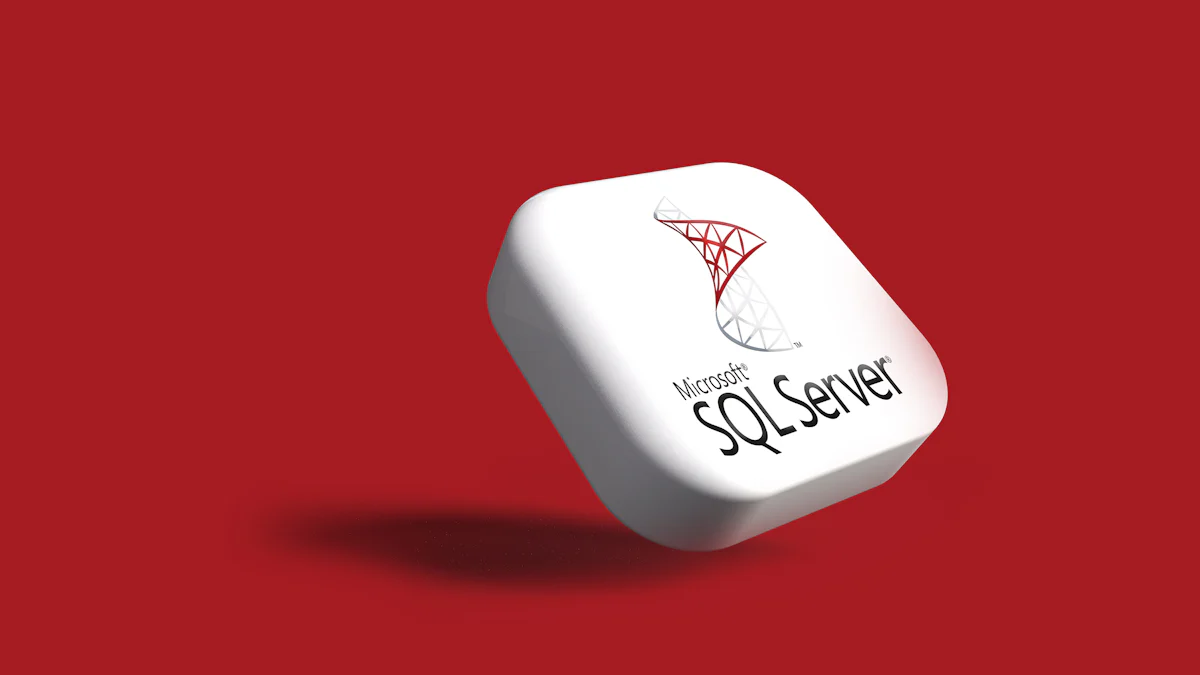
Counting rows in SQL tables is a fundamental task for database management, crucial for data analysis and reporting. With SQL being used by 88% of enterprise applications and *69% of professional developers worldwide*, understanding efficient methods to perform this operation is vital. The sql count rows function is a powerful tool that can enhance data handling capabilities. TiDB database, renowned for its MySQL compatibility and advanced features, offers robust solutions for SQL operations, ensuring high performance and scalability across diverse applications.
Understanding the Basics of SQL Count Rows
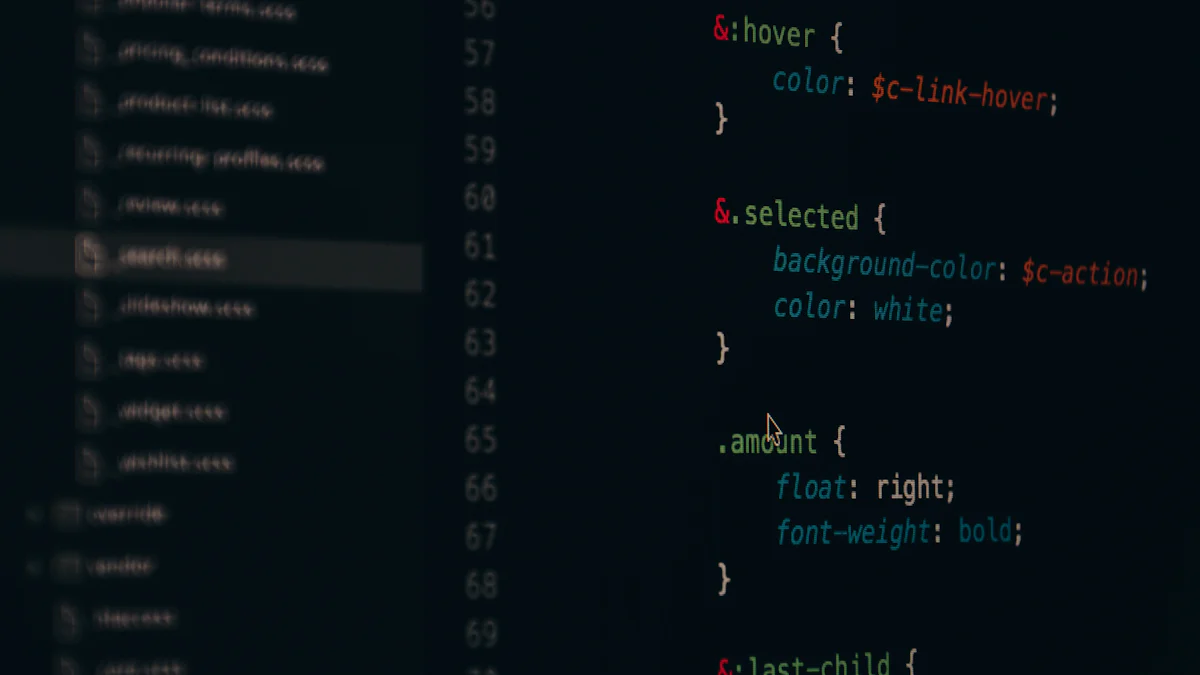
Counting rows in SQL tables is a fundamental operation that provides insights into data size and distribution. The SQL COUNT() Function is a versatile tool used to achieve this, offering various methods to cater to different needs. In this section, we will explore the basic usage of the sql count rows
function, including its syntax and practical applications.
