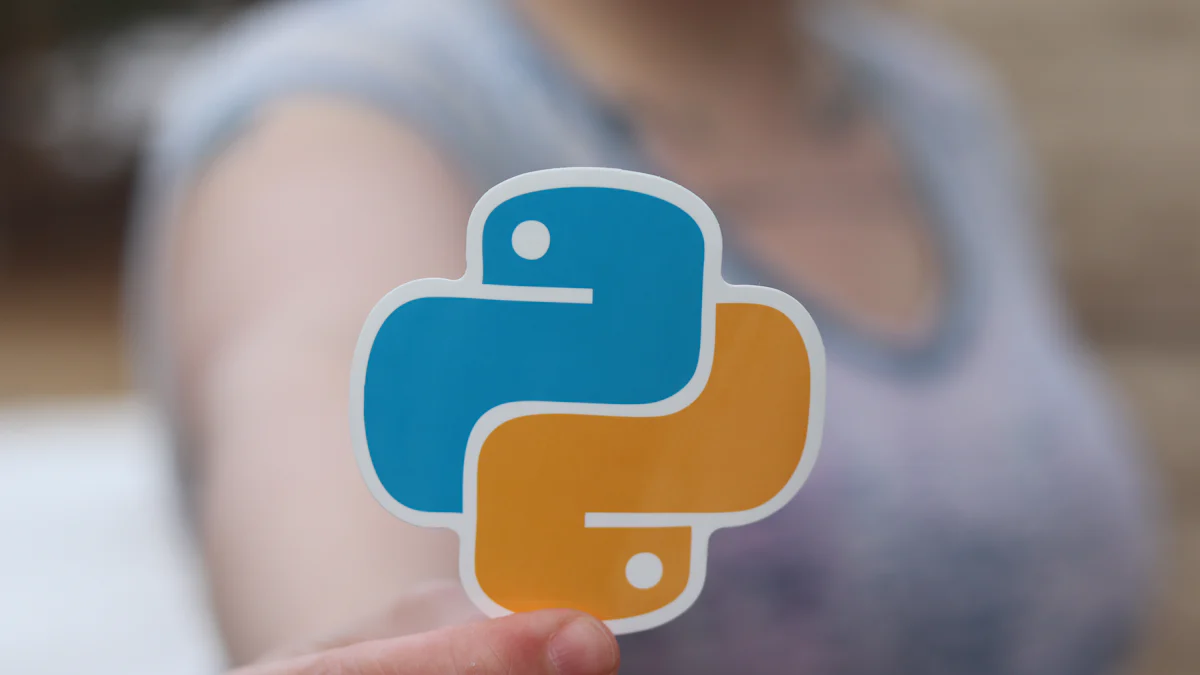
An inverted index is a powerful data structure that revolutionizes how we retrieve information. By mapping content, such as words, to their locations in documents, it allows for fast and efficient query processing. This efficiency is crucial in search engines and databases, enabling them to locate relevant information quickly without scanning entire collections. Compared to the brute-force approach, an inverted index can be up to 50 seconds faster with 1000 lines of data. In this guide, we’ll explore the steps to build an inverted index in Python, enhancing your ability to handle large-scale data efficiently.
Understanding the Inverted Index
Definition and Purpose
What is an Inverted Index?
An inverted index is a fundamental data structure in information retrieval systems, designed to map words to their occurrences within a set of documents. Imagine it as a giant table where each word from your document collection is listed alongside the documents in which it appears. This setup allows for rapid querying, as it eliminates the need to scan entire documents to locate specific terms. By structuring data in this way, inverted indexes enable efficient full-text searches, making them indispensable in environments where quick access to large volumes of text is required.
Why Use an Inverted Index?
The primary advantage of using an inverted index lies in its ability to significantly speed up query processing. When a search query is made, the system can quickly refer to the index to find relevant documents, bypassing the need to examine each document individually. This efficiency is particularly beneficial in search engines and database management systems, where the volume of data can be immense. Moreover, inverted indexes support various types of queries, including phrase searches and proximity searches, enhancing their versatility in handling complex information retrieval tasks.
