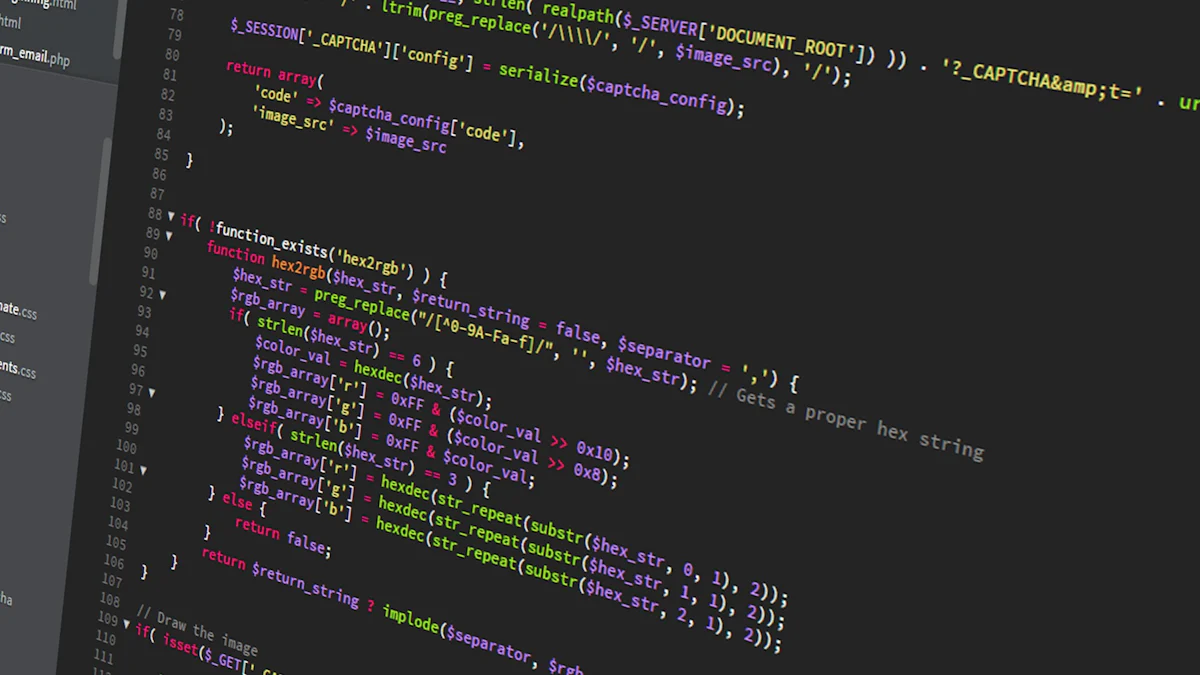
In the world of JavaScript development, efficiently performing a js check if key exists within an object is a fundamental skill. This task is not only about ensuring your code functions correctly but also about optimizing performance and reliability. Various methods, such as the in
operator and hasOwnProperty()
method, are available to achieve this, each with its own advantages and limitations. Choosing the right approach is crucial, especially when dealing with large objects, as it can significantly impact your application’s efficiency. Mastery of these techniques enhances both the robustness and speed of your JavaScript applications.
