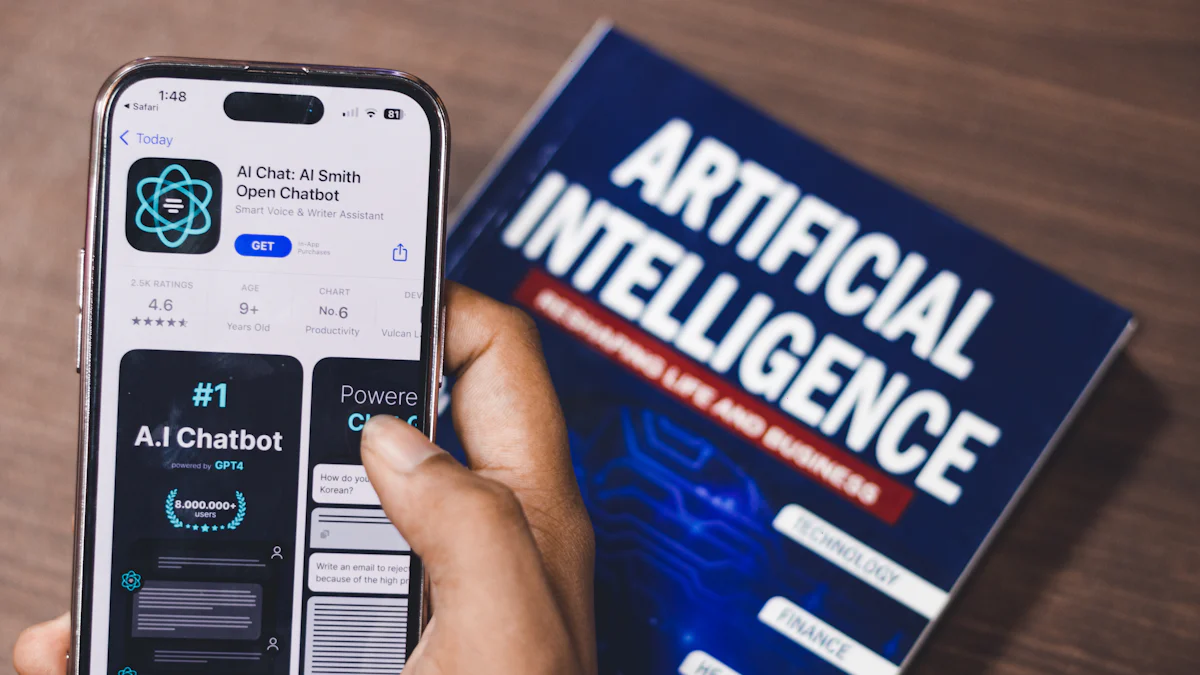
Intelligent chatbots have become indispensable in modern applications, with LangChain MySQL being a revolutionary framework that bridges the advanced natural language processing capabilities of OpenAI’s models with the robust data management of MySQL. By integrating LangChain MySQL, developers can create chatbots that not only understand and respond to user queries in natural language but also leverage powerful database functionalities for enhanced performance and scalability.
Getting Started with LangChain
Introduction to LangChain
What is LangChain?
LangChain is an innovative open-source library designed to simplify the development of applications that leverage Large Language Models (LLMs). By providing a robust framework, LangChain enables developers to create sophisticated AI-driven applications that can interact with external data sources such as personal documents or the internet. This capability is particularly useful for building intelligent chatbots that require real-time data processing and seamless integration with LLMs.
LangChain acts as a bridge between natural language processing (NLP) and databases, allowing users to interact with their databases using natural language. This eliminates the need for complex SQL queries, making data retrieval and manipulation more intuitive and accessible.
Key Features of LangChain
LangChain offers several key features that make it a powerful tool for developers:
- Standard Interface for LLMs: LangChain provides a unified interface to interact with a variety of LLMs, simplifying the process of integrating different models into your applications.