Simple COUNT() Function
The SQL COUNT() Function is an aggregate function that returns the number of rows returned by a query. It is widely used for summarizing data and gaining insights into the size of a dataset. This function is available in all versions of modern SQL, making it a reliable choice for database management tasks.
Syntax and Usage
The syntax for the sql count rows
function is straightforward:
SELECT COUNT(*) FROM table_name;
COUNT(*)
: This form counts all rows in the specified table, including those with NULL values. It is the most commonly used variant when you need a total row count.COUNT(column_name)
: This variant counts only non-null values in the specified column. It is useful when you want to know how many entries exist in a particular field.
Basic Example
Let’s consider a simple example where we have a table named employees
:
SELECT COUNT(*) FROM employees;
This query will return the total number of rows in the employees
table. If you wish to count only the employees with a non-null email address, you can use:
SELECT COUNT(email) FROM employees;
This will give you the count of employees who have provided an email address, excluding any rows where the email is NULL.
COUNT() with Conditions
The SQL COUNT() Function becomes even more powerful when combined with conditions. By using the WHERE
clause, you can filter the rows that are counted based on specific criteria.
Using WHERE Clause
The WHERE
clause allows you to specify conditions that must be met for a row to be included in the count. This is particularly useful when you need to count rows that meet certain criteria.
SELECT COUNT(*) FROM employees WHERE department = 'Sales';
In this example, only employees in the ‘Sales’ department are counted. This approach is invaluable for generating reports or insights based on specific segments of your data.
Practical Example
Consider a scenario where you need to count the number of transactions above a certain value in a transactions
table:
SELECT COUNT(*) FROM transactions WHERE amount > 1000;
This query counts all transactions where the amount exceeds 1000, providing a quick way to assess high-value transactions.
By leveraging the SQL COUNT() Function with conditions, you can tailor your queries to extract meaningful insights from your data. Whether you’re working with large datasets or specific subsets, understanding how to effectively use the sql count rows
function is crucial for efficient database management.
Advanced Techniques for SQL Count Rows
As you delve deeper into SQL, mastering advanced techniques for counting rows becomes essential for effective data analysis and management. These techniques allow you to extract more nuanced insights from your datasets, enabling better decision-making and reporting.
COUNT() with GROUP BY
The COUNT()
function, when used in conjunction with GROUP BY
, becomes a powerful tool for summarizing data and understanding its distribution across different categories. This combination allows you to count occurrences of unique values within your dataset, providing a clear picture of how data is grouped.
Syntax Explanation
The basic syntax for using COUNT()
with GROUP BY
is as follows:
SELECT column_name, COUNT(*)
FROM table_name
GROUP BY column_name;
column_name
: The column by which you want to group the data.COUNT(*)
: Counts all rows within each group.
This syntax enables you to see how many rows fall into each category defined by the column_name
.
Example with Multiple Columns
Consider a scenario where you have a sales
table and you want to know how many sales transactions occurred in each region and for each product. You can achieve this with:
SELECT region, product, COUNT(*)
FROM sales
GROUP BY region, product;
This query groups the data by both region
and product
, providing a count of sales transactions for each unique combination. Such insights are invaluable for businesses looking to understand market performance and customer preferences.
Using Subqueries
Subqueries, or nested queries, offer another layer of sophistication in SQL operations. They allow you to perform complex calculations and filtering by embedding one query within another.
When to Use Subqueries
Subqueries are particularly useful when you need to:
- Filter results based on aggregated data.
- Perform calculations that depend on the results of another query.
- Simplify complex queries by breaking them into manageable parts.
They are a versatile tool in your SQL toolkit, enabling you to tackle intricate data challenges.
Example of Nested Queries
Imagine you have a transactions
table and you want to find the average transaction amount for each customer, but only for those customers who have made more than five transactions. A subquery can help:
SELECT customer_id, AVG(amount)
FROM transactions
WHERE customer_id IN (
SELECT customer_id
FROM transactions
GROUP BY customer_id
HAVING COUNT(*) > 5
)
GROUP BY customer_id;
In this example, the subquery identifies customers with more than five transactions. The outer query then calculates the average transaction amount for these customers. This approach allows you to derive meaningful insights from complex datasets, enhancing your analytical capabilities.
