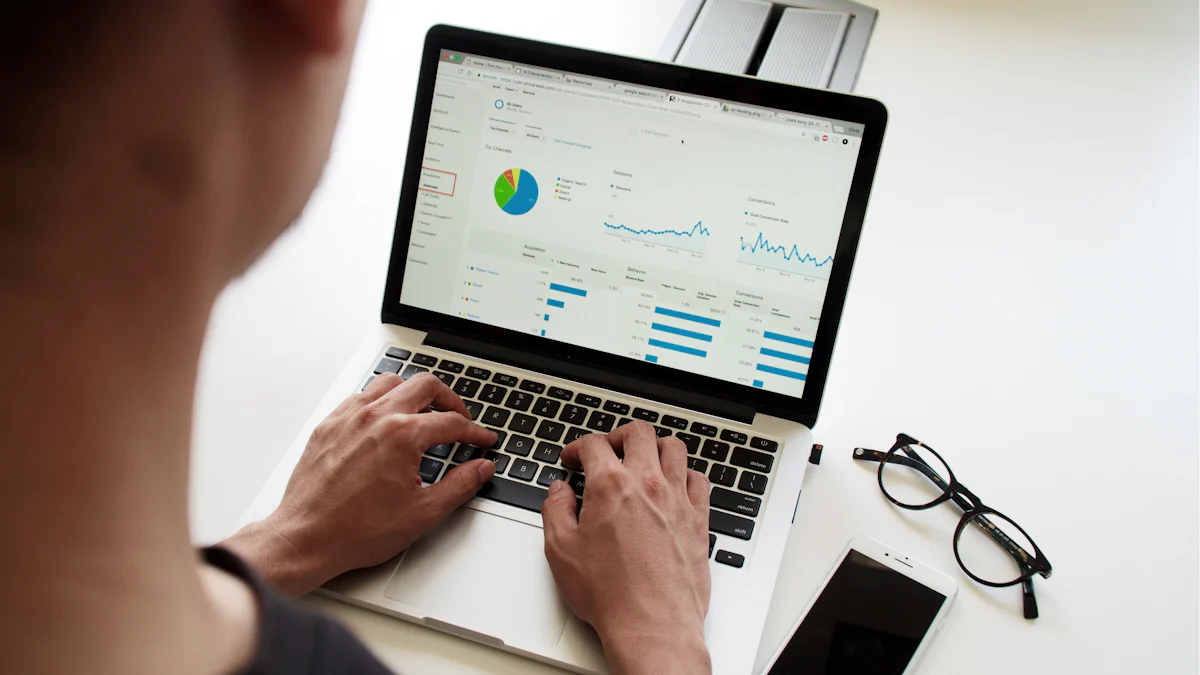
In the realm of SQL, the concept of “upsert SQL” serves as a potent mechanism that merges the functionalities of updating and inserting data. This operation is crucial for preserving data integrity and enhancing database performance by minimizing the necessity for multiple queries. Upsert SQL facilitates effortless data management, guaranteeing that records are either updated or inserted depending on their presence in the database. This feature is especially advantageous in high-transaction settings, where efficiency and speed are critical. By mastering upsert SQL, database administrators can greatly improve the performance and dependability of their systems.
What is an Upsert?
Definition and Purpose
Explanation of Upsert Operations
The term “upsert” is a blend of the words “update” and “insert.” It describes a database operation that either updates an existing row if a specified value already exists in a table or inserts a new row if it doesn’t. This dual functionality allows for more efficient data management by combining two operations into one. In essence, upserts guarantee that your data is either updated or inserted in a single atomic action, which is particularly beneficial in maintaining data integrity and consistency.
Benefits of Using Upserts
Utilizing upserts offers several advantages:
-
Simplified Query Logic: By consolidating insert and update operations, upserts reduce the complexity of your SQL queries. This simplification can lead to fewer errors and easier maintenance.
-
Reduced Database Round-Trips: Instead of executing separate insert and update statements, an upsert operation minimizes the number of interactions with the database. This reduction can significantly improve performance, especially in high-transaction environments.
