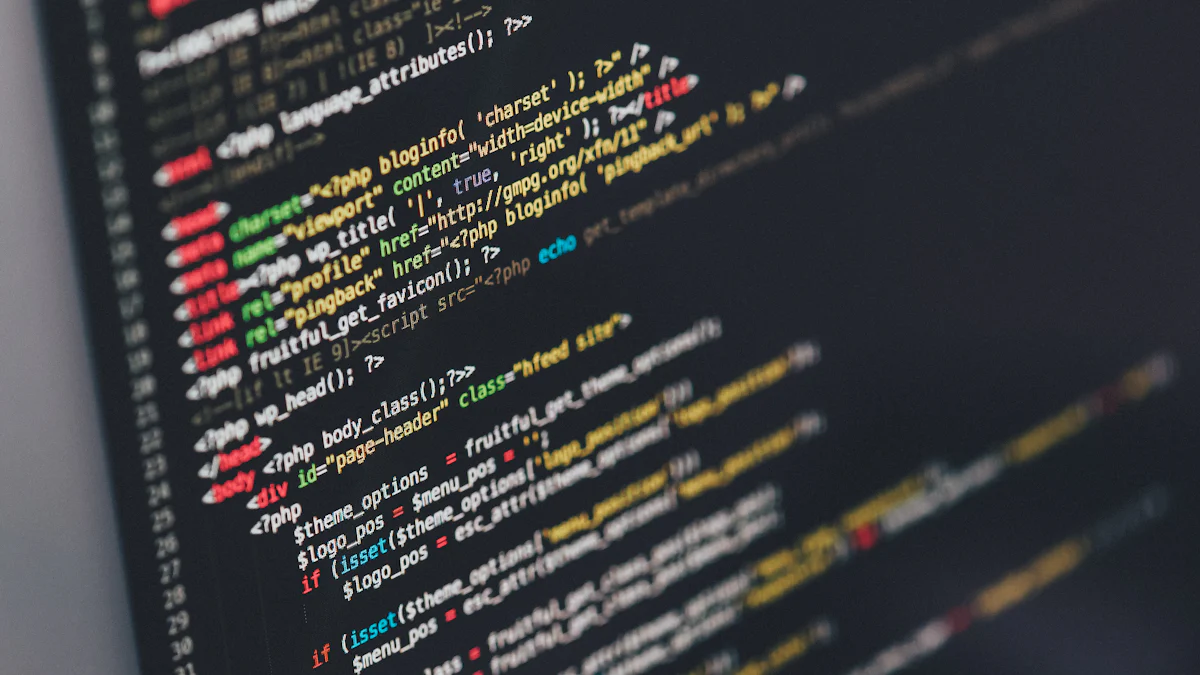
In the world of Python programming, SQLAlchemy Python serves as a fundamental tool for developers aiming to leverage the full potential of database interactions. As the most popular ORM in Python applications, it provides unmatched flexibility, enabling developers to effortlessly create intricate SQL expressions. Mastering advanced SQLAlchemy Python techniques is essential for efficient database management, allowing developers to optimize CRUD operations and effectively analyze SQL queries. Within this context, PingCAP’s TiDB database stands out as a premier solution, celebrated for its scalability and high availability, establishing a new benchmark in the database industry.
