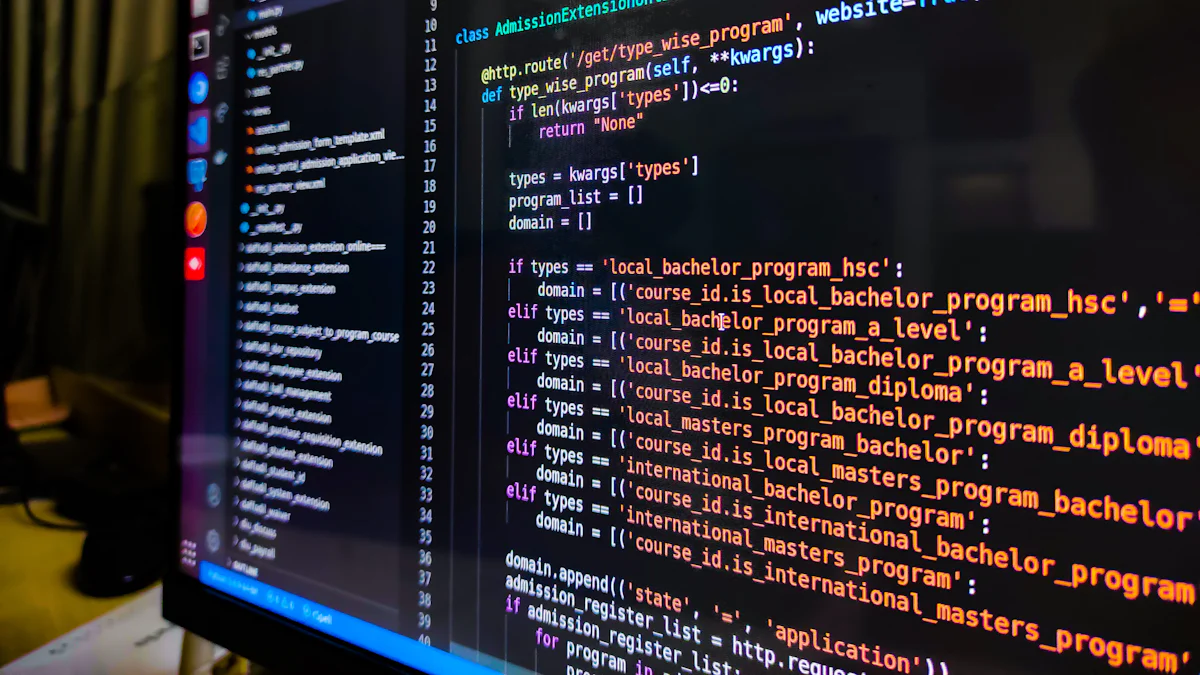
Building robust applications in Go is crucial for ensuring performance, scalability, and maintainability. One of the most popular tools for achieving this is GORM, an open-source ORM library that simplifies database interactions in Go. With over 30,400 stars on GitHub, GORM is widely adopted due to its developer-friendly design and comprehensive feature set. This blog aims to share best practices for using GORM effectively, helping you to build a Go app with GORM that is both efficient and reliable.
Getting Started with GORM
Setting Up Your Go Environment
Before diving into GORM, it’s essential to have your Go environment properly set up. This ensures a smooth development experience and helps avoid common pitfalls.
Installing Go
First, you need to install Go. You can download the latest version of Go from the official Go website. Follow these steps to install Go on your system:
-
Download the Installer:
- For Windows, download the
.msi
file. - For macOS, download the
.pkg
file. - For Linux, download the appropriate tarball for your distribution.
- For Windows, download the
-
Run the Installer:
- On Windows and macOS, simply run the downloaded file and follow the installation prompts.
