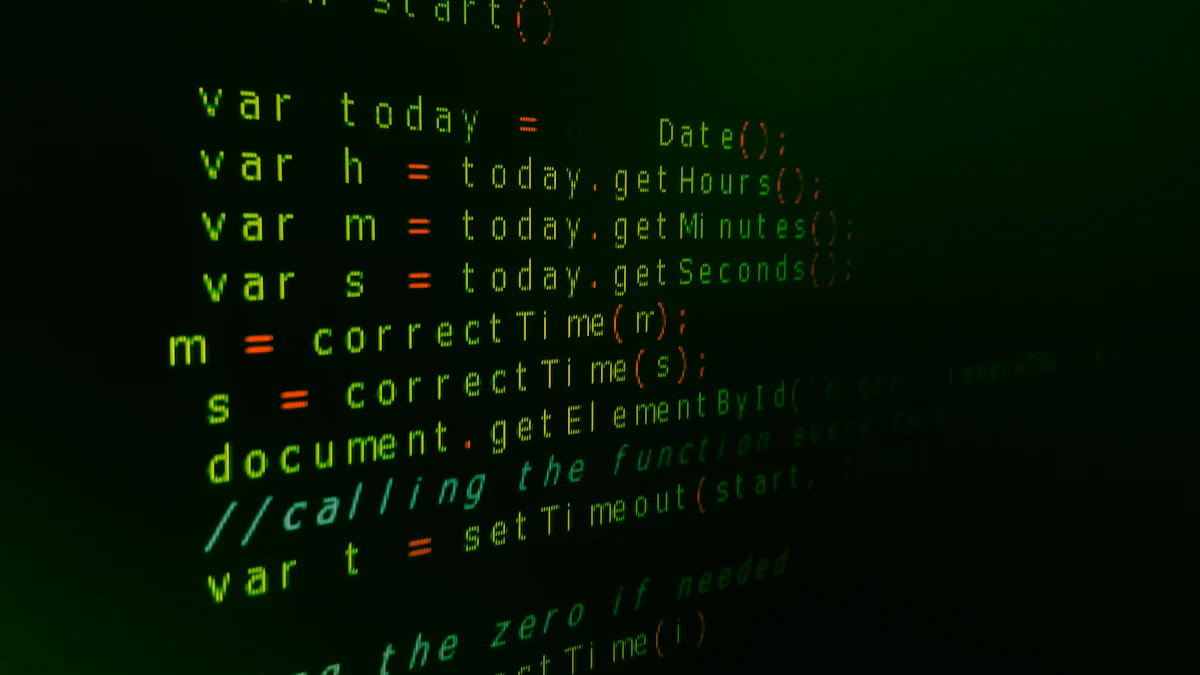
Structured Query Language (SQL) is the backbone of database management, empowering professionals to organize, manipulate, and retrieve data efficiently. Among its many features, user-defined functions (UDFs) stand out for their ability to extend SQL’s capabilities. UDFs allow developers to encapsulate frequently used logic into reusable modules, simplifying complex queries and enhancing code readability. For database professionals, mastering user defined functions in SQL is crucial, as it not only promotes code reusability but also significantly optimizes application performance by moving operations from the application layer to the database layer.
Understanding User-Defined Functions in SQL
What are User-Defined Functions in SQL?
Definition and Purpose
User-defined functions (UDFs) in SQL are custom functions created by users to perform specific tasks within a database. Unlike built-in functions, which come pre-packaged with the SQL system, UDFs allow developers to extend the capabilities of SQL by defining their own logic. This flexibility is invaluable for encapsulating frequently used operations into reusable modules, thereby streamlining complex queries and enhancing code maintainability.
Differences between UDFs and Built-in Functions
While both user-defined functions in SQL and built-in functions serve to perform operations on data, there are key differences between them:
- Creation and Customization: UDFs are crafted by users to meet specific needs, whereas built-in functions are predefined and cannot be altered.
- Flexibility: UDFs provide the ability to implement custom logic that may not be covered by built-in functions.
- Portability: Built-in functions are specific to each SQL system, while UDFs can be designed to work across different SQL platforms like SQL Server, PostgreSQL, and TiDB database.
Types of User-Defined Functions in SQL
Understanding the different types of user-defined functions in SQL is crucial for leveraging their full potential. There are primarily four types:
Scalar Functions
Scalar functions return a single value based on the input parameters. They are useful for computations that need to be applied to individual rows. For example, a scalar function could be used to calculate the age of a person from their date of birth.