Understanding SQLAlchemy Core and ORM
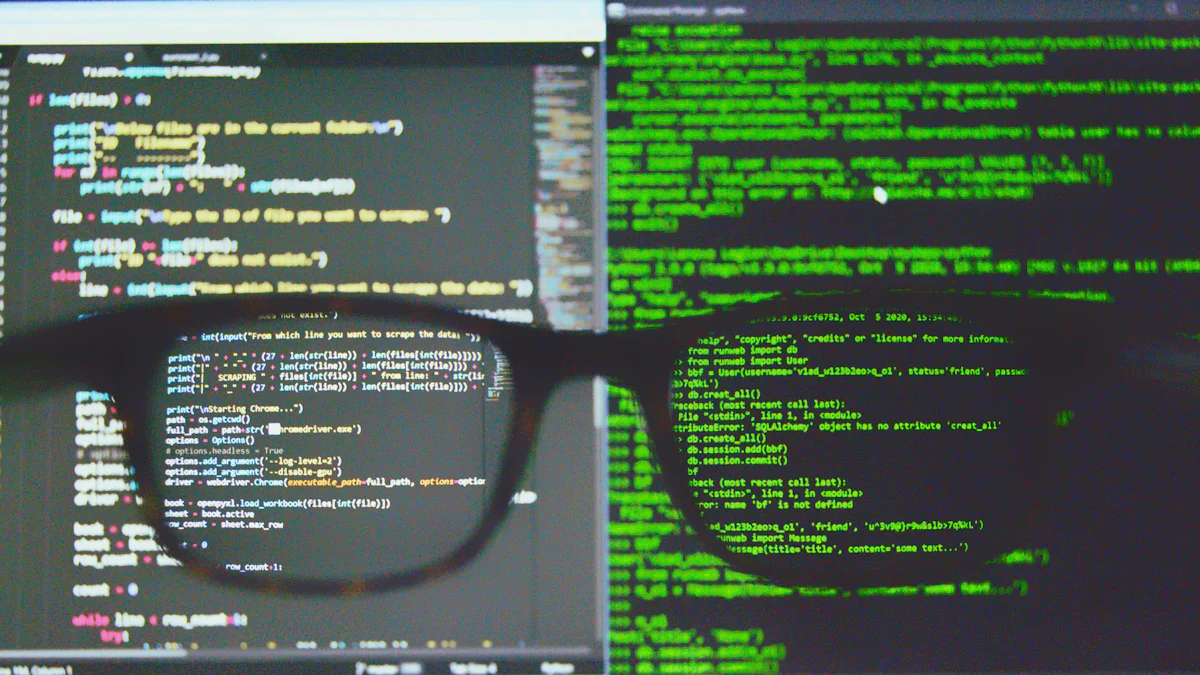
In the realm of Python programming, mastering SQLAlchemy is akin to wielding a powerful tool that can transform database interactions into seamless operations. This section delves into the two primary components of SQLAlchemy: Core and ORM, each offering distinct advantages for developers.
SQLAlchemy Core
Overview of SQLAlchemy Core
SQLAlchemy Core is the foundation of SQLAlchemy’s capabilities, providing a robust and flexible SQL expression language. It allows developers to construct SQL queries using Python constructs, offering a high degree of control over database interactions. This component is particularly beneficial for those who prefer working directly with SQL statements while still leveraging the power of Python.
SQLAlchemy Core’s architecture is designed to be lightweight and efficient, making it ideal for applications where performance is critical. By using Core, developers can create complex SQL expressions, manage transactions, and execute raw SQL queries with ease, all while maintaining the flexibility to switch between different database backends.
Benefits of using SQLAlchemy Core
The benefits of SQLAlchemy Core are manifold:
- Flexibility: Core provides the ability to write complex SQL queries using Python syntax, allowing for intricate data manipulation and retrieval.
- Performance: With its lightweight design, Core is optimized for high-performance applications, ensuring that database interactions are swift and efficient.
- Control: Developers have complete control over the SQL being executed, which is crucial for fine-tuning queries and optimizing performance.
By utilizing SQLAlchemy Core, developers can optimize database interactions in Python applications, ensuring that their applications are both scalable and high-performing.
SQLAlchemy ORM
Introduction to Object-Relational Mapping
Object-Relational Mapping (ORM) is a technique that allows developers to interact with a database using object-oriented programming principles. SQLAlchemy ORM abstracts the complexities of database interactions, enabling developers to work with Python objects instead of raw SQL queries. This abstraction simplifies the process of managing relationships between tables, making it easier to modify records and maintain data integrity.
The ORM layer of SQLAlchemy provides a declarative system for defining database schemas, allowing developers to map Python classes to database tables seamlessly. This mapping is not only intuitive but also enhances code readability and maintainability.
Advantages of using SQLAlchemy ORM
The advantages of using SQLAlchemy ORM are significant:
- Ease of Use: The ORM simplifies database interactions by allowing developers to work with Python objects, reducing the need to write complex SQL queries manually.
- Productivity: By abstracting the database layer, the ORM enables rapid development, allowing developers to focus on business logic rather than database intricacies.
- Maintainability: The declarative nature of the ORM makes it easier to manage and update database schemas, ensuring that applications remain adaptable to changing requirements.
By leveraging SQLAlchemy ORM, developers can build scalable and high-performing applications, optimizing database interactions and enhancing overall productivity. The combination of SQLAlchemy Core and ORM provides a comprehensive toolkit for mastering advanced SQLAlchemy techniques in Python, empowering developers to harness the full potential of the TiDB database.