- On Linux, extract the tarball and move the
go
directory to/usr/local
:tar -C /usr/local -xzf go1.x.x.linux-amd64.tar.gz
-
Set Up Environment Variables:
- Add Go’s binary directory to your
PATH
environment variable:export PATH=$PATH:/usr/local/go/bin
- Verify the installation by running:
go version
- Add Go’s binary directory to your
Setting Up a Go Workspace
A well-organized workspace is crucial for managing your Go projects efficiently. Here’s how to set up your Go workspace:
-
Create a Workspace Directory:
- Choose a directory where you will store all your Go projects. For example:
mkdir -p $HOME/go/{bin,src,pkg}
- Choose a directory where you will store all your Go projects. For example:
-
Set Up Environment Variables:
- Define the
GOPATH
environment variable to point to your workspace directory:export GOPATH=$HOME/go
- Add the workspace’s
bin
directory to yourPATH
:export PATH=$PATH:$GOPATH/bin
- Define the
-
Verify Your Workspace:
- Create a simple Go program to ensure everything is set up correctly:
mkdir -p $GOPATH/src/hello cd $GOPATH/src/hello echo 'package main; import "fmt"; func main() { fmt.Println("Hello, World!") }' > hello.go go run hello.go
- Create a simple Go program to ensure everything is set up correctly:
Installing and Configuring GORM
With your Go environment ready, the next step is to install and configure GORM. GORM is a powerful ORM library that simplifies database operations in Go applications.
Adding GORM to Your Project
To add GORM to your project, you need to use Go modules. Here’s how you can do it:
-
Initialize a New Go Module:
- Navigate to your project directory and initialize a new module:
go mod init your_project_name
- Navigate to your project directory and initialize a new module:
-
Install GORM and the Database Driver:
- Use the
go get
command to install GORM and the MySQL driver (or any other driver you need):go get -u gorm.io/gorm go get -u gorm.io/driver/mysql
- Use the
-
Verify Installation:
- Check the
go.mod
file to ensure that GORM and the driver have been added as dependencies.
- Check the
Basic Configuration Options
Configuring GORM correctly is crucial for optimal performance and ease of use. Here are some basic configuration options:
-
Connecting to the Database:
- Use the following code snippet to connect to a TiDB database:
package main import ( "gorm.io/driver/mysql" "gorm.io/gorm" "log" ) func main() { dsn := "user:password@tcp(host:port)/dbname?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { log.Fatal(err) } // Use db object for database operations }
- Use the following code snippet to connect to a TiDB database:
-
Setting Logger Levels:
- GORM provides various logging levels to help you debug your application:
import "gorm.io/gorm/logger" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{ Logger: logger.Default.LogMode(logger.Info), })
- GORM provides various logging levels to help you debug your application:
-
Connection Pooling:
- Configure connection pooling to manage database connections efficiently:
sqlDB, err := db.DB() if err != nil { log.Fatal(err) } sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour)
- Configure connection pooling to manage database connections efficiently:
By following these steps, you will have a solid foundation for using GORM in your Go projects. This setup not only ensures that your development environment is robust but also prepares you for more advanced GORM features and configurations. For more detailed information, refer to the official GORM documentation.
Best Practices for Database Design
Designing your database effectively is a cornerstone of building robust Go applications with GORM. Proper database design ensures data integrity, optimizes performance, and simplifies maintenance. Here, we will explore best practices for structuring your models and managing relationships using GORM.
Structuring Your Models
Models are the backbone of any ORM, and GORM is no exception. Structuring your models correctly can significantly impact the readability, maintainability, and performance of your application.
Defining Models in GORM
In GORM, models are defined using Go structs. Each struct represents a table in the database, and each field in the struct corresponds to a column. Here’s a simple example:
type User struct {
ID uint `gorm:"primaryKey"`
Name string `gorm:"size:255;not null"`
Email string `gorm:"uniqueIndex;size:255;not null"`
CreatedAt time.Time
UpdatedAt time.Time
}
- Primary Key: The
gorm:"primaryKey"
tag designates the primary key of the table. - Constraints: Tags like
gorm:"size:255;not null"
enforce constraints on the fields, ensuring data integrity. - Indexes: The
gorm:"uniqueIndex"
tag creates a unique index on theEmail
field, preventing duplicate entries.
By defining models this way, you leverage GORM’s ability to manage basic data types efficiently, mapping them seamlessly to database tables.