CREATE FUNCTION CalculateAge (@BirthDate DATE)
RETURNS INT
AS
BEGIN
RETURN DATEDIFF(YEAR, @BirthDate, GETDATE())
END
Table-Valued Functions
Table-valued functions (TVFs) return a table as their result. These are particularly useful when you need to return a set of rows that can be queried further. TVFs can be either inline or multi-statement.
Inline Table-Valued Functions
Inline table-valued functions are similar to views but with parameters. They are defined using a single SELECT statement and are efficient because they do not require a separate execution plan.
CREATE FUNCTION GetEmployeesByDepartment (@DepartmentID INT)
RETURNS TABLE
AS
RETURN
(
SELECT EmployeeID, EmployeeName
FROM Employees
WHERE DepartmentID = @DepartmentID
)
Multi-Statement Table-Valued Functions
Multi-statement table-valued functions allow for more complex logic by using multiple statements to construct the result set. They are defined with a BEGIN...END
block and can include various SQL operations.
CREATE FUNCTION GetEmployeeDetails (@DepartmentID INT)
RETURNS @EmployeeDetails TABLE
(
EmployeeID INT,
EmployeeName NVARCHAR(100),
Position NVARCHAR(50)
)
AS
BEGIN
INSERT INTO @EmployeeDetails
SELECT EmployeeID, EmployeeName, Position
FROM Employees
WHERE DepartmentID = @DepartmentID
RETURN
END
By mastering these types of user-defined functions in SQL, developers can significantly enhance the functionality and performance of their databases. Whether it’s simplifying complex calculations or improving query performance, UDFs offer a powerful toolset for any database professional.
Creating User-Defined Functions in SQL
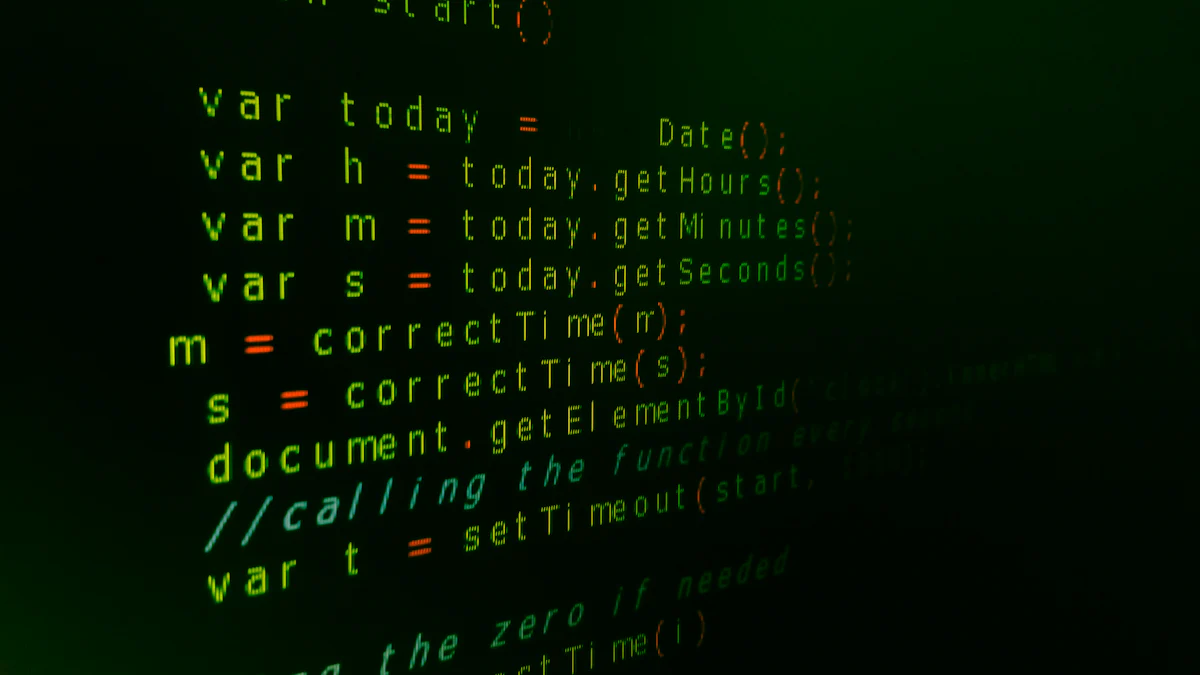
Creating user defined functions in SQL is a critical skill for database professionals. This section will guide you through the steps to create both scalar and table-valued functions, providing practical examples to illustrate each type.
Steps to Create a Scalar Function
Syntax and Structure
Scalar functions return a single value based on the input parameters. They are particularly useful for computations that need to be applied to individual rows. The basic syntax for creating a scalar function in SQL is as follows:
CREATE FUNCTION [schema_name.]function_name
(
@parameter_name data_type,
...