Applications of Inverted Index
Search Engines
In the realm of search engines, inverted indexes are the backbone of indexing algorithms. They allow search engines to process queries at lightning speed by mapping search terms directly to the documents containing them. This capability not only optimizes query speed but also improves the accuracy of search results by ensuring that relevant documents are retrieved quickly. As a result, users experience faster and more precise search outcomes, which is crucial in today’s data-driven world.
Database Management
In database management, inverted indexes play a pivotal role in optimizing data retrieval processes. By employing this data structure, databases can efficiently handle full-text searches across vast datasets. This is particularly useful in applications requiring real-time data access, such as those supported by PingCAP’s TiDB database. The ability to swiftly retrieve and analyze data enhances the overall performance of database systems, making them more responsive to user queries and capable of supporting complex analytical tasks.
Preparing Your Data
Before diving into the construction of an inverted index, it’s crucial to prepare your data meticulously. This preparation involves two key stages: collecting the right data and cleaning it to ensure accuracy and relevance.
Data Collection
Effective data collection is the foundation of building a robust inverted index. It involves gathering data from various sources and understanding the types of data you’ll be working with.
Sources of Data
Data can be sourced from multiple avenues depending on the application:
- Web Scraping: Extracting data from websites using tools like Beautiful Soup or Scrapy.
- APIs: Leveraging public APIs to access structured data.
- Databases: Utilizing existing databases, such as PingCAP’s TiDB database, which supports efficient data retrieval and management.
- Files: Reading from text files, CSVs, or JSON files stored locally or in cloud storage.
Each source has its own set of challenges and benefits. For instance, web scraping provides vast amounts of data but requires handling HTML structures, while APIs offer structured data but may have rate limits.
Types of Data
Understanding the types of data is equally important:
- Structured Data: Organized in a fixed schema, such as tables in a database.
- Unstructured Data: Includes free-form text, such as emails or social media posts.
- Semi-Structured Data: Contains elements of both, like JSON or XML files.
The type of data influences how you will process and clean it. For example, unstructured data often requires more extensive cleaning and normalization.
Data Cleaning
Once collected, data must be cleaned to remove any inconsistencies or irrelevant information. This step ensures that the inverted index is accurate and efficient.
Removing Noise
Noise in data refers to irrelevant or redundant information that can skew results. Common noise includes:
- Stop Words: Commonly used words (e.g., “and”, “the”) that add little value to searches.
- Punctuation: Special characters that can disrupt tokenization.
- HTML Tags: When dealing with web-scraped data, removing HTML tags is essential.
Removing noise enhances the quality of the data, making the inverted index more effective in retrieving relevant documents.
Normalizing Data
Normalization involves standardizing data to ensure consistency:
- Lowercasing: Converting all text to lowercase to avoid case-sensitive discrepancies.
- Stemming and Lemmatization: Reducing words to their base or root form (e.g., “running” to “run”).
- Handling Synonyms and Ambiguity: Addressing variations in word usage to improve search accuracy.
These steps are crucial for overcoming issues like spelling errors and synonyms, which can affect the performance of an inverted index. By normalizing data, you ensure that the index accurately reflects the content of the documents, leading to more precise search results.
Building the Inverted Index
Constructing an inverted index is a meticulous process that involves breaking down your text data into manageable components and organizing it for efficient retrieval. This section will guide you through the critical steps of tokenization and index construction, ensuring you have a robust foundation for your inverted index.
Tokenization
Tokenization is the initial step in building an inverted index, where the text is divided into smaller units, or tokens. These tokens form the basis of the index, allowing for precise mapping of terms to their respective documents.
Splitting Text into Tokens
The process of splitting text into tokens involves parsing the text and identifying individual words or terms. This can be achieved using Python libraries such as NLTK or spaCy, which offer powerful tools for text processing. The goal is to break down the text into meaningful components while preserving the context of each word. For instance, by using whitespace and punctuation as delimiters, you can effectively isolate words and prepare them for indexing.