Using Tags for Field Customization
GORM tags provide a powerful mechanism for customizing field behavior. Here are some commonly used tags:
-
Column Name: Change the default column name.
type Product struct { Code string `gorm:"column:product_code"` }
-
Default Value: Set a default value for a field.
type Order struct { Status string `gorm:"default:'pending'"` }
-
Ignore Field: Exclude a field from the database schema.
type Customer struct { Password string `gorm:"-"` }
Using these tags effectively can help tailor your models to meet specific requirements, enhancing both functionality and performance.
Managing Relationships
Handling relationships between models is another critical aspect of database design. GORM simplifies this process with its intuitive relationship management features.
One-to-One Relationships
One-to-one relationships are defined using foreign keys and associations. Here’s an example:
type Profile struct {
ID uint
UserID uint
User User `gorm:"constraint:OnUpdate:CASCADE,OnDelete:SET NULL;"`
}
In this case, the Profile
model has a one-to-one relationship with the User
model. The gorm:"constraint:OnUpdate:CASCADE,OnDelete:SET NULL;"
tag ensures that changes to the User
model cascade to the Profile
model, maintaining referential integrity.
One-to-Many Relationships
One-to-many relationships are common in database design. Here’s how you can define them in GORM:
type Author struct {
ID uint
Name string
Books []Book `gorm:"foreignKey:AuthorID"`
}
type Book struct {
ID uint
Title string
AuthorID uint
}
In this example, an Author
can have multiple Books
. The gorm:"foreignKey:AuthorID"
tag specifies the foreign key in the Book
model, linking it to the Author
model.
Many-to-Many Relationships
Many-to-many relationships involve a join table to manage the associations. Here’s an example:
type Student struct {
ID uint
Name string
Courses []Course `gorm:"many2many:student_courses;"`
}
type Course struct {
ID uint
Name string
Students []Student `gorm:"many2many:student_courses;"`
}
In this scenario, the Student
and Course
models are linked through a join table named student_courses
. This setup allows for efficient querying and management of many-to-many relationships.
By adhering to these best practices for database design, you can ensure that your Go applications built with GORM are both robust and efficient. Properly structured models and well-managed relationships not only enhance performance but also make your code more maintainable and scalable.
Efficient Querying with GORM
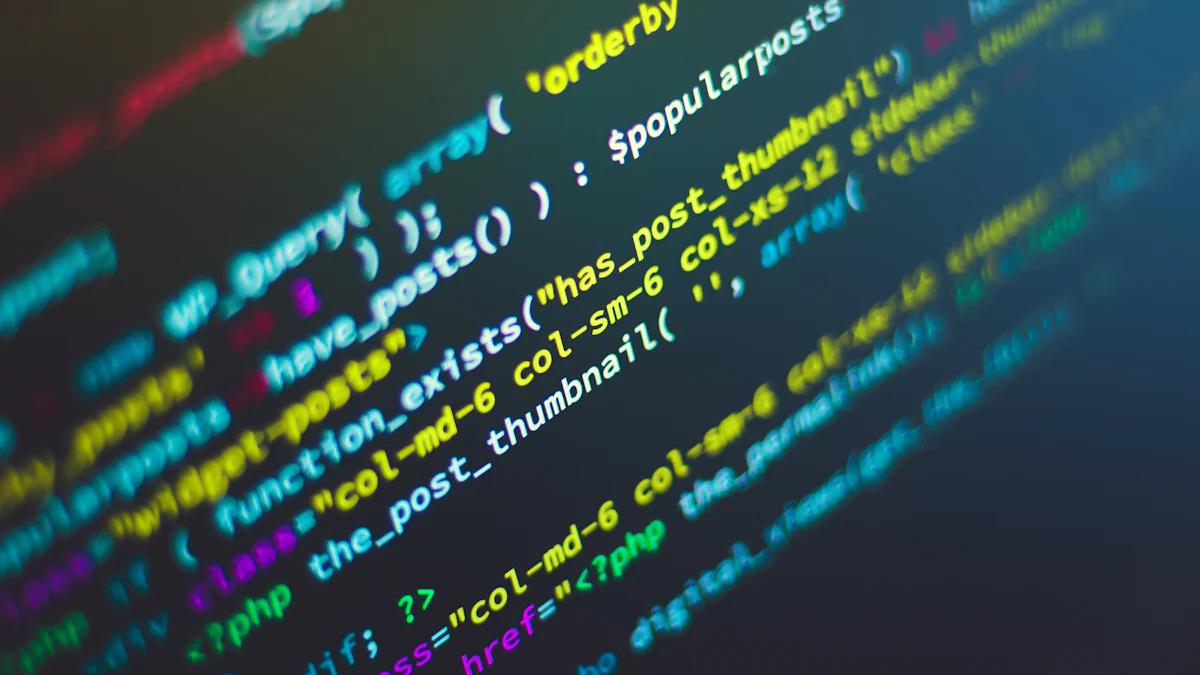
Efficient querying is a cornerstone of robust application development. GORM provides a range of features that make querying both intuitive and powerful. This section will guide you through basic and advanced querying techniques to optimize your Go applications.