- Prompt Construction: It includes tools for constructing effective prompts, which are essential for guiding the behavior of LLMs.
- Conversational Memory: LangChain supports conversational memory, enabling chatbots to maintain context over multiple interactions.
- Intelligent Agents: The framework allows for the creation of intelligent agents that can perform complex tasks by chaining together multiple commands.
- Indexes: LangChain can create and manage indexes, facilitating efficient data retrieval.
- Chains: It enables the execution of a series of prompts to achieve specific outcomes, streamlining the development of complex workflows.
Setting Up LangChain
Installation Requirements
Before you can start using LangChain, ensure that your system meets the following requirements:
- Python 3.8 or higher
- A compatible LLM (e.g., OpenAI’s GPT-3)
- Access to a MySQL database
Additionally, you will need to install several Python packages, including langchain
, pymysql
, and sqlalchemy
.
Step-by-Step Installation Guide
Follow these steps to set up LangChain on your system:
-
Install Python Packages: Open your terminal and run the following commands to install the required packages:
pip install langchain pymysql sqlalchemy
-
Set Up Your MySQL Database: Ensure that you have a running MySQL database. You can use services like Google Cloud SQL for MySQL for high performance and scalability.
-
Configure Environment Variables: Set up environment variables for secure database connections. For example:
import os os.environ['MYSQL_USER'] = 'your_username' os.environ['MYSQL_PASSWORD'] = 'your_password' os.environ['MYSQL_HOST'] = 'your_host' os.environ['MYSQL_DB'] = 'your_database'
-
Initialize LangChain: Create a new Python script or Jupyter Notebook file and initialize LangChain with your MySQL database:
from langchain import LangChain from sqlalchemy import create_engine engine = create_engine(f"mysql+pymysql://{os.getenv('MYSQL_USER')}:{os.getenv('MYSQL_PASSWORD')}@{os.getenv('MYSQL_HOST')}/{os.getenv('MYSQL_DB')}") langchain = LangChain(engine)
Basic LangChain Concepts
Understanding Language Models
Language models are at the core of LangChain. These models, such as OpenAI’s GPT-3, are trained on vast amounts of text data and can generate human-like responses to text inputs. They are capable of understanding context, generating coherent sentences, and even performing tasks like translation and summarization.
In the context of LangChain MySQL, language models are used to interpret user queries and translate them into SQL commands. This allows for seamless interaction with databases, making it easier to retrieve and manage data.
Creating Your First Language Model
To create your first language model using LangChain, follow these steps:
-
Import the Necessary Modules:
from langchain.models import OpenAI
-
Initialize the Language Model:
model = OpenAI(api_key=os.getenv('OPENAI_API_KEY'))
-
Define a Prompt: Create a prompt that instructs the model on what task to perform. For example:
prompt = "Translate the following natural language query into an SQL statement: 'Show me all users who signed up in the last month.'"
-
Generate a Response: Use the model to generate a response based on the prompt:
response = model.generate(prompt) print(response)
By following these steps, you can harness the power of LangChain to build intelligent chatbots that interact with MySQL databases using natural language.
Integrating LangChain with MySQL
Introduction to MySQL
What is MySQL?
MySQL is a widely-used open-source relational database management system (RDBMS). It is known for its reliability, robustness, and ease of use. MySQL enables developers to manage and manipulate data efficiently, making it a popular choice for web applications, data warehousing, and logging applications. Its compatibility with various platforms and support for numerous programming languages make it versatile and adaptable to different development environments.
Key Features of MySQL
MySQL offers several key features that enhance its functionality and performance:
- High Performance: MySQL’s architecture is optimized for speed and efficiency, ensuring quick data retrieval and manipulation.
- Scalability: It supports large-scale applications with the ability to handle extensive databases and high transaction volumes.
- Security: MySQL provides robust security features, including user authentication, data encryption, and access control.
- Replication: It supports master-slave replication, enabling data redundancy and load balancing.
- Flexibility: MySQL is compatible with various storage engines, allowing developers to choose the best fit for their needs.