-
Enhanced Data Integrity: Upserts ensure that data remains consistent and accurate by atomically handling potential conflicts, such as duplicate entries. This feature is crucial for applications where data consistency is paramount, like in PostgreSQL’s UPSERT operation.
Upsert vs. Insert and Update
Key Differences
While inserts and updates are fundamental SQL operations, upserts provide a unique advantage by merging these two actions. Here’s how they differ:
-
Insert: Adds a new record to the database. If the record already exists, it typically results in an error unless handled explicitly.
-
Update: Modifies existing records but does not add new ones. If the record doesn’t exist, no action is taken.
-
Upsert: Combines both functionalities by checking for the existence of a record. It updates the record if it exists or inserts a new one if it doesn’t.
Use Cases for Each Operation
Understanding when to use each operation is vital for effective database management:
-
Insert: Best used when adding entirely new data without concern for existing records. Ideal for initial data loads or when duplicates are not possible.
-
Update: Suitable for modifying specific fields in existing records. Use this when you’re sure the record already exists and you need to change its attributes.
-
Upsert: Perfect for scenarios where data consistency and integrity are critical, such as in data pipelines or real-time applications. Upserts are particularly useful when dealing with potential duplicates, ensuring that your database remains accurate and up-to-date without additional logic to handle conflicts.
By leveraging upserts, database administrators can streamline operations, enhance performance, and maintain robust data integrity across various SQL databases, including TiDB database, MySQL, and PostgreSQL.
Implementing Upserts in Different SQL Databases
Understanding how to implement upsert operations across various databases is crucial for database administrators and developers. Each database management system (DBMS) has its unique syntax and methods for executing upsert operations, which can significantly impact performance and data integrity. Let’s explore how upsert SQL is implemented in MySQL, PostgreSQL, and SQL Server.
Upserts in MySQL
Syntax and Queries
In MySQL, the upsert operation is typically achieved using the INSERT ... ON DUPLICATE KEY UPDATE
statement. This approach allows you to insert a new row into a table or update an existing row if a duplicate key conflict occurs.
INSERT INTO table_name (column1, column2)
VALUES (value1, value2)
ON DUPLICATE KEY UPDATE column1 = value1, column2 = value2;
This syntax checks for a duplicate key before deciding whether to insert a new record or update an existing one, making it a powerful tool for maintaining data consistency.
Practical Examples
Consider a scenario where you manage a user database and need to ensure that user information is always up-to-date. Using MySQL’s upsert SQL, you can efficiently handle this:
INSERT INTO users (user_id, email)
VALUES (1, 'newemail@example.com')
ON DUPLICATE KEY UPDATE email = 'newemail@example.com';
This query will update the email of the user with user_id
1 if they already exist, or insert a new user if they don’t.
Upserts in PostgreSQL
Syntax and Queries
PostgreSQL offers a robust implementation of upsert SQL through the [INSERT ON CONFLICT](https://www.cockroachlabs.com/blog/sql-upsert/)
clause. This feature provides a seamless way to handle conflicts by specifying actions to take when a conflict arises.
INSERT INTO table_name (column1, column2)
VALUES (value1, value2)
ON CONFLICT (conflict_target) DO UPDATE SET column1 = value1, column2 = value2;
The ON CONFLICT
clause allows you to define how to resolve conflicts, ensuring data integrity and reducing the complexity of your queries.
Practical Examples
Imagine you’re managing an inventory database and need to update product quantities without creating duplicates. PostgreSQL’s upsert SQL can be utilized as follows:
INSERT INTO inventory (product_id, quantity)
VALUES (101, 50)
ON CONFLICT (product_id) DO UPDATE SET quantity = inventory.quantity + 50;
This query will add 50 to the existing quantity of the product with product_id
101 if it exists, or insert a new record if it doesn’t.
Upserts in SQL Server
Syntax and Queries
In SQL Server, the MERGE
statement is used to perform upsert operations. This statement allows you to merge data from a source table into a target table, handling inserts and updates based on specified conditions.