Basic Queries
Retrieving Records
Retrieving records is one of the most fundamental operations in any database-driven application. GORM simplifies this process with straightforward methods:
var users []User
result := db.Find(&users)
if result.Error != nil {
log.Fatal(result.Error)
}
In this example, db.Find(&users)
retrieves all records from the users
table. The result
object contains useful metadata, such as the number of rows affected and any errors encountered.
Filtering and Sorting
Filtering and sorting are essential for narrowing down query results. GORM allows you to apply filters and sort results effortlessly:
var activeUsers []User
db.Where("status = ?", "active").Order("created_at desc").Find(&activeUsers)
Here, db.Where("status = ?", "active")
filters users with an active
status, and .Order("created_at desc")
sorts them by the created_at
field in descending order. This combination of filtering and sorting ensures that you retrieve only the relevant data, organized in the desired sequence.
Advanced Query Techniques
Joins and Preloading
Joins and preloading are advanced techniques for fetching related data efficiently. GORM supports both inner joins and left joins, as well as preloading associations:
var orders []Order
db.Preload("Customer").Find(&orders)
In this example, db.Preload("Customer")
fetches associated Customer
records for each Order
, reducing the number of queries and improving performance. For more complex scenarios, you can use joins:
var results []struct {
OrderID uint
CustomerName string
}
db.Table("orders").
Select("orders.id as order_id, customers.name as customer_name").
Joins("[left join](https://docs-archive.pingcap.com/tidb/v6.6/dev-guide-sample-application-golang) customers on customers.id = orders.customer_id").
Scan(&results)
This query uses a left join to combine orders
and customers
tables, selecting specific fields into a custom struct.
Transactions and Concurrency Control
Transactions are crucial for ensuring data integrity, especially in multi-step operations. GORM makes it easy to manage transactions:
tx := db.Begin()
if err := tx.Error; err != nil {
log.Fatal(err)
}
if err := tx.Create(&order).Error; err != nil {
tx.Rollback()
log.Fatal(err)
}
if err := tx.Create(&payment).Error; err != nil {
tx.Rollback()
log.Fatal(err)
}
if err := tx.Commit().Error; err != nil {
log.Fatal(err)
}
In this example, tx := db.Begin()
starts a new transaction. If any operation fails, tx.Rollback()
reverts all changes. Finally, tx.Commit()
applies the changes if all operations succeed.
Concurrency control is another critical aspect, particularly in high-traffic applications. GORM supports both optimistic and pessimistic locking:
-
Optimistic Locking: Uses a version field to detect conflicts.
type Product struct { ID uint Version int `gorm:"version"` } var product Product db.First(&product, 1) product.Version++ db.Save(&product)
-
Pessimistic Locking: Locks rows to prevent concurrent updates.
var product Product db.Clauses(clause.Locking{Strength: "UPDATE"}).First(&product, 1)
By leveraging these advanced techniques, you can ensure data consistency and optimize performance in your Go applications using GORM.