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "Building an inverted index requires careful planning."
tokens = word_tokenize(text)
print(tokens)
# Output: ['Building', 'an', 'inverted', 'index', 'requires', 'careful', 'planning', '.']
Handling Special Characters
Special characters, such as punctuation marks and symbols, can disrupt the tokenization process if not handled properly. It’s essential to clean these characters from your text to ensure that your tokens are accurate and relevant. Removing punctuation and converting text to lowercase are common practices that enhance the quality of the tokens.
import re
def clean_text(text):
# Remove punctuation and convert to lowercase
text = re.sub(r'[^ws]', '', text).lower()
return text
cleaned_text = clean_text("Building an inverted index requires careful planning.")
tokens = word_tokenize(cleaned_text)
print(tokens)
# Output: ['building', 'an', 'inverted', 'index', 'requires', 'careful', 'planning']
Index Construction
Once tokenization is complete, the next step is to construct the inverted index itself. This involves mapping each token to the documents in which it appears, creating a structured representation of your data.
Mapping Terms to Documents
Mapping terms to documents is a crucial aspect of index construction. Each token is associated with a list of document identifiers, indicating where the term appears. This mapping allows for quick retrieval of documents based on search queries. In Python, this can be implemented using dictionaries, where keys are tokens and values are lists of document IDs.
from collections import defaultdict
def build_inverted_index(docs):
inverted_index = defaultdict(list)
for doc_id, text in enumerate(docs):
tokens = word_tokenize(clean_text(text))
for token in tokens:
if doc_id not in inverted_index[token]:
inverted_index[token].append(doc_id)
return inverted_index
documents = [
"Building an inverted index requires careful planning.",
"An inverted index maps terms to document locations."
]
index = build_inverted_index(documents)
print(index)
# Output: {'building': [0], 'an': [0, 1], 'inverted': [0, 1], 'index': [0, 1], ...}
Storing the Index
Storing the inverted index efficiently is vital for performance, especially when dealing with large datasets. The index can be stored in various formats, such as JSON or databases like PingCAP’s TiDB database, which supports scalable and high-performance data storage. Choosing the right storage solution ensures that your inverted index remains accessible and responsive to queries.
import json
# Convert the inverted index to JSON format for storage
index_json = json.dumps(index)
with open('inverted_index.json', 'w') as f:
f.write(index_json)
By following these steps, you can successfully build an inverted index that enhances the efficiency of information retrieval in your applications. This structured approach not only improves query performance but also lays the groundwork for advanced search capabilities.
Implementing in Python with PingCAP’s TiDB
In this section, we’ll delve into the practical implementation of an inverted index using Python, leveraging the capabilities of PingCAP’s TiDB database. This powerful database solution not only supports efficient data storage but also enhances the performance of your inverted index through its robust features.
Setting Up the Environment
Before we dive into coding, it’s essential to set up the right environment. This involves ensuring you have all the necessary libraries and tools installed.
Required Libraries
To build an inverted index and integrate it with TiDB, you’ll need a few key libraries:
- MySQL Connector/Python: As TiDB is MySQL-compatible, this library will facilitate communication between Python and your TiDB database.
- NLTK: For tokenization and text processing.
- pandas: To handle data manipulation and analysis.
- json: For handling JSON data formats, useful for storing and retrieving the inverted index.
Installation Steps
Setting up your environment involves installing these libraries. You can do this using pip
, Python’s package manager:
pip install mysql-connector-python nltk pandas
Additionally, ensure that your TiDB Cloud account is set up if you’re using the cloud-based service. This will allow you to focus on building your application without worrying about the complexities of database management.
Writing the Code
With the environment ready, let’s move on to writing the code for building and storing the inverted index using TiDB.
Code Snippets for Tokenization
Tokenization is the first step in creating an inverted index. We’ll use the NLTK library to break down text into tokens:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def tokenize_text(text):
return word_tokenize(text.lower())
# Example usage
text = "Implementing an inverted index with TiDB is efficient."
tokens = tokenize_text(text)
print(tokens)
# Output: ['implementing', 'an', 'inverted', 'index', 'with', 'tidb', 'is', 'efficient']
Code Snippets for Index Construction
Once tokenized, the next step is to construct the inverted index and store it in TiDB. Here’s how you can map terms to documents and store the index:
from collections import defaultdict
import mysql.connector
def build_inverted_index(docs):
inverted_index = defaultdict(list)
for doc_id, text in enumerate(docs):
tokens = tokenize_text(text)
for token in tokens:
if doc_id not in inverted_index[token]:
inverted_index[token].append(doc_id)
return inverted_index
# Connect to TiDB
conn = mysql.connector.connect(
host='your_tidb_host',
user='your_username',
password='your_password',
database='your_database'
)
cursor = conn.cursor()
# Create a table for storing the inverted index
cursor.execute("""
CREATE TABLE IF NOT EXISTS inverted_index (
term VARCHAR(255),
document_ids JSON
)
""")
# Insert the inverted index into TiDB
index = build_inverted_index([
"Implementing an inverted index with TiDB is efficient.",
"TiDB supports large-scale data management."