)
RETURNS data_type
AS
BEGIN
-- Function logic goes here
RETURN scalar_expression
END
In this syntax:
[schema_name.]function_name
specifies the name of the function, optionally prefixed by the schema name.@parameter_name data_type
defines the input parameters and their data types.RETURNS data_type
specifies the data type of the value returned by the function.- The
BEGIN...END
block contains the logic of the function, culminating in aRETURN
statement that provides the scalar result.
Example of a Scalar Function
Let’s consider a practical example where we create a scalar function to calculate the age of a person from their date of birth:
CREATE FUNCTION CalculateAge (@BirthDate DATE)
RETURNS INT
AS
BEGIN
RETURN DATEDIFF(YEAR, @BirthDate, GETDATE())
END
In this example:
- The function
CalculateAge
takes a single parameter@BirthDate
of typeDATE
. - It returns an
INT
representing the age. - The function uses the
DATEDIFF
function to calculate the difference in years between the birth date and the current date.
Steps to Create a Table-Valued Function
Syntax and Structure
Table-valued functions (TVFs) return a table as their result, making them ideal for scenarios where you need to return a set of rows. There are two main types of TVFs: inline and multi-statement.
Inline Table-Valued Functions
Inline table-valued functions are defined using a single SELECT
statement. The syntax is straightforward:
CREATE FUNCTION [schema_name.]function_name
(
@parameter_name data_type,
...
)
RETURNS TABLE
AS
RETURN
(
SELECT column_list
FROM table_name
WHERE condition
)
Multi-Statement Table-Valued Functions
Multi-statement TVFs allow for more complex logic by using multiple statements to construct the result set. The syntax includes a BEGIN...END
block:
CREATE FUNCTION [schema_name.]function_name
(
@parameter_name data_type,
...
)
RETURNS @table_variable TABLE
(
column_definition,
...
)
AS
BEGIN
-- Function logic goes here
RETURN
END
Example of a Table-Valued Function
Here’s an example of an inline table-valued function that retrieves employees by department:
CREATE FUNCTION GetEmployeesByDepartment (@DepartmentID INT)
RETURNS TABLE
AS
RETURN
(
SELECT EmployeeID, EmployeeName
FROM Employees
WHERE DepartmentID = @DepartmentID
)
In this example:
- The function
GetEmployeesByDepartment
takes a single parameter@DepartmentID
of typeINT
. - It returns a table with columns
EmployeeID
andEmployeeName
for employees in the specified department.
Multi-Statement Table-Valued Function
Now, let’s look at a multi-statement table-valued function that provides detailed employee information:
CREATE FUNCTION GetEmployeeDetails (@DepartmentID INT)
RETURNS @EmployeeDetails TABLE
(
EmployeeID INT,
EmployeeName NVARCHAR(100),
Position NVARCHAR(50)
)
AS
BEGIN
INSERT INTO @EmployeeDetails
SELECT EmployeeID, EmployeeName, Position
FROM Employees
WHERE DepartmentID = @DepartmentID
RETURN
END
In this example:
- The function
GetEmployeeDetails
takes a single parameter@DepartmentID
of typeINT
. - It returns a table variable
@EmployeeDetails
with columnsEmployeeID
,EmployeeName
, andPosition
. - The function uses an
INSERT INTO
statement to populate the table variable with data from theEmployees
table.
By mastering the creation of user defined functions in SQL, you can encapsulate complex logic into reusable modules, significantly enhancing the functionality and performance of your databases. Whether you’re working with scalar or table-valued functions, these tools offer powerful ways to streamline your SQL queries and improve code maintainability.