Advanced Query Techniques with TiDB
When working with SQLAlchemy Python, mastering advanced query techniques is crucial for optimizing database interactions. The TiDB database, known for its scalability and high availability, offers a robust platform to implement these techniques effectively. This section delves into complex queries and query optimization strategies that enhance performance and efficiency in Python applications.
Complex Queries in SQLAlchemy Python
In the realm of SQLAlchemy Python, complex queries are pivotal for extracting meaningful insights from data. Two essential components of complex queries are joins and subqueries, and aggregate functions.
Using Joins and Subqueries
Joins and subqueries are powerful tools in SQLAlchemy Python that allow developers to retrieve data from multiple tables efficiently. Joins enable the combination of rows from two or more tables based on related columns, which is particularly useful when dealing with normalized databases. For instance, you might use an INNER JOIN
to fetch customer orders along with their details:
from sqlalchemy import select, join
stmt = select([orders, customers]).select_from(
join(orders, customers, orders.c.customer_id == customers.c.id)
)
Subqueries, on the other hand, allow you to nest queries within other queries, providing a way to perform operations like filtering or aggregation before the main query executes. This can be particularly beneficial when you need to calculate values or filter results dynamically.
Implementing Aggregate Functions
Aggregate functions such as SUM
, AVG
, COUNT
, and MAX
are integral to summarizing data in SQLAlchemy Python. They help in deriving insights by performing calculations on a set of values and returning a single value. For example, calculating the total sales for a specific period can be achieved using the SUM
function:
from sqlalchemy import func
stmt = select([func.sum(sales.amount)]).where(sales.date.between('2023-01-01', '2023-12-31'))
These functions, combined with the flexibility of SQLAlchemy Python, enable developers to write concise and efficient queries that can handle complex data retrieval scenarios.
Query Optimization in SQLAlchemy Python
Optimizing queries is essential for enhancing the performance of applications using the TiDB database. Efficient query execution and performance analysis are key aspects of this process.
Techniques for Efficient Query Execution
To ensure efficient query execution in SQLAlchemy Python, developers should focus on minimizing round-trips to the database and leveraging batch operations. By reducing the number of queries sent to the database, applications can achieve significant performance improvements. Utilizing SQLAlchemy’s bulk_insert_mappings
and bulk_update_mappings
methods can streamline operations involving large datasets.
Another best practice is implementing caching strategies to store frequently accessed data temporarily. This reduces the need to repeatedly query the database, thereby saving time and resources.
Analyzing Query Performance
Analyzing query performance is vital for identifying bottlenecks and optimizing SQLAlchemy Python applications. Tools like EXPLAIN in SQL can provide insights into how queries are executed, allowing developers to pinpoint areas for improvement. Additionally, monitoring query execution times and resource usage can help in fine-tuning queries for better performance.
By adopting these optimization techniques, developers can harness the full potential of the TiDB database, ensuring that their applications are both responsive and scalable. Mastering these advanced SQLAlchemy Python techniques not only enhances database interactions but also empowers developers to build robust and efficient applications.
Customizing SQLAlchemy Models
In the dynamic world of database management, customizing SQLAlchemy models is a vital skill for developers looking to tailor their applications to specific needs. This section explores advanced techniques in SQLAlchemy Python, focusing on model inheritance and custom data types, which are essential for creating flexible and efficient database schemas.
Model Inheritance
Model inheritance in SQLAlchemy Python allows developers to create a hierarchy of classes that map to database tables, facilitating code reuse and enhancing maintainability. This approach is particularly beneficial when dealing with complex data structures that share common attributes.
Single Table Inheritance
Single Table Inheritance is a straightforward approach where all classes in the hierarchy are stored in a single database table. This method is efficient as it reduces the number of joins required during queries. However, it may lead to sparse tables if subclasses have many unique fields.
In SQLAlchemy Python, implementing Single Table Inheritance involves defining a base class with a discriminator column to differentiate between subclasses. Here’s a simple example:
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import declarative_base
Base = declarative_base()
class Employee(Base):
__tablename__ = 'employee'
id = Column(Integer, primary_key=True)
name = Column(String(50))
type = Column(String(20))
__mapper_args__ = {
'polymorphic_identity': 'employee',
'polymorphic_on': type
}
class Manager(Employee):
__mapper_args__ = {
'polymorphic_identity': 'manager',
}
This setup allows you to query all employees or filter specifically for managers using the same table, showcasing the flexibility of SQLAlchemy Python.
