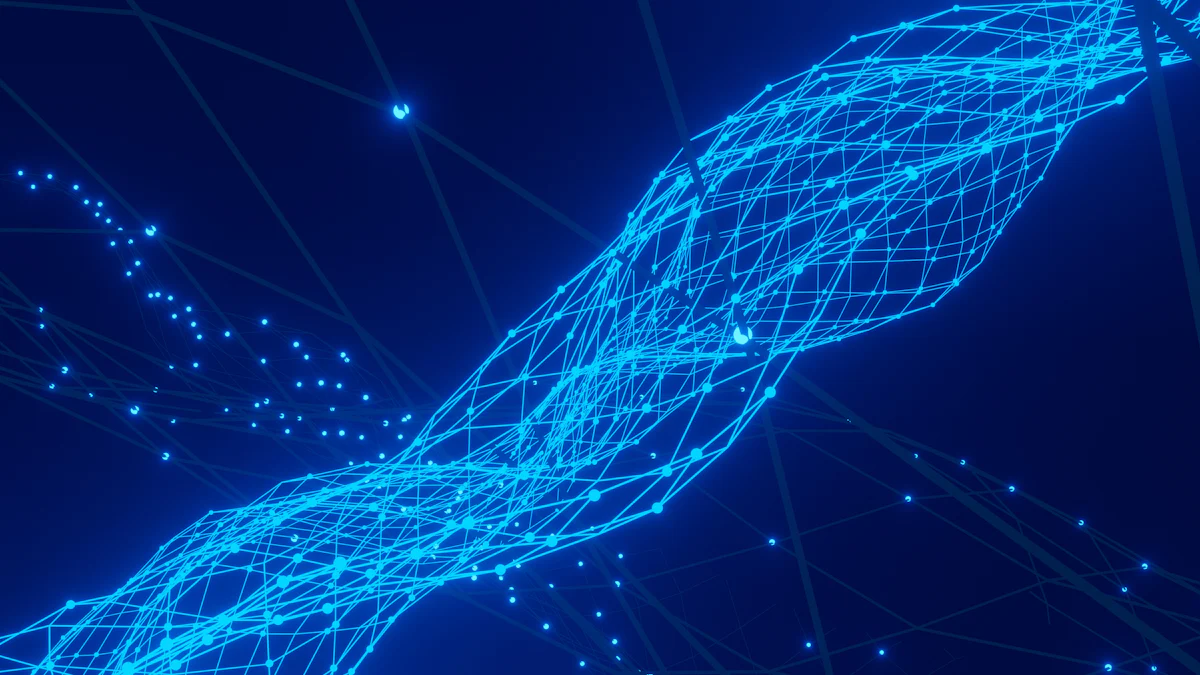
Semantic similarity is a cornerstone of Natural Language Processing (NLP), enabling machines to comprehend the meaning behind text. Its significance spans various applications, from enhancing search engine accuracy to improving chatbot interactions and refining recommendation systems. As the demand for precise tools grows, understanding and leveraging semantic similarity becomes crucial for developing intelligent, responsive systems.
Understanding Semantic Similarity
Definition and Importance
What is Semantic Similarity?
Semantic similarity is a metric used to evaluate the degree of similarity between two pieces of text based on their meaning or semantic content. Unlike simple lexical matching, which focuses on the exact words used, semantic similarity delves into the underlying context and concepts conveyed by the text. This approach allows for a more nuanced understanding of language, enabling machines to process and interpret human language more effectively.
In technical terms, semantic similarity can be quantified using various mathematical models and algorithms that analyze the relationships between words, phrases, or entire documents. These models often leverage word embeddings, vector space models, and deep learning techniques to capture the intricate patterns of meaning within the text.
Why is it Important in NLP?
Semantic similarity plays a pivotal role in Natural Language Processing (NLP) as it underpins many advanced language understanding tasks. By accurately assessing the semantic relationships between different pieces of text, NLP systems can perform a wide range of functions more effectively. Here are some key reasons why semantic similarity is crucial in NLP:
- Enhanced Comprehension: It enables machines to grasp the context and nuances of human language, leading to more accurate interpretations and responses.