- Community Support: Being open-source, MySQL has a vast community of developers contributing to its continuous improvement and offering support.
Setting Up MySQL
Installation Requirements
Before setting up MySQL, ensure your system meets the following requirements:
- A supported operating system (e.g., Windows, macOS, Linux)
- Sufficient disk space and memory
- Administrative privileges to install software
Additionally, you will need to download the MySQL installer from the official MySQL website.
Step-by-Step Installation Guide
Follow these steps to install MySQL on your system:
-
Download MySQL Installer: Visit the MySQL Downloads page and download the appropriate installer for your operating system.
-
Run the Installer: Launch the installer and follow the on-screen instructions. Choose the setup type that best suits your needs (e.g., Developer Default, Server Only).
-
Configure MySQL Server: During the installation process, you will be prompted to configure the MySQL server. Set the root password and create additional user accounts if necessary.
-
Complete Installation: Once the configuration is complete, finish the installation process. You can verify the installation by opening the MySQL Command Line Client and logging in with the root account.
-
Install MySQL Workbench (Optional): For a graphical interface to manage your databases, you can also install MySQL Workbench, available on the same downloads page.
Connecting LangChain to MySQL
Configuring the Connection
To connect LangChain to your MySQL database, you need to configure the connection settings. This involves setting up environment variables and initializing the connection in your Python script.
-
Set Environment Variables: Configure the necessary environment variables for secure database connections:
import os os.environ['MYSQL_USER'] = 'your_username' os.environ['MYSQL_PASSWORD'] = 'your_password' os.environ['MYSQL_HOST'] = 'your_host' os.environ['MYSQL_DB'] = 'your_database'
-
Initialize the Connection: Use the
sqlalchemy
library to create an engine that connects to your MySQL database:from sqlalchemy import create_engine engine = create_engine(f"mysql+pymysql://{os.getenv('MYSQL_USER')}:{os.getenv('MYSQL_PASSWORD')}@{os.getenv('MYSQL_HOST')}/{os.getenv('MYSQL_DB')}")
-
Integrate with LangChain: Initialize LangChain with the created engine:
from langchain import LangChain langchain = LangChain(engine)
Testing the Connection
Once the connection is configured, it’s crucial to test it to ensure everything is working correctly.
-
Run a Test Query: Execute a simple query to verify the connection:
result = engine.execute("SELECT 1") print(result.fetchone())
-
Check for Errors: If there are any issues, check the error messages and ensure that your environment variables and database credentials are correct.