Joined Table Inheritance
Joined Table Inheritance, on the other hand, stores each class in its own table, with subclasses having foreign key references to the parent table. This approach is ideal for scenarios where subclasses have distinct fields, ensuring data normalization and reducing redundancy.
In SQLAlchemy Python, Joined Table Inheritance is implemented by defining separate tables for each subclass:
class Engineer(Employee):
__tablename__ = 'engineer'
id = Column(Integer, ForeignKey('employee.id'), primary_key=True)
specialty = Column(String(50))
__mapper_args__ = {
'polymorphic_identity': 'engineer',
}
By leveraging Joined Table Inheritance, developers can maintain a clean and organized database schema, optimizing queries and ensuring efficient data retrieval.
Custom Data Types
Custom data types in SQLAlchemy Python provide the flexibility to define and use specialized data structures that go beyond standard SQL types. This capability is crucial for applications requiring unique data handling or storage formats.
Creating Custom Types
Creating custom types in SQLAlchemy Python involves subclassing the TypeDecorator
class and implementing the necessary methods to handle the conversion between Python objects and database values. For example, you might create a custom type to store JSON data:
from sqlalchemy.types import TypeDecorator, VARCHAR
import json
class JSONEncodedDict(TypeDecorator):
impl = VARCHAR
def process_bind_param(self, value, dialect):
if value is not None:
value = json.dumps(value)
return value
def process_result_value(self, value, dialect):
if value is not None:
value = json.loads(value)
return value
This custom type allows you to seamlessly store and retrieve JSON data in your SQLAlchemy Python models, enhancing the versatility of your application.
Using Custom Types in Models
Once a custom type is defined, it can be used in your SQLAlchemy Python models just like any other column type. This integration ensures that your application can handle complex data structures efficiently:
class Product(Base):
__tablename__ = 'product'
id = Column(Integer, primary_key=True)
name = Column(String(50))
attributes = Column(JSONEncodedDict)
By utilizing custom data types, developers can extend the functionality of their SQLAlchemy Python models, accommodating specific business requirements and improving data management.
Managing Transactions and Sessions with TiDB
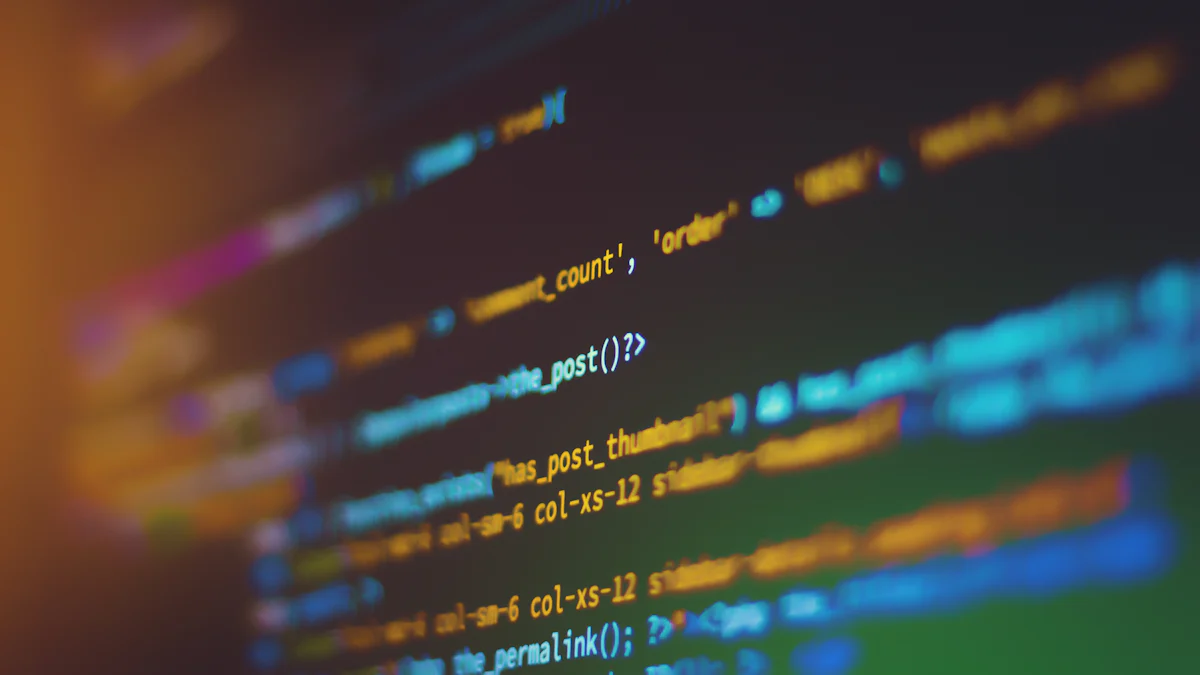
In the realm of database management, effectively handling transactions and sessions is crucial for maintaining data integrity and ensuring optimal performance. With SQLAlchemy Python, developers can leverage advanced techniques to manage these aspects seamlessly, especially when working with the robust TiDB database. This section delves into the intricacies of transaction and session management, providing insights and best practices for Python developers.