- Improved User Experience: Applications like search engines and chatbots can provide more relevant and meaningful results, enhancing user satisfaction.
- Efficiency in Data Processing: It facilitates the efficient organization and retrieval of information, making it easier to manage large volumes of textual data.
Applications
Search Engines
Search engines rely heavily on semantic similarity to deliver precise and relevant search results. By understanding the intent behind a user’s query and comparing it with indexed documents, search engines can rank pages not just by keyword matching but by how well they match the user’s intended meaning. This leads to more accurate and useful search results, improving the overall user experience.
For example, if a user searches for “best places to visit in summer,” a search engine utilizing semantic similarity can prioritize articles about popular summer destinations over those that merely contain the keywords “places,” “visit,” and “summer.”
Chatbots
Chatbots and virtual assistants leverage semantic similarity to interpret user inputs and generate appropriate responses. By analyzing the semantic content of user queries, chatbots can understand the context and provide more accurate and relevant answers. This capability is essential for creating conversational agents that can engage in meaningful and coherent dialogues with users.
For instance, when a user asks a chatbot, “What’s the weather like today?” the chatbot can use semantic similarity to understand that the user is inquiring about the current weather conditions and respond accordingly with the latest weather update.
Recommendation Systems
Top Tools for Calculating Semantic Similarity
Tool 1: Word2Vec
Introduction to Word2Vec
Word2Vec is a widely used word embedding technique developed by Google. It transforms words into continuous vector values, capturing semantic relationships between them. The embeddings are learned through a two-layer neural network, which inadvertently captures linguistic contexts during the training process. This approach provides flexibility through two distinct model architectures: Continuous Bag of Words (CBOW) and continuous skip-gram.
Key Features
- Efficient Training: Utilizes a shallow neural network, making it computationally efficient.
- Two Model Architectures: CBOW predicts the target word from surrounding context words, while skip-gram does the opposite.
- Contextual Understanding: Captures both syntactic and semantic relationships between words.
Basic Implementation Steps
- Data Preparation: Collect and preprocess text data.
- Model Selection: Choose between CBOW and skip-gram architectures.
- Training: Use libraries like
gensim
in Python to train the model. - Embedding Extraction: Extract word vectors for further analysis or application.
from gensim.models import Word2Vec
# Example implementation
sentences = [["this", "is", "a", "sentence"], ["another", "sentence"]]
model = Word2Vec(sentences, vector_size=100, window=5, min_count=1, workers=4)
word_vector = model.wv['sentence']
Example Use Cases
- Text Classification: Enhance the performance of classifiers by using word embeddings as features.
- Machine Translation: Improve translation quality by understanding the semantic similarity between source and target languages.
- Information Retrieval: Enhance search engines by understanding the context of queries.
Advantages
- Efficiency: Fast training and inference.
- Flexibility: Applicable to various NLP tasks.
- Contextual Embeddings: Captures rich semantic information.
Limitations
- Fixed Vocabulary: Cannot handle out-of-vocabulary words.
- Lack of Polysemy Handling: Struggles with words having multiple meanings.
Tool 2: GloVe
Introduction to GloVe
GloVe (Global Vectors for Word Representation) is a powerful word embedding technique developed by Stanford. It captures semantic relationships between words by considering their co-occurrence probabilities within a corpus. The key to GloVe’s effectiveness lies in the construction of a word-context matrix and the subsequent factorization process.
Key Features
- Co-occurrence Matrix: Uses global word-word co-occurrence statistics.
- Efficient Factorization: Employs matrix factorization to generate word vectors.
- Semantic Richness: Captures both syntactic and semantic properties of words.
Basic Implementation Steps
- Corpus Collection: Gather a large text corpus.
- Co-occurrence Matrix Construction: Build a matrix of word co-occurrences.
