Go Modules are a game-changer in the world of Go programming, offering a modern approach to dependency management that enhances efficiency and reliability. With 87% of Go developers utilizing modules, it’s clear that this feature is integral to the language’s ecosystem. Designed with beginners in mind, this guide will walk you through the essentials of using a golang module. Whether you’re building websites, web services, or exploring new projects, understanding Go Modules is crucial for seamless development. Dive in and discover how these tools can simplify your coding journey and elevate your projects.
Getting Started with Go Modules
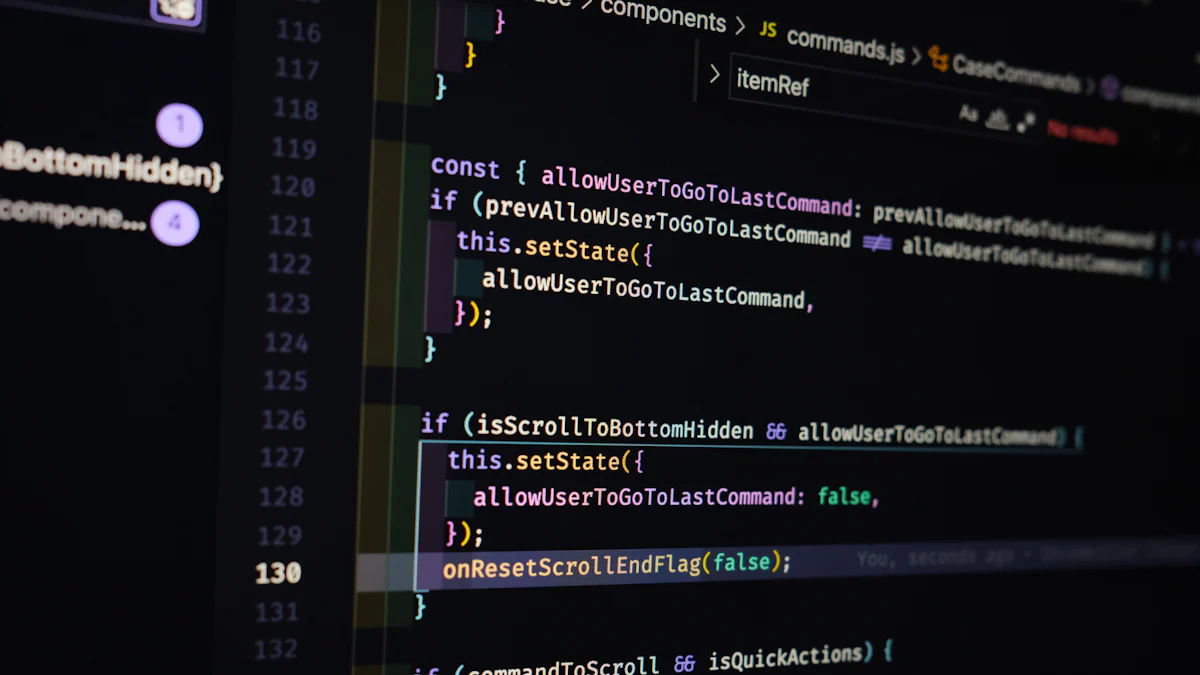
Embarking on your journey with Go Modules is a pivotal step in mastering Go programming. This section will guide you through the essentials, ensuring you have a solid foundation to build upon.
What are Go Modules?
Definition and Purpose
Go Modules are the building blocks of modern Go projects. Introduced in Go 1.11, they provide a structured way to manage dependencies and versioning in Go applications. A module is essentially a collection of Go packages stored in a directory with a go.mod
file at its root. This file defines the module’s path and its dependencies, allowing for seamless integration and management of external packages.
The primary purpose of Go Modules is to simplify dependency management. By using modules, developers can easily specify which versions of external libraries their projects depend on, ensuring consistency across different environments and builds.
Benefits of Using Go Modules
The adoption of Go Modules brings several benefits:
- Version Control: Modules allow precise control over which versions of dependencies are used, reducing compatibility issues and unexpected behavior.
- Dependency Management: With the
go.mod
file, tracking and updating dependencies becomes straightforward, making it easier to maintain large projects. - No GOPATH Restrictions: Unlike previous versions, Go Modules do not require projects to be located within the
GOPATH
, offering more flexibility in organizing code. - Community Support: As highlighted by Bartlomiej, the majority of Go users have embraced modules, leading to innovations such as advanced security features and reliable tool installations.
Setting Up Your First Go Module
Getting started with your first Go Module is a straightforward process. Here’s how you can set up your project:
Initializing a Module
Create a New Directory: Begin by creating a directory for your project. This will serve as the root for your module.
mkdir my-go-project
cd my-go-projectInitialize the Module: Use the
go mod init
command to create a new module. Replacemy-go-project
with your desired module name.go mod init my-go-project
This command generates a go.mod
file, which is crucial for managing your project’s dependencies.