Practical Applications of User-Defined Functions in SQL
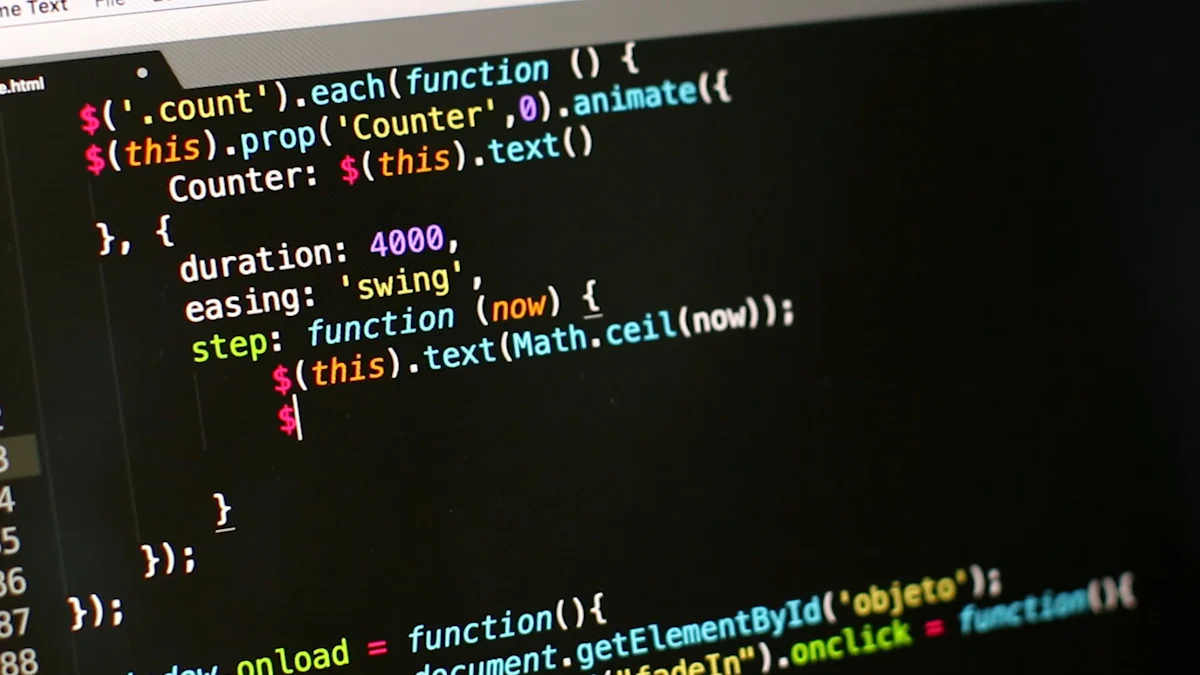
User defined functions in SQL are not just theoretical constructs; they have practical applications that can significantly enhance the performance, efficiency, and maintainability of your database operations. This section delves into how UDFs can be leveraged in real-world scenarios, particularly focusing on enhancing query performance with TiDB, simplifying complex calculations, and promoting reusability and maintenance.
Enhancing Query Performance with TiDB
One of the standout benefits of user defined functions in SQL is their ability to enhance query performance, especially when used with advanced databases like TiDB. By centralizing logic within the database layer, UDFs reduce the need for repetitive computations and streamline data retrieval processes.
Use Cases and Examples
Consider a scenario where you need to frequently calculate the total sales for different regions. Instead of writing complex queries every time, you can create a scalar function to encapsulate this logic:
CREATE FUNCTION CalculateTotalSales (@RegionID INT)
RETURNS DECIMAL(10, 2)
AS
BEGIN
RETURN (SELECT SUM(SalesAmount) FROM Sales WHERE RegionID = @RegionID)
END
By using this UDF, you can significantly reduce the complexity of your queries and improve performance. TiDB’s distributed architecture further optimizes these functions by caching and distributing the logic across nodes, ensuring faster execution times.
Addend Analytics highlights that “UDFs can improve performance by centralizing logic. Additionally, SQL Server can cache and optimize UDFs, leading to faster query execution.”
Simplifying Complex Calculations
User defined functions in SQL are invaluable for simplifying complex calculations that would otherwise make your queries cumbersome and difficult to read. By encapsulating these calculations within UDFs, you can make your SQL code more readable and maintainable.
Use Cases and Examples
Imagine you need to calculate the compounded annual growth rate (CAGR) for various financial metrics. Instead of embedding this complex formula in multiple queries, you can create a scalar function:
CREATE FUNCTION CalculateCAGR (@InitialValue DECIMAL(10, 2), @FinalValue DECIMAL(10, 2), @Years INT)
RETURNS DECIMAL(10, 2)
AS
BEGIN
RETURN POWER((@FinalValue / @InitialValue), (1.0 / @Years)) - 1
END
This function can then be reused across different queries, ensuring consistency and reducing the likelihood of errors. As Rakesh notes, “User-defined functions in SQL Server provide a convenient way to encapsulate logic and promote code reusability.”
Reusability and Maintenance
One of the most compelling reasons to use user defined functions in SQL is the reusability and ease of maintenance they offer. By encapsulating frequently used logic into UDFs, you can ensure that your codebase is more modular and easier to manage.
Benefits of Using UDFs in Large Projects
In large projects, maintaining a consistent codebase can be challenging. UDFs help by allowing you to define complex logic once and reuse it across multiple queries. This not only reduces the risk of errors but also makes it easier to update and maintain your code.