By following these steps, you can seamlessly integrate LangChain MySQL, enabling your chatbot to interact with the database using natural language queries. This integration leverages the advanced NLP capabilities of LangChain and the robust data management of MySQL, providing a powerful solution for building intelligent chatbots.
Building Your First Intelligent Chatbot
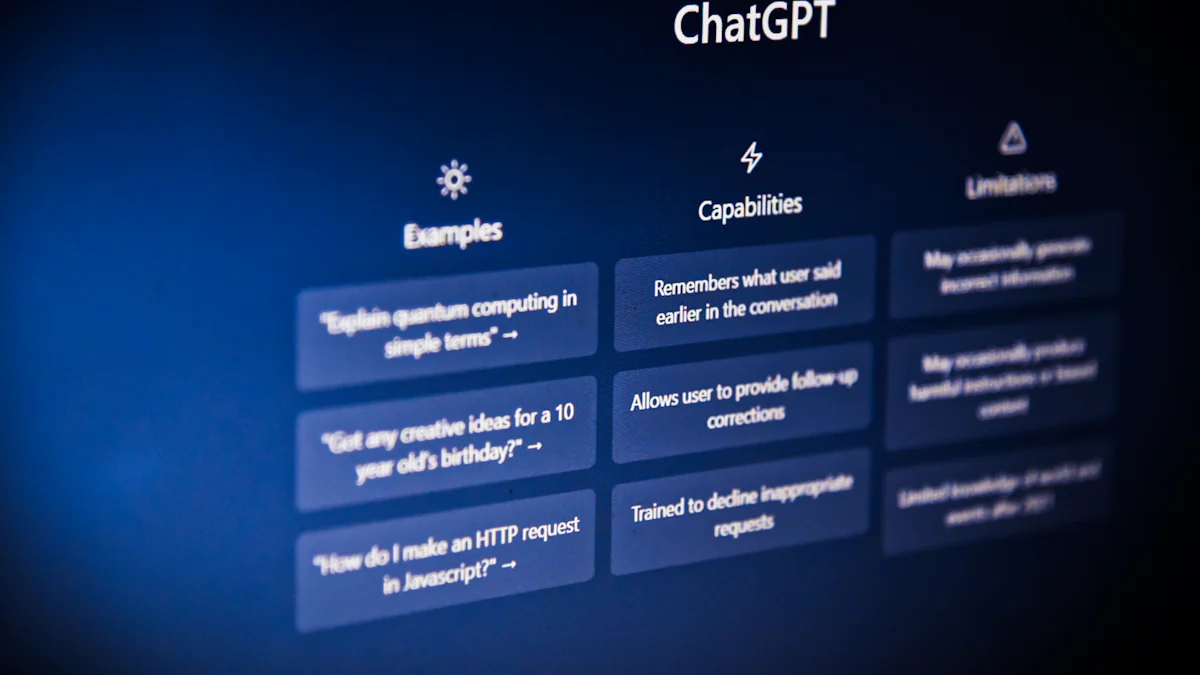
Designing the Chatbot
Defining the Chatbot’s Purpose
The first step in building an intelligent chatbot is to clearly define its purpose. This involves understanding the specific needs of your users and how the chatbot can address them. For instance, you might want a customer support bot that can handle common inquiries, a sales assistant that guides users through product selections, or an internal tool for querying company databases.
Tip: Clearly defining the chatbot’s purpose will help you design more effective conversation flows and ensure that the bot meets user expectations.
Planning the Conversation Flow
Once the purpose is defined, the next step is to plan the conversation flow. This involves mapping out how the chatbot will interact with users, including the types of questions it will ask, the responses it will give, and how it will handle different scenarios. Tools like flowcharts can be very useful for visualizing these interactions.
- Identify Key Interactions: Determine the main points of interaction between the user and the chatbot.
- Design User Prompts: Create prompts that guide the user through the conversation.
- Handle Edge Cases: Plan for unexpected inputs or errors to ensure a smooth user experience.
Example: If you’re building a customer support bot, your flow might include greeting the user, asking for their issue, providing solutions, and escalating to a human agent if needed.
Implementing the Chatbot
Writing the Chatbot Code
With the conversation flow planned, it’s time to start coding. Using LangChain, you can leverage advanced NLP models to interpret user inputs and generate appropriate responses. Here’s a basic structure to get you started:
-
Initialize LangChain:
from langchain import LangChain from sqlalchemy import create_engine import os engine = create_engine(f"mysql+pymysql://{os.getenv('MYSQL_USER')}:{os.getenv('MYSQL_PASSWORD')}@{os.getenv('MYSQL_HOST')}/{os.getenv('MYSQL_DB')}") langchain = LangChain(engine)
-
Define the Conversation Logic:
def handle_user_input(user_input): prompt = f"Translate the following natural language query into an SQL statement: '{user_input}'" response = langchain.generate(prompt) return response
-
Create the Main Loop:
while True: user_input = input("You: ") if user_input.lower() == "exit": break response = handle_user_input(user_input) print(f"Bot: {response}")
Integrating LangChain and MySQL
Integrating LangChain with MySQL allows your chatbot to perform complex data retrieval and manipulation tasks using natural language. This integration is crucial for making your chatbot truly intelligent and capable of handling real-world queries.