Performance Optimization
Optimizing performance is crucial for building robust Go applications with GORM. This section delves into techniques for indexing, caching, profiling, and monitoring to ensure your application runs efficiently.
Indexing and Caching
Creating Indexes
Indexes are fundamental for speeding up data retrieval and enhancing query performance. By creating indexes on frequently queried columns, you can significantly reduce the time it takes to fetch data.
-
Primary Indexes: Automatically created for primary key fields.
type User struct { ID uint `gorm:"primaryKey"` Name string `gorm:"index"` // Creates an index on the Name field }
-
Unique Indexes: Ensure that all values in a column are unique.
type Product struct { Code string `gorm:"uniqueIndex"` }
-
Composite Indexes: Combine multiple columns into a single index.
type Order struct { UserID uint `gorm:"index"` ProductID uint `gorm:"index"` gorm.Model }
Creating indexes helps the database engine quickly locate rows without scanning the entire table, which is especially beneficial for large datasets.
Implementing Caching Strategies
Caching is another powerful technique to improve performance by storing frequently accessed data in memory, reducing the need for repeated database queries.
-
In-Memory Caching: Use libraries like
groupcache
orfreecache
to store frequently accessed data in memory.import ( "github.com/coocood/freecache" ) cacheSize := 100 * 1024 * 1024 // 100MB cache := freecache.NewCache(cacheSize)
-
Distributed Caching: Implement distributed caching solutions like Redis to handle larger datasets and provide high availability.
import ( "github.com/go-redis/redis/v8" "context" ) rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379", }) ctx := context.Background() err := rdb.Set(ctx, "key", "value", 0).Err()
By combining indexing and caching strategies, you can significantly enhance the responsiveness and scalability of your Go applications using GORM.
Profiling and Monitoring
Using Profiling Tools
Profiling tools are essential for identifying performance bottlenecks in your application. They help you understand where your application spends most of its time and resources.
-
pprof: A popular profiling tool in Go that provides insights into CPU and memory usage.
import ( _ "net/http/pprof" "net/http" ) go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }()
-
GoTrace: Another powerful tool for tracing and visualizing the execution of your Go programs.
go tool trace trace.out
Using these tools, you can gather detailed performance metrics and make informed decisions to optimize your code.
Monitoring Database Performance
Monitoring the performance of your TiDB database is crucial for maintaining optimal application performance. Here are some best practices:
-
Prometheus and Grafana: Use Prometheus for collecting metrics and Grafana for visualizing them.
global: scrape_interval: 15s scrape_configs: - job_name: 'tidb' static_configs: - targets: ['localhost:9090']
-
TiDB Dashboard: Leverage the built-in TiDB Dashboard for real-time monitoring and performance analysis.
tiup cluster display <cluster-name>
-
Slow Query Log: Enable and analyze slow query logs to identify and optimize slow-running queries.
SET GLOBAL tidb_slow_log_threshold = 300;
By continuously profiling and monitoring your application and database, you can proactively address performance issues and ensure your Go applications remain robust and efficient.
Incorporating these performance optimization techniques will help you build high-performing Go applications with GORM, ensuring they scale effectively and maintain responsiveness under load.
Error Handling and Debugging
Error handling and debugging are critical aspects of building robust Go applications with GORM. Properly managing errors ensures your application can gracefully recover from unexpected situations, while effective debugging techniques help you identify and resolve issues quickly. This section will cover common errors in GORM and provide practical debugging strategies.
Common Errors in GORM
Handling Connection Issues
Connection issues are among the most frequent problems developers encounter when working with databases. These issues can stem from network problems, incorrect configuration, or database server downtime. Here are some best practices for handling connection issues in GORM:
-
Retry Logic: Implement retry logic to handle transient connection errors.
var db *gorm.DB var err error for i := 0; i < 3; i++ { db, err = gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err == nil { break } time.Sleep(2 * time.Second) } if err != nil { log.Fatalf("failed to connect to database: %v", err) }
-
Connection Pooling: Properly configure connection pooling to manage resources efficiently.
sqlDB, err := db.DB() if err != nil { log.Fatal(err) } sqlDB.SetMaxIdleConns(10) sqlDB.SetMaxOpenConns(100) sqlDB.SetConnMaxLifetime(time.Hour)
-
Graceful Shutdown: Ensure your application can gracefully handle shutdowns and restarts.
defer func() { sqlDB, err := db.DB() if err == nil { sqlDB.Close() } }()
By implementing these strategies, you can mitigate the impact of connection issues on your application’s stability.