Understanding JavaScript Objects
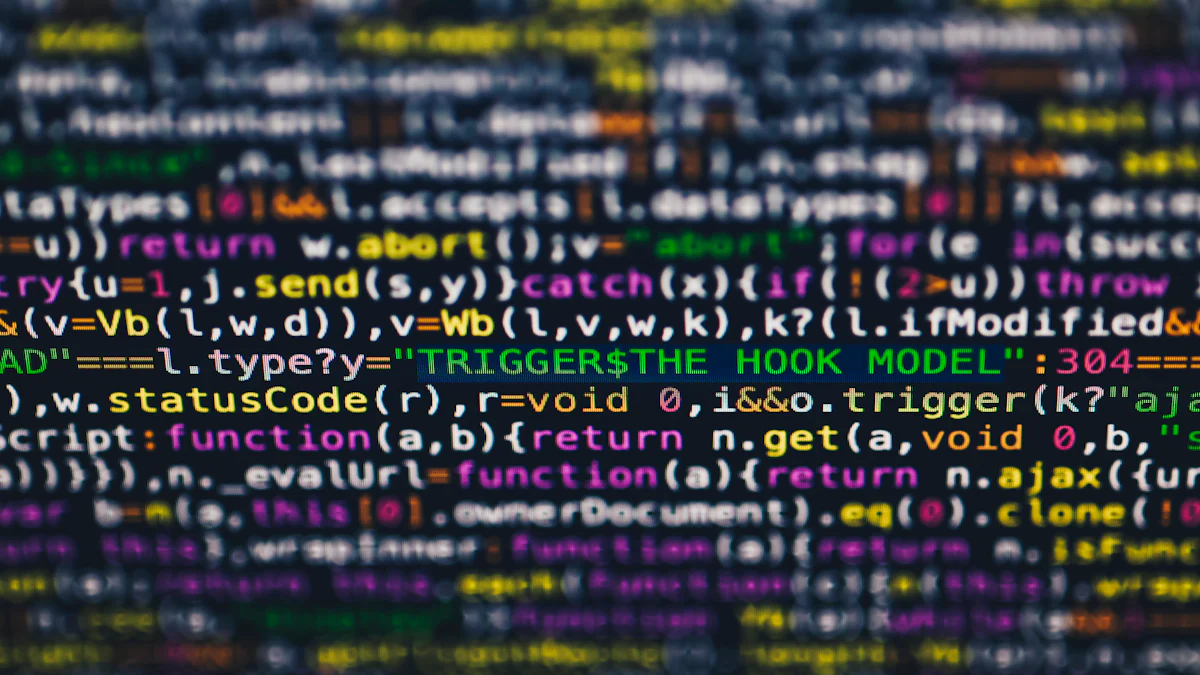
JavaScript objects are a cornerstone of the language, serving as the primary means for storing and organizing data. To effectively manipulate these objects, developers must understand their structure and the significance of key existence within them.
Basics of JavaScript Objects
Definition and Structure
In JavaScript, an object is a collection of key-value pairs where each key is a string (or Symbol) that maps to a value. This value can be anything from a primitive data type like a number or string to more complex structures like arrays or other objects. The syntax for creating an object is straightforward:
let person = {
name: "Alice",
age: 30,
occupation: "Engineer"
};
Here, name
, age
, and occupation
are keys, and “Alice”, 30
, and “Engineer” are their respective values. This flexibility makes objects incredibly versatile for various programming tasks.
Common Use Cases
JavaScript objects are used in numerous scenarios, such as:
- Data Storage: Objects can store related data and functions, making them ideal for modeling real-world entities.
- Configuration Settings: They often hold configuration settings for applications, allowing easy access and modification.
- APIs and JSON: Objects are fundamental to working with APIs and JSON, as they naturally map to JSON’s structure.
Understanding these use cases helps developers leverage objects effectively in their projects.
Importance of Key Existence
Impact on Code Functionality
Checking if a key exists in an object is crucial for ensuring code functionality. For example, when accessing an object’s property, verifying its existence prevents runtime errors and ensures the application behaves as expected. Using methods like the in
operator or hasOwnProperty()
method allows developers to perform a reliable js check if key exists:
if ('name' in person) {
console.log("Name exists!");
}
if (person.hasOwnProperty('age')) {
console.log("Age is a direct property!");
}
These methods help maintain code robustness by confirming whether a key is present before proceeding with operations that depend on it.
Performance Considerations
Performance is another critical factor when checking key existence. The in
operator checks both the object and its prototype chain, which can be beneficial or detrimental depending on the context. In contrast, hasOwnProperty()
only checks the object’s own properties, offering a more precise approach when inherited properties are irrelevant.