- Matrix Factorization: Apply factorization techniques to obtain word vectors.
- Embedding Usage: Use the generated embeddings in downstream tasks.
import numpy as np
from glove import Glove
# Example implementation
corpus = [['this', 'is', 'a', 'sentence'], ['another', 'sentence']]
glove = Glove(no_components=100, learning_rate=0.05)
glove.fit(corpus, epochs=10, no_threads=4)
word_vector = glove.word_vectors[glove.dictionary['sentence']]
Example Use Cases
- Sentiment Analysis: Improve sentiment classification by leveraging semantic similarities.
- Named Entity Recognition: Enhance entity recognition by understanding word contexts.
- Document Clustering: Group similar documents based on their semantic content.
Advantages
- Global Context: Considers entire corpus for embedding generation.
- Semantic Accuracy: Produces high-quality word vectors.
- Scalability: Efficient for large datasets.
Limitations
- Resource Intensive: Requires significant computational resources.
- Static Embeddings: Cannot adapt to new words or contexts.
Tool 3: BERT
Introduction to BERT
BERT (Bidirectional Encoder Representations from Transformers) is a groundbreaking model developed by Google. Unlike traditional models, BERT processes text bidirectionally, allowing it to capture context from both directions. This results in highly accurate semantic representations of text.
Key Features
- Bidirectional Context: Understands context from both left and right.
- Pre-trained Models: Leverages pre-trained models for various NLP tasks.
- Fine-tuning Capability: Can be fine-tuned for specific applications.
Basic Implementation Steps
- Pre-trained Model Selection: Choose a pre-trained BERT model from libraries like
transformers
. - Tokenization: Tokenize input text using BERT’s tokenizer.
- Model Inference: Use the model to generate embeddings.
- Fine-tuning: Fine-tune the model for specific tasks if needed.
from transformers import BertTokenizer, BertModel
# Example implementation
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertModel.from_pretrained('bert-base-uncased')
inputs = tokenizer("This is a sentence.", return_tensors='pt')
outputs = model(**inputs)
embeddings = outputs.last_hidden_state
Example Use Cases
- Question Answering: Enhance QA systems by understanding the context of questions and answers.
- Text Summarization: Generate concise summaries by capturing the essence of documents.
- Semantic Search: Improve search accuracy by understanding query semantics.
Advantages
- High Accuracy: Superior performance on various NLP benchmarks.
- Versatility: Applicable to a wide range of NLP tasks.
- Contextual Understanding: Captures deep semantic relationships.
Limitations
- Computationally Expensive: Requires significant resources for training and inference.
- Complexity: More complex to implement and fine-tune compared to simpler models.
Tool 4: FastText
Introduction to FastText
FastText, developed by Facebook‘s AI Research (FAIR) lab, is an extension of the Word2Vec model designed to address some of its limitations. Unlike traditional word embeddings, FastText represents each word as a bag of character n-grams. This allows it to generate embeddings for words that were not present in the training data, making it particularly useful for handling out-of-vocabulary words and morphologically rich languages.
Key Features
- Subword Information: Utilizes character n-grams to create word vectors, capturing morphological information.
- Efficient Training: Capable of training on large datasets quickly.
- Hierarchical Softmax: Uses a hierarchical softmax function to speed up the computation of probabilities.
- Pre-trained Models: Offers pre-trained models for various languages, facilitating quick deployment.
Basic Implementation Steps
- Install FastText: Use the
fasttext
library in Python. - Data Preparation: Preprocess text data by tokenizing and normalizing.
- Model Training: Train the model using the
train_unsupervised
function. - Embedding Extraction: Extract word vectors for downstream tasks.
import fasttext
# Example implementation
model = fasttext.train_unsupervised('data.txt', model='skipgram')
word_vector = model.get_word_vector('example')
Example Use Cases
- Text Classification: Enhance classification tasks by using robust word embeddings.