-
Set Up Database Connection:
from sqlalchemy import create_engine engine = create_engine(f"mysql+pymysql://{os.getenv('MYSQL_USER')}:{os.getenv('MYSQL_PASSWORD')}@{os.getenv('MYSQL_HOST')}/{os.getenv('MYSQL_DB')}")
-
Execute SQL Queries:
def execute_query(query): result = engine.execute(query) return result.fetchall()
-
Integrate with LangChain:
def handle_user_input(user_input): prompt = f"Translate the following natural language query into an SQL statement: '{user_input}'" sql_query = langchain.generate(prompt) results = execute_query(sql_query) return results
Testing and Debugging
Common Issues and Solutions
While developing your chatbot, you may encounter several common issues. Here are some tips to address them:
- Incorrect SQL Translation: Ensure that the prompts used to generate SQL queries are clear and specific.
- Connection Errors: Verify that your database credentials and environment variables are correctly set.
- Performance Bottlenecks: Optimize your SQL queries and consider indexing frequently accessed tables.
Pro Tip: Regularly test your chatbot with a variety of inputs to identify and fix issues early.
Best Practices for Testing
Effective testing is essential for ensuring that your chatbot performs well in real-world scenarios. Here are some best practices:
- Unit Testing: Test individual components of your chatbot to ensure they work as expected.
- Integration Testing: Verify that LangChain and MySQL integration works seamlessly.
- User Testing: Conduct tests with real users to gather feedback and make necessary adjustments.
Case Study: In the guide “Chat with MySQL Using Python and LangChain,” developers successfully created custom chains for chatting with databases using natural language, showcasing the practical application of these testing practices.
By following these steps, you can build a robust and intelligent chatbot that leverages the power of LangChain and MySQL. This combination allows for seamless natural language interactions with your database, providing a user-friendly interface for complex data management tasks.
Advanced Features and Optimization
Enhancing Chatbot Intelligence
Using Advanced Language Models
To elevate the intelligence of your chatbot, leveraging advanced language models is crucial. These models, such as OpenAI’s GPT-4, offer enhanced capabilities in understanding and generating human-like text. By integrating these models with LangChain, you can create chatbots that provide more accurate and contextually relevant responses.
Key Benefits:
- Improved Understanding: Advanced language models can better comprehend complex queries and nuances in user inputs.
- Enhanced Responses: They generate more coherent and contextually appropriate replies, making interactions feel more natural.
- Broader Knowledge Base: These models are trained on diverse datasets, enabling them to handle a wide range of topics and questions.
For instance, using LangChain’s prompt templates, you can fine-tune the chatbot’s personality and responses, ensuring it behaves realistically and aligns with your brand’s voice. This customization is particularly valuable for creating engaging and consistent user experiences.
Incorporating Machine Learning Techniques
Incorporating machine learning techniques into your chatbot can further enhance its capabilities. Machine learning algorithms can analyze user interactions, identify patterns, and continuously improve the chatbot’s performance over time.
Steps to Incorporate Machine Learning:
- Data Collection: Gather user interaction data to train your models.
- Model Training: Use this data to train machine learning models that can predict user intents and generate appropriate responses.
- Continuous Improvement: Implement feedback loops where the chatbot learns from each interaction, refining its responses and improving accuracy.
Machine learning can also be used to personalize user experiences. For example, by analyzing past interactions, the chatbot can tailor its responses to individual users, providing a more personalized and engaging experience.
Optimizing Performance
Database Optimization Tips
Optimizing your MySQL database is essential for ensuring your chatbot performs efficiently, especially as the volume of data and number of queries increase.
Tips for Database Optimization:
- Indexing: Create indexes on frequently queried columns to speed up data retrieval.
- Query Optimization: Analyze and optimize SQL queries to reduce execution time. Use tools like
EXPLAIN
to understand query performance. - Partitioning: Partition large tables to improve query performance and manageability.
- Caching: Implement caching strategies to store frequently accessed data, reducing the load on the database.
By following these optimization tips, you can significantly improve the performance of your MySQL database, ensuring that your chatbot can handle high volumes of queries efficiently.