In terms of efficiency, the in
operator is generally faster due to its simplicity, but its broader scope may lead to unexpected results if not used carefully. Therefore, understanding the nuances between these methods is essential for optimizing performance in large-scale applications.
By mastering these concepts, developers can write more efficient and error-free JavaScript code, enhancing the overall quality of their applications.
Methods for Checking Key Existence in JavaScript

When working with JavaScript objects, it’s crucial to know how to efficiently perform a js check if key exists. This ensures your code runs smoothly and avoids unnecessary errors. Let’s explore some of the most effective methods for this task.
Using the ‘in’ Operator
Syntax and Usage
The in
operator is a straightforward way to determine if a key exists within an object or its prototype chain. Its syntax is simple:
if ('key' in object) {
// Key exists
}
This method checks for the presence of a specified property, returning true
if the property is found anywhere in the object, including its prototype chain.
Advantages and Limitations
Advantages:
- Simplicity: The
in
operator is easy to use and understand, making it a popular choice for quick checks. - Comprehensive: It checks both the object’s own properties and inherited properties, which can be useful in certain scenarios.
Limitations:
- Prototype Chain: Because it checks the entire prototype chain, it might return
true
for keys that are not directly part of the object, potentially leading to unexpected results.
Using ‘hasOwnProperty()’ Method
Syntax and Usage
The hasOwnProperty()
method is another reliable way to perform a js check if key exists, focusing solely on the object’s own properties:
if (object.hasOwnProperty('key')) {
// Key is a direct property
}
This method ensures that only the object’s own properties are checked, excluding any inherited ones.
Advantages and Limitations
Advantages:
- Precision: It provides a precise check by focusing only on the object’s direct properties, making it ideal when inherited properties should be ignored.
- Reliability: Offers a more controlled approach, reducing the risk of false positives from inherited properties.
Limitations:
- Slightly Slower: Although very close in performance to the
in
operator, it may be marginally slower due to its specificity.
Comparing ‘in’ and ‘hasOwnProperty()’
Key Differences
Understanding the differences between these two methods is essential for choosing the right one for your needs:
- Scope: The
in
operator checks both own and inherited properties, whilehasOwnProperty()
checks only own properties. - Performance: The
in
operator is generally faster, buthasOwnProperty()
offers more precision.
When to Use Each Method
- Use
in
: When you need to check for a property’s existence across the entire prototype chain, such as when dealing with objects that rely on inherited properties. - Use
hasOwnProperty()
: When you want to ensure the property is a direct member of the object, avoiding potential pitfalls from inherited properties.
By mastering these methods, developers can efficiently perform a js check if key exists, ensuring robust and optimized JavaScript applications. Whether you choose the in
operator for its speed or hasOwnProperty()
for its precision, understanding their nuances will enhance your coding practices.
Advanced Techniques for Efficient Key Checking
In the realm of JavaScript development, mastering advanced techniques for efficiently checking if a key exists in an object can significantly enhance your code’s performance and reliability. Let’s delve into some sophisticated methods that go beyond the basics.
Using ‘Object.keys()’ and ‘includes()’
Syntax and Usage
The Object.keys()
method is a powerful tool in JavaScript that returns an array of a given object’s own enumerable property names. By leveraging this array, you can utilize the includes()
method to determine if a specific key exists within the object. Here’s how you can implement this approach:
const person = {
name: "Alice",
age: 30,
occupation: "Engineer"
};
if (Object.keys(person).includes('name')) {
console.log("Name key exists!");
}
This technique is particularly useful when you need to perform a js check if key exists without traversing the prototype chain, ensuring that only the object’s own properties are considered.
Advantages and Limitations
Advantages:
- Precision: Similar to
hasOwnProperty()
, this method focuses solely on the object’s own properties, providing a precise check. - Flexibility: The use of
includes()
allows for easy integration with other array methods, offering greater flexibility in handling complex scenarios.