- Spell Correction: Improve spell correction systems by understanding word morphology.
- Language Modeling: Develop language models that can handle rare and compound words effectively.
Advantages
- Handling Out-of-Vocabulary Words: Generates embeddings for unseen words.
- Morphological Awareness: Captures subword information, beneficial for languages with rich morphology.
- Speed: Fast training and inference times.
Limitations
- Context Independence: Does not capture contextual information as effectively as models like BERT.
- Fixed Embeddings: Produces static word embeddings, limiting adaptability to new contexts.
Tool 5: Universal Sentence Encoder
Introduction to Universal Sentence Encoder
The Universal Sentence Encoder (USE), developed by Google, is designed to encode sentences into high-dimensional vectors that capture semantic similarity. Unlike word-level embeddings, USE focuses on sentence-level embeddings, making it ideal for tasks that require understanding the meaning of entire sentences or paragraphs.
Key Features
- Sentence-Level Embeddings: Encodes entire sentences, capturing their overall meaning.
- Pre-trained Models: Available in TensorFlow Hub, ready for immediate use.
- Versatility: Suitable for a wide range of NLP tasks, including semantic similarity, clustering, and classification.
Basic Implementation Steps
- Install TensorFlow Hub: Use the
tensorflow_hub
library in Python. - Load Pre-trained Model: Load the Universal Sentence Encoder from TensorFlow Hub.
- Encode Sentences: Use the model to generate sentence embeddings.
- Utilize Embeddings: Apply the embeddings in various NLP tasks.
import tensorflow_hub as hub
# Example implementation
embed = hub.load("https://tfhub.dev/google/universal-sentence-encoder/4")
sentences = ["This is a sentence.", "Another sentence."]
embeddings = embed(sentences)
Example Use Cases
- Semantic Search: Improve search engines by understanding the meaning of queries and documents.
- Text Clustering: Group similar texts based on their semantic content.
- Paraphrase Detection: Identify sentences that convey the same meaning using sentence embeddings.
Advantages
- Contextual Understanding: Captures the meaning of entire sentences.
- Ease of Use: Pre-trained models simplify deployment.
- Versatile Applications: Applicable to various NLP tasks requiring semantic similarity.
Limitations
- Resource Intensive: Requires significant computational resources for inference.
- Fixed Context: Cannot dynamically adapt to new contexts without retraining.
Tool 6: InferSent
Introduction to InferSent
InferSent, developed by Facebook, is a sentence embedding technique that leverages supervised learning to generate high-quality sentence representations. It is trained on the Stanford Natural Language Inference (SNLI) dataset, making it particularly effective for tasks that involve understanding the semantic similarity between sentences.
Key Features
- Supervised Learning: Trained on labeled data to capture nuanced semantic relationships.
- High-Quality Embeddings: Produces robust sentence embeddings that perform well on various benchmarks.
- Compatibility: Works well with other machine learning frameworks and models.
Basic Implementation Steps
- Install Required Libraries: Use libraries like
torch
andInferSent
. - Load Pre-trained Model: Download and load the pre-trained InferSent model.
- Encode Sentences: Generate sentence embeddings using the model.
- Apply Embeddings: Use the embeddings in downstream NLP tasks.
import torch
from models import InferSent
# Example implementation
params_model = {'bsize': 64, 'word_emb_dim': 300, 'enc_lstm_dim': 2048, 'pool_type': 'max', 'dpout_model': 0.0}
model = InferSent(params_model)
model.load_state_dict(torch.load('infersent.allnli.pickle'))
sentences = ["This is a sentence.", "Another sentence."]
embeddings = model.encode(sentences, bsize=128, tokenize=False)
Example Use Cases
- Semantic Textual Similarity: Measure the similarity between sentences for applications like plagiarism detection.
- Question Answering: Improve QA systems by understanding the semantic content of questions and answers.
- Text Summarization: Generate summaries by capturing the essence of documents.
Advantages
- High Performance: Produces state-of-the-art sentence embeddings.