Understanding go.mod File
The go.mod
file is the heart of a Go Module. It contains essential information about your module, including:
- Module Path: The unique identifier for your module, typically a URL or a local path.
- Go Version: Specifies the version of Go used to build the module.
- Dependencies: Lists all external packages required by your module, along with their versions.
Here’s a simple example of a go.mod
file:
module my-go-project
go 1.16
require (
github.com/go-sql-driver/mysql v1.5.0
)
In this example, the module depends on the mysql
driver, specifying the exact version needed. This ensures that anyone building your project will use the same library version, maintaining consistency.
By following these steps, you’ll have a fully initialized Go Module, ready to support your development efforts. For more detailed guidance, consider exploring resources like the Go Modules Reference or Francesc Campoy’s insightful video series on Go modules.
Managing Dependencies in Golang Module
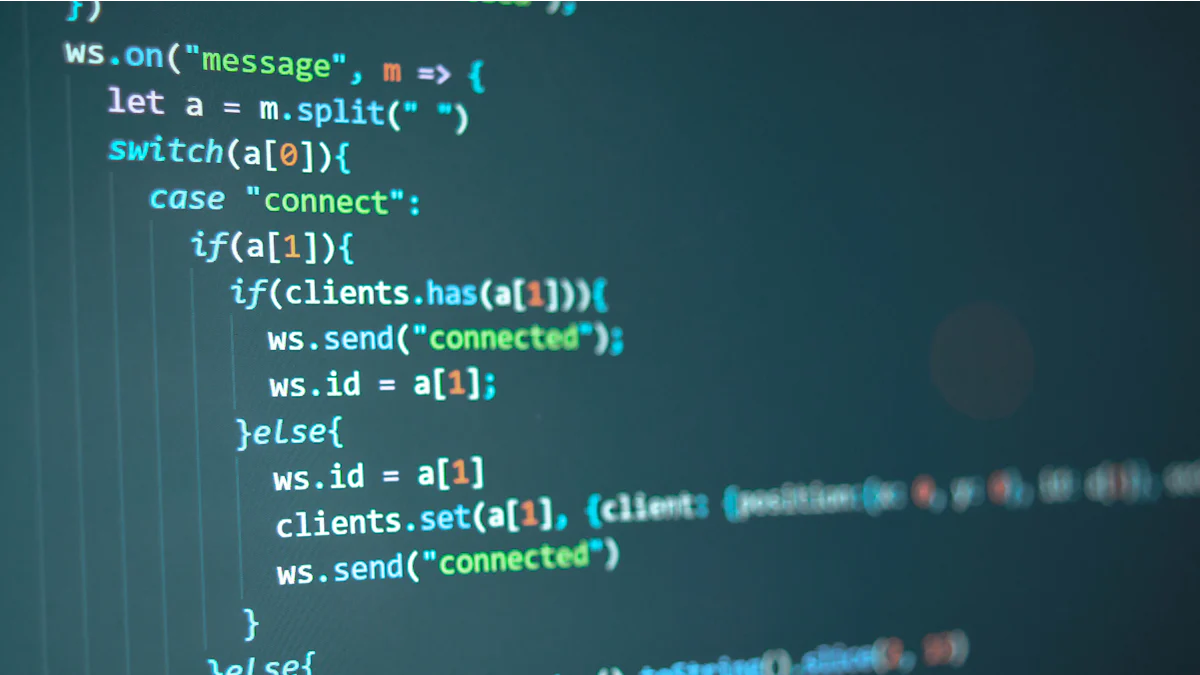
Navigating the world of dependencies in a golang module can seem daunting at first, but with Go Modules, the process becomes both intuitive and efficient. This section will guide you through adding, upgrading, and removing dependencies, ensuring your projects remain robust and up-to-date.
Adding Dependencies
When working with a golang module, adding dependencies is a straightforward task that enhances your project’s functionality by incorporating external packages.
Using go get Command
The go get
command is your primary tool for fetching new dependencies. It downloads the specified package and updates your go.mod
file automatically. Here’s how you can use it:
go get github.com/some/package
This command fetches the latest version of the package, making it available for use in your project. The seamless integration of go get
with Go Modules simplifies the process, allowing you to focus on development rather than dependency management.
Specifying Versions
Version control is a critical aspect of managing dependencies in a golang module. By specifying versions, you ensure that your project uses the exact library versions you intend, avoiding unexpected behavior due to changes in newer releases. To specify a version, simply append it to the package path:
go get github.com/some/package@v1.2.3
This command fetches version v1.2.3
of the package, updating your go.mod
file accordingly. Such precision in versioning is one of the key benefits of using Go Modules, providing stability and predictability in your projects.