For example, if you have a complex discount calculation that needs to be applied across various parts of your application, you can encapsulate this logic in a UDF:
CREATE FUNCTION CalculateDiscount (@Price DECIMAL(10, 2), @DiscountRate DECIMAL(5, 2))
RETURNS DECIMAL(10, 2)
AS
BEGIN
RETURN @Price * (1 - @DiscountRate / 100)
END
By doing so, any changes to the discount logic need to be made in only one place, ensuring consistency and simplifying maintenance.
Baya Pavliashvili emphasizes that “UDFs provide certain advantages, such as encapsulating frequently used logic, which makes them ideal for large projects where maintainability is crucial.”
Best Practices for Using User-Defined Functions in SQL
User defined functions in SQL are powerful tools, but like any tool, they must be used wisely to maximize their benefits. This section will guide you through best practices to ensure your UDFs are efficient, secure, and maintainable.
Performance Considerations
While user defined functions in SQL can significantly enhance performance by centralizing logic within the database layer, it’s essential to be aware of potential pitfalls that could negate these benefits.
Avoiding Common Pitfalls
- Avoid Overuse: Not every piece of logic needs to be encapsulated in a UDF. Overusing UDFs can lead to performance bottlenecks, especially if they are called repeatedly within large queries.
- Optimize for Performance: Ensure that your UDFs are optimized for performance. For example, avoid using functions that perform complex calculations or access large datasets within a loop.
- Consider Inline Functions: Inline table-valued functions are generally more efficient than multi-statement table-valued functions because they do not require a separate execution plan.
- Minimize Side Effects: UDFs should be designed to minimize side effects. For instance, avoid modifying database state within a UDF, as this can lead to unexpected behavior and performance issues.
Pro Tip: Use the
SET NOCOUNT ON
statement within your UDFs to prevent the sending of DONE_IN_PROC messages to the client for each statement, which can improve performance.
Security Considerations
Security is paramount when working with user defined functions in SQL. Poorly designed UDFs can introduce vulnerabilities that compromise the integrity and security of your database.
Ensuring Safe and Secure UDFs
- Validate Input Parameters: Always validate input parameters to prevent SQL injection attacks. Ensure that parameters are of the expected type and within the expected range.
- Use Proper Permissions: Restrict permissions on UDFs to only those users who need them. Avoid granting unnecessary privileges that could be exploited.
- Avoid Dynamic SQL: Where possible, avoid using dynamic SQL within UDFs. If dynamic SQL is necessary, ensure that it is properly parameterized to prevent injection attacks.
- Review and Audit: Regularly review and audit your UDFs for security vulnerabilities. This includes checking for potential injection points, ensuring proper error handling, and validating all inputs.
Security Tip: Use the
EXECUTE AS
clause to specify the security context under which the UDF is executed, providing an additional layer of security.
Testing and Debugging
Thorough testing and debugging are crucial to ensure that your user defined functions in SQL perform as expected and are free from errors.
Techniques for Effective Testing
- Unit Testing: Develop unit tests for your UDFs to verify that they return the correct results for a variety of input scenarios. Use frameworks like tSQLt for SQL Server to automate these tests.
- Edge Cases: Test edge cases and invalid inputs to ensure that your UDFs handle them gracefully. This includes testing for null values, boundary conditions, and unexpected data types.
- Performance Testing: Conduct performance testing to ensure that your UDFs do not introduce significant overhead. Use tools like SQL Server Profiler or Extended Events to monitor performance.
- Debugging Tools: Utilize debugging tools and techniques to troubleshoot issues within your UDFs. This includes using print statements, temporary tables, and SQL Server Management Studio’s debugging features.
Testing Tip: Implement logging within your UDFs to capture execution details, which can be invaluable for debugging and performance tuning.
By adhering to these best practices, you can ensure that your user defined functions in SQL are efficient, secure, and maintainable. Whether you’re optimizing query performance, securing your database, or ensuring robust functionality through thorough testing, these guidelines will help you make the most of UDFs in your SQL environment.
User-defined functions (UDFs) in SQL are invaluable tools that enhance the flexibility, efficiency, and maintainability of database operations. By encapsulating frequently used logic into reusable modules, UDFs promote code reuse and improve query performance, especially when used with advanced databases like TiDB database. We encourage you to experiment with creating and using UDFs in your projects to fully leverage their potential. Mastering UDFs will not only streamline your SQL queries but also elevate your database management skills, ensuring robust and optimized systems.
See Also
Maximizing Query Performance with SQL EXPLAIN Tool
Exploring SQL Performance Optimization Techniques
Streamlining SQL Formatting Using TiDB Playground