- Supervised Training: Benefits from labeled data to capture detailed semantic relationships.
- Versatility: Suitable for various NLP tasks involving semantic similarity.
Limitations
- Training Data Dependency: Performance may vary depending on the quality and size of the training data.
- Resource Intensive: Requires substantial computational resources for training and inference.
Tool 7: ELMo
Introduction to ELMo
ELMo (Embeddings from Language Models) is a deep contextualized word representation model developed by the Allen Institute for AI. Unlike traditional word embeddings that assign a single vector to each word, ELMo generates word representations that are sensitive to the context in which they appear. This is achieved through a bidirectional LSTM (Long Short-Term Memory) network, which processes text in both forward and backward directions.
Key Features
- Contextualized Embeddings: Generates different embeddings for the same word depending on its context.
- Deep Learning: Utilizes a deep, bidirectional LSTM architecture.
- Pre-trained Models: Available for immediate use, reducing the need for extensive training.
Basic Implementation Steps
- Install Dependencies: Use libraries like
allennlp
andtorch
. - Load Pre-trained Model: Download and load the ELMo model.
- Tokenize Text: Prepare your text data for embedding generation.
- Generate Embeddings: Use the model to create contextualized word embeddings.
from allennlp.modules.elmo import Elmo, batch_to_ids
# Example implementation
options_file = "https://allennlp.s3.amazonaws.com/models/elmo/2x4096_512_2048cnn_2xhighway/elmo_2x4096_512_2048cnn_2xhighway_options.json"
weight_file = "https://allennlp.s3.amazonaws.com/models/elmo/2x4096_512_2048cnn_2xhighway/elmo_2x4096_512_2048cnn_2xhighway_weights.hdf5"
elmo = Elmo(options_file, weight_file, 2, dropout=0)
sentences = [['This', 'is', 'a', 'sentence'], ['Another', 'sentence']]
character_ids = batch_to_ids(sentences)
embeddings = elmo(character_ids)
Example Use Cases
- Named Entity Recognition: Improve entity recognition by leveraging context-aware embeddings.
- Sentiment Analysis: Enhance sentiment classification by understanding the nuanced meanings of words.
- Machine Translation: Improve translation quality by capturing the context of words and phrases.
Advantages
- Context Sensitivity: Captures the meaning of words based on their context.
- Versatility: Applicable to various NLP tasks requiring deep semantic understanding.
- Pre-trained Models: Reduces the need for extensive computational resources for training.
Limitations
- Resource Intensive: Requires significant computational power for inference.
- Complexity: More complex to implement compared to simpler models like Word2Vec.
Tool 8: RoBERTa
Introduction to RoBERTa
RoBERTa (Robustly optimized BERT approach) is an enhanced version of BERT, developed by Facebook AI. It builds on BERT’s architecture by optimizing the training process and increasing the amount of training data. RoBERTa achieves state-of-the-art performance on various NLP benchmarks by fine-tuning hyperparameters and removing certain training constraints.
Key Features
- Optimized Training: Utilizes a larger dataset and longer training times.
- Improved Performance: Outperforms BERT on several NLP tasks.
- Flexibility: Can be fine-tuned for specific applications.
Basic Implementation Steps
- Install Transformers Library: Use the
transformers
library by Hugging Face. - Load Pre-trained Model: Choose a pre-trained RoBERTa model.
- Tokenize Input Text: Tokenize the text using RoBERTa’s tokenizer.
- Generate Embeddings: Use the model to produce embeddings.
from transformers import RobertaTokenizer, RobertaModel
# Example implementation
tokenizer = RobertaTokenizer.from_pretrained('roberta-base')
model = RobertaModel.from_pretrained('roberta-base')
inputs = tokenizer("This is a sentence.", return_tensors='pt')
outputs = model(**inputs)
embeddings = outputs.last_hidden_state
Example Use Cases
- Text Classification: Enhance classification tasks with robust embeddings.