Managing Data Integrity Errors
Data integrity errors occur when the data in your database does not meet the expected constraints or rules. These errors can lead to inconsistencies and application crashes. Here are some tips for managing data integrity errors:
-
Validation: Use GORM’s validation features to enforce data integrity at the application level.
type User struct { ID uint `gorm:"primaryKey"` Email string `gorm:"uniqueIndex;not null"` Age int `gorm:"check:age >= 18"` }
-
Error Handling: Capture and handle data integrity errors gracefully.
user := User{Email: "example@example.com", Age: 17} if err := db.Create(&user).Error; err != nil { log.Printf("error creating user: %v", err) }
-
Database Constraints: Define constraints at the database level to ensure data integrity.
ALTER TABLE users ADD CONSTRAINT chk_age CHECK (age >= 18);
By combining application-level validation with database constraints, you can maintain data integrity and prevent errors from propagating through your system.
Debugging Techniques
Using GORM’s Debug Mode
GORM provides a debug mode that logs all SQL statements generated by the ORM. This feature is invaluable for identifying issues related to SQL queries and understanding how GORM translates your code into SQL.
-
Enable Debug Mode: You can enable debug mode by calling the
Debug
method on your*gorm.DB
instance.db := db.Debug()
-
Analyze Logs: Review the logged SQL statements to identify any anomalies or performance bottlenecks.
[info] executing: SELECT * FROM `users` WHERE `users`.`id` = 1
Using GORM’s debug mode helps you gain insights into the ORM’s behavior and troubleshoot query-related issues effectively.
Logging and Analyzing Queries
Effective logging and analysis of queries are crucial for diagnosing and resolving issues in your application. Here are some best practices for logging and analyzing queries in GORM:
-
Custom Logger: Implement a custom logger to capture detailed information about each query.
import ( "gorm.io/gorm/logger" "log" "os" ) newLogger := logger.New( log.New(os.Stdout, "rn", log.LstdFlags), logger.Config{ SlowThreshold: time.Second, LogLevel: logger.Info, Colorful: true, }, ) db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{ Logger: newLogger, })
-
Slow Query Analysis: Identify and optimize slow queries by setting a threshold for logging slow queries.
newLogger := logger.New( log.New(os.Stdout, "rn", log.LstdFlags), logger.Config{ SlowThreshold: 200 * time.Millisecond, LogLevel: logger.Warn, Colorful: true, }, )
-
Query Metrics: Integrate with monitoring tools like Prometheus to collect and analyze query metrics.
import ( "github.com/prometheus/client_golang/prometheus" "github.com/prometheus/client_golang/prometheus/promhttp" "net/http" ) queryDuration := prometheus.NewHistogramVec( prometheus.HistogramOpts{ Name: "query_duration_seconds", Help: "Duration of database queries.", }, []string{"query"}, ) prometheus.MustRegister(queryDuration) http.Handle("/metrics", promhttp.Handler()) go http.ListenAndServe(":8080", nil)
By implementing these logging and analysis techniques, you can gain valuable insights into your application’s database interactions and optimize performance accordingly.
Building a Go App with GORM and TiDB
Integrating TiDB with GORM in your Go applications can significantly enhance performance, scalability, and ease of development. This section will guide you through setting up a TiDB cluster, connecting GORM to TiDB, and leveraging TiDB’s unique features to build a robust application.
Setting Up TiDB
Before you can start using TiDB with GORM, you need to set up a TiDB cluster. TiDB offers several deployment options, including serverless and dedicated clusters, to suit different needs.
Creating a TiDB Cluster
To create a TiDB cluster, follow these steps:
-
Choose Your Deployment Method:
- Serverless: Ideal for development and testing environments.