])
for term, doc_ids in index.items():
cursor.execute("INSERT INTO inverted_index (term, document_ids) VALUES (%s, %s)", (term, json.dumps(doc_ids)))
conn.commit()
cursor.close()
conn.close()
By following these steps, you can efficiently implement an inverted index in Python, utilizing the scalability and robustness of PingCAP’s TiDB database. This setup not only enhances the speed of query processing but also ensures that your data is managed effectively, allowing you to focus on developing innovative applications.
Testing and Optimization
The journey of building an inverted index doesn’t end with its construction. To ensure it performs optimally, it’s crucial to test and refine the index. This section will guide you through practical approaches to testing and optimizing your inverted index, enhancing its speed and efficiency.
Testing the Inverted Index
Testing is a vital step in verifying that your inverted index functions as expected. By establishing robust test cases and employing effective debugging strategies, you can identify and resolve potential issues early on.
Test Cases
Creating comprehensive test cases is essential for validating the accuracy and reliability of your inverted index. Consider the following scenarios:
- Basic Retrieval: Ensure that the index correctly retrieves documents containing specific terms.
- Phrase Searches: Test the ability of the index to handle multi-word queries and return relevant documents.
- Edge Cases: Include tests for uncommon scenarios, such as queries with special characters or stop words.
- Scalability: Evaluate the index’s performance with varying data sizes to ensure it scales effectively.
By covering these aspects, you can confidently assess the functionality of your inverted index across different use cases.
Debugging Tips
When issues arise, having a set of debugging tips can streamline the troubleshooting process:
- Log Outputs: Implement logging to track the flow of data and identify where errors occur.
- Step-by-Step Execution: Break down the indexing process into smaller steps to isolate problematic areas.
- Data Inspection: Examine the contents of your index and input data to spot discrepancies.
- Peer Review: Collaborate with colleagues to gain fresh perspectives on potential solutions.
These strategies can help you efficiently pinpoint and resolve issues, ensuring your inverted index operates smoothly.
Optimizing Performance
Optimization is key to maximizing the efficiency of your inverted index. By focusing on speed and memory usage, you can enhance its overall performance.
Improving Speed
To boost the speed of your inverted index, consider the following techniques:
- Efficient Data Structures: Utilize data structures like hash tables or tries to accelerate lookups.
- Parallel Processing: Leverage multi-threading or distributed computing to process large datasets concurrently.
- Index Compression: Implement compression algorithms to reduce index size and improve access times.
These strategies can significantly enhance query performance, allowing your inverted index to retrieve information swiftly.
Reducing Memory Usage
Managing memory usage is crucial, especially when dealing with extensive datasets. Here are some approaches to consider:
- Sparse Indexing: Focus on indexing only the most relevant terms to minimize memory consumption.
- Data Pruning: Regularly clean and update the index to remove obsolete entries.
- Storage Solutions: Explore efficient storage options, such as PingCAP’s TiDB database, which supports scalable and high-performance data management.
By optimizing memory usage, you can ensure your inverted index remains responsive and capable of handling large-scale data efficiently.
In conclusion, testing and optimization are integral to maintaining a high-performing inverted index. By implementing these strategies, you can enhance the speed, accuracy, and scalability of your index, ensuring it meets the demands of modern information retrieval systems.
Building an inverted index in Python is a rewarding endeavor that enhances your ability to manage and retrieve information efficiently. By following the structured process outlined, you can create a robust data structure that significantly improves query performance. The benefits of using an inverted index are manifold: it allows for fast and efficient query processing, facilitates full-text searches, and is indispensable in search engines and database management systems like PingCAP’s TiDB database. However, it’s essential to recognize its limitations, such as increased storage requirements. We encourage you to explore further optimizations and experiment with different strategies to fully harness the potential of inverted indexes in your projects.