- Question Answering: Improve QA systems by understanding complex queries.
- Semantic Search: Enhance search engines by accurately interpreting user queries.
Advantages
- High Performance: Superior results on various NLP benchmarks.
- Robust Training: Benefits from extensive training optimizations.
- Versatility: Suitable for a wide range of NLP applications.
Limitations
- Computationally Expensive: Requires substantial resources for training and inference.
- Complexity: More challenging to implement and fine-tune compared to simpler models.
Tool 9: Sentence-BERT
Introduction to Sentence-BERT
Sentence-BERT (SBERT) is a modification of BERT designed specifically for generating sentence embeddings. Developed by UKP Lab, SBERT fine-tunes BERT using Siamese and triplet network structures, making it highly effective for tasks that require comparing sentence pairs, such as semantic similarity and paraphrase detection.
Key Features
- Sentence-Level Embeddings: Generates embeddings for entire sentences.
- Efficient Similarity Computation: Optimized for comparing sentence pairs.
- Pre-trained Models: Available for quick deployment.
Basic Implementation Steps
- Install Sentence-Transformers Library: Use the
sentence-transformers
library. - Load Pre-trained Model: Choose a pre-trained SBERT model.
- Encode Sentences: Generate sentence embeddings.
- Utilize Embeddings: Apply the embeddings in various NLP tasks.
from sentence_transformers import SentenceTransformer
# Example implementation
model = SentenceTransformer('paraphrase-MiniLM-L6-v2')
sentences = ["This is a sentence.", "Another sentence."]
embeddings = model.encode(sentences)
Example Use Cases
- Semantic Textual Similarity: Measure the similarity between sentences for applications like plagiarism detection.
- Paraphrase Identification: Detect sentences that convey the same meaning.
- Text Clustering: Group similar texts based on their semantic content.
Advantages
- High Accuracy: Produces reliable sentence embeddings.
- Efficiency: Optimized for fast similarity computation.
- Versatility: Suitable for a variety of NLP tasks involving sentence comparisons.
Limitations
- Resource Intensive: Requires significant computational resources for training and inference.
- Fixed Context: Cannot dynamically adapt to new contexts without retraining.
Tool 10: SimCSE
Introduction to SimCSE
SimCSE (Simple Contrastive Learning of Sentence Embeddings) is a state-of-the-art technique developed to generate high-quality sentence embeddings. Unlike traditional methods, SimCSE leverages contrastive learning, a powerful approach that trains models to distinguish between similar and dissimilar sentence pairs. This method enhances the model’s ability to capture nuanced semantic relationships, making it highly effective for various NLP tasks requiring semantic similarity.
Key Features
- Contrastive Learning: Utilizes contrastive learning to improve the quality of sentence embeddings.
- Pre-trained Models: Offers pre-trained models for quick deployment and fine-tuning.
- Versatility: Suitable for a wide range of applications, including semantic textual similarity and paraphrase detection.
- Efficiency: Optimized for both training and inference, ensuring fast and accurate results.
Basic Implementation Steps
- Install Dependencies: Use libraries like
transformers
andsentence-transformers
. - Load Pre-trained Model: Select a pre-trained SimCSE model from available repositories.
- Tokenize Sentences: Prepare your text data by tokenizing sentences.
- Generate Embeddings: Use the model to create sentence embeddings for downstream tasks.
from sentence_transformers import SentenceTransformer
# Example implementation
model = SentenceTransformer('princeton-nlp/sup-simcse-bert-base-uncased')
sentences = ["This is a sentence.", "Another sentence."]
embeddings = model.encode(sentences)
Example Use Cases
- Semantic Textual Similarity: Measure the similarity between sentences for applications like plagiarism detection and duplicate content identification.
- Paraphrase Detection: Identify sentences that convey the same meaning, enhancing tools for content generation and summarization.
- Text Clustering: Group similar texts based on their semantic content, useful for organizing large datasets.
Advantages
- High Accuracy: Produces reliable and accurate sentence embeddings, outperforming many traditional methods.
