer needed
DROP TABLE IF EXISTS #temp_UserData;
Common Pitfalls
Scope and Lifetime Issues
Understanding the scope and lifetime of temporary tables is essential to avoid unexpected behavior:
- Session-Specific Nature: Local temporary tables are only accessible within the session that created them. They are automatically dropped when the session ends. This means that if you need to share data between sessions, you should use global temporary tables.
- Global Temporary Tables: These are accessible across all sessions but are dropped only when the last session referencing them ends. Be cautious with their use to avoid unintended data persistence.
-- Example of creating a global temporary table
CREATE TEMPORARY TABLE ##temp_GlobalData (
DataID INT,
DataValue VARCHAR(100)
);
Performance Considerations
While temporary tables can significantly improve performance, misuse can lead to resource bottlenecks:
- Overuse: Creating too many temporary tables or using them for large datasets can consume significant memory and disk space, impacting overall database performance.
- Long-Lived Tables: Temporary tables that persist for long durations can tie up resources unnecessarily. Always ensure they are dropped as soon as their purpose is fulfilled.
- Complex Queries: While temporary tables simplify complex queries, overly complex temporary table operations can negate performance benefits. Always balance complexity with performance.
-- Example of dropping a temporary table to free up resources
DROP TABLE IF EXISTS ##temp_GlobalData;
By adhering to these best practices and being mindful of common pitfalls, you can leverage the full potential of temporary tables in the TiDB database, ensuring efficient and optimized database operations.
In summary, temporary tables in SQL are a powerful tool for managing intermediate data and simplifying complex queries. They offer significant benefits like performance improvement, data isolation, and enhanced query readability. We encourage you to practice creating and using temporary tables to fully leverage their capabilities in your database operations.
Feel free to share your experiences or ask questions in the comments below. Your insights and inquiries help us all learn and grow together. Happy querying!
See Also
Optimizing Databases: Step-by-Step SQL Partitioning Tutorial
Understanding SQL Data Structures
Streamlining SQL Formatting Using TiDB Playground