Transaction Management
Transactions are fundamental in ensuring that a series of operations on a database are executed reliably and consistently. In SQLAlchemy Python, understanding and implementing transactions correctly is key to maintaining data accuracy and relevance.
Understanding Transactions in SQLAlchemy
A transaction in SQLAlchemy Python represents a sequence of operations performed as a single unit of work. This means that either all operations within the transaction are completed successfully, or none are applied, ensuring data integrity. SQLAlchemy Python abstracts the complexity of transaction management, allowing developers to focus on the logic of their applications without worrying about the underlying database operations.
To minimize time wastage and optimize performance, it’s essential to understand the transaction state in SQLAlchemy Python. By doing so, developers can efficiently manage resources and ensure that transactions do not hold locks longer than necessary, which could lead to bottlenecks.
from sqlalchemy import create_engine
from sqlalchemy.orm import sessionmaker
engine = create_engine('mysql+pymysql://user:password@host/dbname')
Session = sessionmaker(bind=engine)
session = Session()
try:
# Begin a transaction
session.begin()
# Perform database operations
# ...
# Commit the transaction
session.commit()
except Exception as e:
# Rollback in case of error
session.rollback()
raise
finally:
# Close the session
session.close()
Implementing Transactional Operations
Implementing transactional operations in SQLAlchemy Python involves using the session
object to manage the lifecycle of a transaction. This includes beginning a transaction, committing changes, and rolling back in case of errors. By encapsulating operations within transactions, developers can ensure data consistency and handle errors gracefully.
The TiDB database supports distributed transactions using a two-phase commit protocol, which SQLAlchemy Python can leverage to maintain data integrity across multiple nodes. This capability is particularly beneficial for applications requiring high availability and strong consistency.
Session Management
Managing sessions effectively is another critical aspect of working with SQLAlchemy Python. Sessions serve as the interface between the application and the database, managing connections and transaction lifecycles.
Configuring Sessions
Configuring sessions in SQLAlchemy Python involves setting up the sessionmaker to bind to an engine, which represents the database connection. This configuration determines how sessions interact with the database, including connection pooling and transaction isolation levels.
from sqlalchemy.orm import scoped_session
# Configure a scoped session
session_factory = sessionmaker(bind=engine)
Session = scoped_session(session_factory)
Using a scoped session ensures that a single session is used per thread, which is crucial for web applications where multiple requests may be handled concurrently. This setup helps in managing resources efficiently and prevents conflicts arising from concurrent access.
Best Practices for Session Management
Adopting best practices for session management in SQLAlchemy Python can significantly enhance application performance and reliability:
Adopting best practices for session management in SQLAlchemy Python can significantly enhance application performance and reliability:
- Scope Sessions Appropriately: Ensure that sessions are scoped to the lifespan of a request or a unit of work. This prevents sessions from persisting longer than necessary, which could lead to resource leaks.
- Handle Exceptions Gracefully: Always include exception handling in your session management code to rollback transactions in case of errors, ensuring that the database remains in a consistent state.
- Optimize Connection Usage: Utilize connection pooling to manage database connections efficiently, reducing the overhead of establishing new connections for each transaction.
By following these best practices, developers can harness the full potential of the TiDB database, ensuring that their applications are both robust and efficient. Mastering these advanced SQLAlchemy Python techniques empowers developers to build scalable and high-performing applications, optimizing database interactions and enhancing overall productivity.
In this journey through advanced SQLAlchemy Python techniques, we’ve explored the intricacies of Core and ORM, delved into complex queries, and optimized database interactions. These skills are invaluable for any developer aiming to build efficient and scalable applications. By applying these techniques, you can significantly enhance your database management capabilities in Python.
As you continue to explore and practice, remember the robust features of the TiDB database. Its scalability and high availability make it an ideal companion for your SQLAlchemy Python endeavors. Embrace these tools and techniques, and watch your applications thrive.