- Dedicated: Suitable for production environments requiring high availability and performance.
-
Create a Cluster:
- For a serverless cluster, visit the TiDB Cloud Console and follow the prompts to create a new cluster.
- For a dedicated cluster, you can use TiUP to deploy a local or cloud-based cluster.
-
Configure Access:
- Once your cluster is up and running, configure access by setting up user credentials and network permissions. Ensure you have the necessary connection details like host, port, user, and password.
Connecting GORM to TiDB
With your TiDB cluster ready, the next step is to connect GORM to TiDB. Here’s how you can do it:
-
Install Required Packages:
go get -u gorm.io/gorm go get -u gorm.io/driver/mysql
-
Set Up Connection String:
- Create a
.env
file in your project directory and add your TiDB connection details:TIDB_HOST='your_tidb_host' TIDB_PORT='4000' TIDB_USER='your_user' TIDB_PASSWORD='your_password' TIDB_DB_NAME='your_database' USE_SSL='true'
- Create a
-
Initialize GORM with TiDB:
- Use the following code snippet to connect GORM to your TiDB database:
package main import ( "gorm.io/driver/mysql" "gorm.io/gorm" "log" "os" ) func main() { dsn := os.Getenv("TIDB_USER") + ":" + os.Getenv("TIDB_PASSWORD") + "@tcp(" + os.Getenv("TIDB_HOST") + ":" + os.Getenv("TIDB_PORT") + ")/" + os.Getenv("TIDB_DB_NAME") + "?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { log.Fatal(err) } // Use db object for database operations }
- Use the following code snippet to connect GORM to your TiDB database:
Leveraging TiDB Features
TiDB offers several unique features that can be leveraged to optimize your Go applications. Here, we’ll focus on two key features: AUTO_RANDOM
and secondary indexes.
Using AUTO_RANDOM
The AUTO_RANDOM
feature in TiDB helps distribute data more evenly across the cluster, reducing hotspots and improving write performance. To use AUTO_RANDOM
with GORM, define your model as follows:
type User struct {
ID uint64 `gorm:"primaryKey;autoIncrement:false;column:id"`
Name string `gorm:"size:255;not null"`
}
func (User) TableName() string {
return "users"
}
In your SQL schema, you can enable AUTO_RANDOM
for the primary key:
CREATE TABLE users (
id BIGINT PRIMARY KEY AUTO_RANDOM,
name VARCHAR(255) NOT NULL
);
This setup ensures that new records are distributed evenly, enhancing performance and scalability.
Optimizing with Secondary Indexes
Secondary indexes are crucial for optimizing query performance, especially for large datasets. TiDB supports creating various types of indexes to speed up data retrieval.
-
Define Indexes in Your Model:
type Product struct { Code string `gorm:"uniqueIndex"` Price float64 }
-
Create Composite Indexes:
type Order struct { UserID uint `gorm:"index"` ProductID uint `gorm:"index"` gorm.Model }
-
Use SQL to Define Indexes:
CREATE INDEX idx_user_product ON orders (user_id, product_id);
By strategically using secondary indexes, you can significantly reduce query times and improve the overall performance of your application.
Leveraging these TiDB features allows you to build a Go app with GORM that is not only efficient but also scalable and robust, capable of handling high loads and complex queries with ease.
In this blog, we’ve explored key best practices for building robust Go applications with GORM, from setting up your environment to optimizing performance and handling errors. By applying these practices, you can simplify database interactions, enhance code maintainability, and improve overall application efficiency.
We encourage you to implement these strategies in your real-world projects. As Thirdfort highlights, adopting GORM allows developers to focus on application logic rather than database complexities, boosting productivity and system security.
Feel free to share your experiences and questions. Your feedback is invaluable in fostering a community of continuous improvement and innovation.
See Also
Optimal Strategies for Kubernetes Database Management
Transitioning Beyond MySQL: 5 Vital Factors for Growth and Speed
Constructing RAG App with LlamaIndex and TiDB Serverless Database