MERGE INTO target_table AS target
USING source_table AS source
ON target.key_column = source.key_column
WHEN MATCHED THEN
UPDATE SET target.column1 = source.column1
WHEN NOT MATCHED THEN
INSERT (column1, column2) VALUES (source.column1, source.column2);
The MERGE
statement provides a flexible way to implement upsert SQL, enabling complex data transformations and integrations.
Practical Examples
Suppose you have a sales database and want to update sales records or add new ones as needed. SQL Server’s MERGE
statement can be employed like this:
MERGE INTO sales AS target
USING (SELECT 1 AS sale_id, 100 AS amount) AS source
ON target.sale_id = source.sale_id
WHEN MATCHED THEN
UPDATE SET target.amount = source.amount
WHEN NOT MATCHED THEN
INSERT (sale_id, amount) VALUES (source.sale_id, source.amount);
This operation ensures that the sales record is updated if it exists or inserted if it’s new, streamlining data management processes.
By mastering upsert SQL across these popular databases, you can enhance your ability to maintain data integrity and optimize database performance effectively.
Common Challenges and Best Practices
In the realm of database management, implementing upserts can present certain challenges. However, by understanding these challenges and adopting best practices, you can ensure that your upsert operations are both efficient and effective.
Handling Conflicts
Handling conflicts is a critical aspect of executing upsert operations. Conflicts typically arise when there is an attempt to insert a record that violates unique constraints or primary keys.
Conflict Resolution Strategies
To manage conflicts effectively, consider the following strategies:
-
Use of Unique Constraints: Ensure that your tables are designed with appropriate unique constraints. This setup will help in identifying potential conflicts during upsert operations.
-
Leverage Database-Specific Features: Different databases offer unique features for conflict resolution. For instance, PostgreSQL’s
ON CONFLICT
clause allows you to specify how to handle conflicts, such as updating existing records or ignoring the insert. -
Atomic Operations: Implement atomic operations to ensure that upserts are completed in a single transaction, thus maintaining data integrity even in the event of a conflict.
Examples of Conflict Handling
Consider a scenario where you’re managing a customer database, and you need to update customer details without creating duplicates:
INSERT INTO customers (customer_id, email)
VALUES (123, 'newemail@example.com')
ON CONFLICT (customer_id) DO UPDATE SET email = 'newemail@example.com';
This PostgreSQL query efficiently resolves conflicts by updating the email if the customer ID already exists, ensuring data consistency and accuracy.
Performance Considerations
Optimizing the performance of upsert operations is crucial, especially in high-transaction environments where efficiency is paramount.
Optimizing Upsert Operations
To enhance the performance of your upserts, consider these optimization techniques:
-
Indexing: Proper indexing can significantly speed up the search process for existing records, reducing the time required for upsert operations.
-
Batch Processing: Whenever possible, perform upserts in batches rather than individually. This approach reduces the overhead of multiple database calls and improves throughput.
-
Database Configuration: Adjust database configurations to optimize performance. For example, tuning parameters related to write operations can lead to more efficient upserts.
Tips for Efficient Upserts
Here are some practical tips to ensure your upserts are executed efficiently:
-
Monitor and Analyze: Regularly monitor your database performance and analyze upsert operations to identify bottlenecks. Tools like query analyzers can provide insights into areas needing improvement.
-
Use Appropriate SQL Constructs: Select the right SQL constructs based on your DBMS. For example, use
MERGE
in SQL Server for complex upsert scenarios, as it provides a flexible way to handle both inserts and updates. -
Test and Iterate: Continuously test your upsert strategies in a controlled environment before deploying them in production. This practice helps in identifying potential issues and refining your approach.
By adopting these best practices, you can effectively manage conflicts and optimize the performance of your upsert operations, ensuring that your database remains robust and efficient.
In conclusion, mastering the concept of upserts in SQL is a vital skill for database administrators and developers. By understanding how to effectively implement upserts, you can streamline data operations, reduce redundancy, and maintain data integrity across various platforms like MySQL, PostgreSQL, and SQL Server. This knowledge empowers you to handle complex scenarios efficiently, such as managing inventory updates or ensuring accurate customer records. Embrace these insights and apply them to real-world situations to enhance your database management capabilities and drive performance improvements.