Limitations:
- Performance Overhead: Generating an array of keys and then checking for inclusion introduces additional overhead, making it less efficient than direct property checks like the
in
operator orhasOwnProperty()
method. - Not Suitable for Large Objects: For objects with a large number of properties, this method may not be the most performant choice due to its O(n) complexity.
Performance Optimization Tips
When performing a js check if key exists, optimizing for performance is crucial, especially in applications where speed and efficiency are paramount.
Best Practices
- Choose the Right Method: Select the method that best suits your needs. Use the
in
operator for a quick, broad check across the prototype chain, andhasOwnProperty()
for a precise check of direct properties. - Minimize Overhead: Avoid unnecessary operations like creating arrays of keys when simpler methods suffice.
- Profile Your Code: Regularly profile your code to identify bottlenecks and optimize key-checking operations accordingly.
Common Pitfalls to Avoid
- Ignoring Prototype Chain: Be aware of the implications of checking inherited properties. The
in
operator includes these, which might lead to unexpected results if not handled properly. - Overusing Array Methods: While methods like
Object.keys()
andincludes()
offer flexibility, they can introduce inefficiencies in performance-critical sections of your code. - Assuming Key Existence Guarantees Value: Remember that a key’s existence doesn’t guarantee a meaningful value. Always validate the value after confirming the key’s presence.
By incorporating these advanced techniques and optimization tips, developers can perform a js check if key exists more effectively, ensuring their JavaScript applications are both robust and high-performing. Understanding the nuances of each method will empower you to make informed decisions, enhancing your coding practices and application efficiency.
PingCAP’s Approach to Efficient Key Checking
In the ever-evolving landscape of JavaScript development, efficiently checking if a key exists in an object is paramount. PingCAP, with its innovative TiDB database, offers a unique approach to this challenge, ensuring both performance and reliability.
Leveraging TiDB for JavaScript Key Checking
Integration with JavaScript
PingCAP’s TiDB database seamlessly integrates with JavaScript environments, providing developers with advanced capabilities for managing data. By leveraging TiDB, developers can perform a js check if key exists with enhanced precision and speed. The integration process is straightforward, allowing JavaScript applications to communicate with TiDB through standard SQL queries. This compatibility ensures that developers can utilize TiDB’s powerful features without disrupting their existing workflows.
The integration supports various JavaScript frameworks and libraries, making it versatile for different project requirements. Whether you’re building a web application or a complex data-driven system, TiDB’s integration capabilities ensure that your JavaScript environment remains efficient and responsive.
Benefits of Using TiDB
TiDB offers several compelling benefits for developers looking to optimize their JavaScript applications:
-
Scalability: TiDB’s distributed architecture allows it to handle large volumes of data effortlessly. This scalability ensures that key existence checks remain fast and efficient, even as your data grows.
-
Consistency and Reliability: With strong consistency guarantees, TiDB ensures that your data operations, including key existence checks, are reliable and accurate. This consistency is crucial for applications that require real-time data processing and decision-making.
-
Performance Optimization: TiDB is designed for high-performance operations, reducing latency and improving the speed of key existence checks. This optimization is particularly beneficial for applications with stringent performance requirements.
-
Flexibility: TiDB’s compatibility with MySQL means that developers can easily transition their existing applications to leverage TiDB’s advanced features. This flexibility allows for seamless adoption without the need for extensive code rewrites.
By incorporating TiDB into your JavaScript projects, you can enhance the efficiency of performing a js check if key exists, ensuring that your applications are both robust and high-performing. PingCAP’s commitment to innovation and customer satisfaction makes TiDB an ideal choice for developers seeking cutting-edge solutions for their database needs.
In this guide, we’ve explored various methods for checking key existence in JavaScript objects, such as the in
operator and hasOwnProperty()
method. Each approach offers unique benefits and limitations, making it crucial to select the right one based on your project’s needs. By understanding these techniques, you can enhance both the efficiency and reliability of your code. We encourage you to apply these strategies in your JavaScript projects, ensuring robust and high-performing applications. Remember, choosing the appropriate method is key to optimizing your development process.