By mastering these advanced techniques, you can leverage the full potential of SQL to manage and analyze your data more effectively. Whether you’re summarizing data with GROUP BY
or utilizing subqueries for detailed analysis, these methods are essential for any data professional seeking to deepen their understanding of SQL.
Performance Considerations in TiDB
When working with large datasets, optimizing performance is crucial. TiDB database offers several strategies to enhance efficiency, ensuring smooth and fast operations.
Indexing for Efficiency
Indexes are vital for speeding up data retrieval processes. They play a significant role in how quickly you can count rows in a table.
How Indexes Affect COUNT()
Indexes can dramatically influence the performance of the COUNT()
function. By indexing columns that are frequently queried, you can reduce the time it takes to execute count operations. However, it’s essential to choose the right indexing strategy, especially when dealing with complex workloads involving numerous tables and queries.
TiDB Consulting Team: “Even experienced experts struggle to identify the most effective indexing strategy for intricate workloads involving numerous tables and countless SQL queries.”
To address this challenge, TiDB introduces tools like TiAdvisor, which automates index discovery, enhancing workload performance without the need for extensive manual intervention.
TiDB Team: “In this blog, we introduce TiDB Index Advisor—or TiAdvisor for short—a novel tool that automates index discovery to enhance user workload performance.”
Best Practices
- Use Composite Indexes: For queries involving multiple columns, composite indexes can be more efficient than single-column indexes.
- Regularly Review Index Usage: Analyze query patterns to ensure indexes are still relevant and beneficial.
- Leverage TiAdvisor: Utilize TiAdvisor to automate and optimize index selection, saving time and improving performance.
Handling Large Datasets with TiDB
Managing large datasets requires careful consideration to maintain performance and scalability.
Techniques for Optimization
- Horizontal Scalability: TiDB’s architecture allows for seamless scaling by adding more nodes, ensuring that performance remains consistent even as data grows.
- Utilize TiFlash: For analytical queries, TiFlash can significantly boost performance by storing data in a columnar format.
TiDB Team: “The tool ran in minutes and produced comparable performance to a manual process that took a couple of weeks!”
Real-world Examples
Consider a scenario where a company needs to analyze transaction data across multiple regions. By implementing TiFlash and leveraging TiAdvisor, they can efficiently handle large volumes of data, ensuring timely insights and decision-making.
Common Pitfalls and Solutions
When working with SQL, understanding the nuances of the COUNT()
function is essential to avoid common pitfalls. This section explores how to handle these challenges effectively.
Misunderstanding NULL Values
How COUNT() Handles NULLs
The COUNT()
function in SQL can be a bit tricky when it comes to NULL values. By default, COUNT(column_name)
only considers non-null entries within the specified column. This means that if you’re counting a column with NULL values, those rows will not be included in the count.
For example:
SELECT COUNT(email) FROM employees;
This query will count only the rows where the email
column is not NULL. If you need a comprehensive count of all rows, including those with NULL values, use COUNT(*)
.
Solutions and Workarounds
To ensure accurate results:
- Use
COUNT(*)
to include all rows, regardless of NULL values.
SELECT COUNT(*) FROM employees;
- If you need to count specific non-null fields, ensure your logic accounts for potential NULLs by using conditional expressions or default values.
Understanding these distinctions helps prevent errors in data analysis and ensures that your queries yield the expected results.
Overhead of Complex Queries
Identifying performance bottlenecks
Complex queries can often lead to performance issues, especially in large datasets. The overhead of such queries may result from inefficient indexing, poorly structured queries, or excessive use of nested subqueries.
To identify bottlenecks:
- Use the
EXPLAIN
statement to analyze query execution plans. - Monitor query performance metrics to pinpoint slow operations.
Tips for Improvement
Enhancing query performance requires a strategic approach:
-
Optimize Index Usage: Ensure that indexes are used effectively. TiDB database’s TiAdvisor tool can automate index optimization, saving time and improving efficiency.
-
Simplify Queries: Break down complex queries into simpler parts. Use subqueries judiciously to maintain clarity and performance.
-
Leverage TiFlash: For analytical workloads, TiFlash can significantly speed up query execution by utilizing columnar storage.
By addressing these common pitfalls, you can enhance the performance and accuracy of your SQL operations, ensuring efficient data management and analysis.
In this blog, we’ve explored various methods for counting rows in SQL tables, emphasizing the importance of selecting the right approach for your specific needs. Whether using simple COUNT()
functions or advanced techniques like GROUP BY
and subqueries, understanding these methods enhances your data management skills. Practice and experimentation are key to mastering these concepts. Leveraging TiDB database’s capabilities ensures efficient SQL operations, offering scalability and performance for complex workloads. Embrace these tools to unlock deeper insights and drive informed decision-making in your data-driven endeavors.