Upgrading Dependencies
Keeping your dependencies up-to-date is essential for leveraging the latest features and security patches. Go Modules make this process efficient and manageable.
Checking for Updates
Before upgrading, it’s wise to check for available updates. You can do this by running:
go list -u -m all
This command lists all modules in your project, highlighting those with newer versions available. Regularly checking for updates ensures your project remains current and secure.
Updating to a New Version
Once you’ve identified outdated dependencies, upgrading them is simple. Use the go get
command with the desired version:
go get github.com/some/package@latest
This command updates the package to its latest version, reflecting the change in your go.mod
file. By maintaining updated dependencies, you enhance your project’s performance and security.
Removing Dependencies
Over time, some dependencies may become obsolete or unused. Efficiently managing these is crucial for keeping your golang module lean and efficient.
Identifying Unused Dependencies
To identify unused dependencies, Go provides the go mod tidy
command. This tool cleans up your go.mod
and go.sum
files by removing unnecessary entries:
go mod tidy
Running this command helps streamline your project, ensuring only essential dependencies are retained.
Cleaning Up go.mod
After identifying unused dependencies, it’s important to clean up your go.mod
file. The go mod tidy
command not only identifies but also removes these dependencies, keeping your project organized and efficient.
Advanced Module Management with TiDB
As you delve deeper into Go Modules, you’ll encounter scenarios that require advanced management techniques, especially when working with sophisticated databases like TiDB. This section will guide you through handling version conflicts and working with private modules, ensuring your projects remain robust and efficient.
Handling Version Conflicts
Version conflicts can be a common challenge when managing dependencies in Go Modules. Understanding how to navigate these conflicts is crucial for maintaining a stable development environment.
Understanding Semantic Versioning
Semantic Versioning (SemVer) is a versioning scheme that provides a clear and predictable way to manage changes in software packages. It uses a three-part version number: MAJOR.MINOR.PATCH
. Here’s what each part signifies:
- MAJOR: Increments when there are incompatible API changes.
- MINOR: Increments when functionality is added in a backward-compatible manner.
- PATCH: Increments when backward-compatible bug fixes are made.
By adhering to SemVer, you can better anticipate the impact of updates on your project. For instance, updating a dependency from version 1.2.3
to 1.3.0
should not break your code, whereas an update to 2.0.0
might.
Resolving Conflicts
When you encounter version conflicts, it’s essential to resolve them efficiently to maintain project stability. Here are some strategies:
Review Dependency Graph: Use the
go mod graph
command to visualize your project’s dependency tree. This helps identify conflicting versions.go mod graph
Update Dependencies: If a conflict arises due to outdated dependencies, consider updating them to compatible versions using the
go get
command.go get github.com/some/package@latest
Pin Specific Versions: In cases where newer versions introduce breaking changes, pinning dependencies to specific versions in your
go.mod
file can prevent unexpected issues.
By understanding and applying these techniques, you can effectively manage version conflicts, ensuring your TiDB projects run smoothly.
Working with Private Modules
In some cases, you may need to work with private Go Modules that are not publicly accessible. Managing these modules requires additional configuration to ensure secure and seamless access.
Configuring Access
To access private modules, you must configure your environment to authenticate with the repository hosting the module. This often involves setting up SSH keys or using personal access tokens.
- SSH Keys: Generate an SSH key pair and add the public key to your repository’s access settings.
- Access Tokens: For repositories that support it, generate a personal access token and use it in place of a password when cloning or fetching modules.
Using GOPRIVATE Environment Variable
The GOPRIVATE
environment variable is a powerful tool for managing private modules. It tells the Go command which modules are private, preventing it from attempting to fetch them from public proxies.
To set the GOPRIVATE
variable, add the following line to your shell configuration file (e.g., .bashrc
, .zshrc
):
export GOPRIVATE=github.com/your-private-repo
Replace github.com/your-private-repo
with the domain of your private module. This configuration ensures that your Go environment respects the privacy of your modules, allowing you to work securely and efficiently.
By mastering these advanced module management techniques, you’ll be well-equipped to handle complex dependency scenarios, especially when integrating with powerful databases like TiDB. This knowledge not only enhances your current projects but also prepares you for future challenges in Go development.
In this journey through Go Modules, we’ve unraveled the essentials of setting up and managing dependencies in your Go projects. From initializing your first module to mastering advanced techniques with the TiDB database, you’ve gained a solid foundation. Now, it’s time to put this knowledge into practice. Experiment, explore, and refine your skills. We invite you to share your experiences and insights—your feedback is invaluable. Let’s continue to grow and innovate together in the dynamic world of Go programming!