- Ease of Use: Pre-trained models simplify the deployment process, allowing for quick integration into existing systems.
- Scalability: Efficient training and inference make it suitable for large-scale applications.
Limitations
- Resource Intensive: Requires significant computational resources for both training and inference, which may be a constraint for some users.
- Dependency on Training Data: The quality of embeddings can vary depending on the training data used, necessitating careful selection and preprocessing of datasets.
PingCAP’s Contribution to Semantic Similarity
TiDB and Semantic Similarity
Advanced Vector Database Features
PingCAP’s TiDB database stands out with its advanced vector database features, which are specifically optimized for AI applications. These features enable the efficient handling of high-dimensional data, making it an ideal choice for tasks involving semantic similarity. With TiDB, users can store and manage vector representations of text, images, and other data types, facilitating complex queries and analyses.
One of the key advantages of TiDB is its support for Hybrid Transactional and Analytical Processing (HTAP) workloads. This allows users to perform real-time analytics on transactional data without compromising performance. By leveraging these capabilities, developers can build sophisticated NLP applications that require both high throughput and low latency.
Efficient Vector Indexing
Efficient vector indexing is crucial for performing fast and accurate similarity searches. TiDB incorporates state-of-the-art indexing techniques that significantly enhance the speed and precision of vector searches. This is particularly beneficial for applications such as recommendation systems, where quick retrieval of similar items is essential.
TiDB’s indexing capabilities are designed to handle large-scale datasets, ensuring that even as the volume of data grows, the performance remains consistent. The use of multi-dimensional indexes allows for efficient querying and retrieval of vectors, making it easier to find semantically similar items in vast datasets.
Semantic Searches
Semantic searches go beyond traditional keyword-based searches by understanding the meaning and context of the query. TiDB’s advanced vector database features enable semantic searches, allowing users to retrieve results that are more relevant and meaningful.
For instance, in a search engine application, TiDB can be used to compare the semantic similarity between user queries and indexed documents. This ensures that the search results are not just based on keyword matching but also on the underlying context and intent of the query. By integrating TiDB’s semantic search capabilities, developers can create more intuitive and user-friendly search experiences.
Integration with AI Frameworks
TiDB seamlessly integrates with various AI frameworks, making it easier for developers to incorporate advanced machine learning models into their applications. This integration supports the deployment of models that require high-performance vector storage and retrieval, such as those used for semantic similarity tasks.
For example, TiDB can be used in conjunction with Google’s Universal Sentence Encoder to store and manage sentence embeddings. These embeddings can then be efficiently queried to find semantically similar sentences, enhancing applications like chatbots and recommendation systems.
Moreover, TiDB’s compatibility with popular machine learning libraries such as TensorFlow and PyTorch allows for smooth integration of pre-trained models like InferSent and FastText. This flexibility enables developers to leverage the strengths of different models and frameworks, creating robust and scalable NLP solutions.
In conclusion, PingCAP’s TiDB database offers a comprehensive suite of features that significantly enhance the calculation and application of semantic similarity. From advanced vector indexing to seamless integration with AI frameworks, TiDB provides the tools necessary to build intelligent, responsive, and efficient NLP applications.
Semantic similarity is a fundamental aspect of NLP, enabling machines to understand and process human language more effectively. The tools discussed, from Word2Vec and GloVe to advanced models like BERT, RoBERTa, and SimCSE, each offer unique features and benefits. These tools enhance various applications, including search engines, chatbots, and recommendation systems.
We encourage you to explore these tools further, considering your specific needs and project requirements. Share your experiences or suggest other tools in the comments below. Your insights can help others navigate the evolving landscape of semantic similarity in NLP.
See Also
Enhancing Semantic Capabilities through Azure OpenAI and TiDB Vector Integration
TiDB Serverless: Unleashing Semantic Search Potential
Creating Semantic Cache Service using Jina AI Embedding and TiDB Vector