Improving Response Times
Fast response times are critical for maintaining a smooth and engaging user experience. Here are some strategies to improve your chatbot’s response times:
- Asynchronous Processing: Use asynchronous processing to handle multiple requests concurrently, reducing wait times for users.
- Load Balancing: Distribute the load across multiple servers to prevent any single server from becoming a bottleneck.
- Efficient Code: Write efficient and optimized code to minimize processing time. Avoid unnecessary computations and streamline your logic.
By implementing these strategies, you can ensure that your chatbot responds quickly to user queries, providing a seamless and satisfying experience.
Real-World Use Cases
Examples of Successful Chatbots
Several organizations have successfully implemented intelligent chatbots using LangChain and MySQL, demonstrating the potential of these technologies.
Case Study: Customer Support Bot
A leading e-commerce company developed a customer support bot using LangChain and MySQL. The bot handles common inquiries, processes returns, and provides order status updates. By leveraging advanced language models, the bot understands and responds to a wide range of customer queries, reducing the workload on human agents and improving customer satisfaction.
Case Study: Sales Assistant
A tech startup created a sales assistant chatbot to guide users through product selections. The bot uses machine learning to analyze user preferences and recommend products. By integrating with MySQL, the bot accesses real-time inventory data, ensuring accurate and up-to-date recommendations.
Lessons Learned from Real-World Implementations
Implementing intelligent chatbots comes with its own set of challenges and lessons. Here are some key takeaways from real-world implementations:
- User Feedback: Continuously gather and analyze user feedback to identify areas for improvement.
- Scalability: Design your system to scale efficiently as the number of users and volume of data grows.
- Security: Ensure robust security measures are in place to protect user data and maintain privacy.
- Maintenance: Regularly update and maintain your chatbot to incorporate new features and address any issues.
By learning from these real-world examples, you can build more effective and resilient chatbots that deliver exceptional user experiences.
Leveraging TiDB for Enhanced Capabilities
Introduction to TiDB
TiDB is an advanced, open-source, distributed SQL database that offers MySQL compatibility and supports Hybrid Transactional and Analytical Processing (HTAP) workloads. This makes it an ideal choice for applications requiring high availability, strong consistency, and large-scale data processing.
Key Features of TiDB
TiDB’s architecture and features provide several advantages for integrating with LangChain MySQL:
- Easy Horizontal Scaling: TiDB separates computing from storage, allowing you to scale out or scale in the computing or storage capacity online as needed. This ensures that your application can handle increasing loads without significant downtime.
- Financial-Grade High Availability: With data stored in multiple replicas and the Multi-Raft protocol ensuring strong consistency, TiDB guarantees high availability even when some replicas fail. You can configure the geographic location and number of replicas to meet different disaster tolerance levels.
- Real-Time HTAP: TiDB provides two storage engines: TiKV (row-based) and TiFlash (columnar). TiFlash replicates data from TiKV in real-time, ensuring consistent data between the two engines and solving HTAP resource isolation issues.
- Cloud-Native Distributed Database: Designed for the cloud, TiDB offers flexible scalability, reliability, and security. TiDB Cloud, the fully-managed service, allows you to deploy and run TiDB clusters with just a few clicks.
- MySQL Compatibility: TiDB is compatible with the MySQL protocol and ecosystem, enabling seamless migration of applications without changing code in many cases. It also provides a series of data migration tools.
Benefits of Using TiDB with LangChain
Integrating LangChain MySQL with TiDB brings numerous benefits:
- Enhanced Performance: TiDB’s horizontal scalability and high availability ensure that your chatbot can handle large volumes of queries efficiently.
- Seamless Integration: TiDB’s MySQL compatibility allows for easy integration with LangChain, enabling natural language interactions with your database.
- Advanced Capabilities: The combination of TiDB’s real-time HTAP capabilities and LangChain’s NLP prowess allows for sophisticated data processing and retrieval, enhancing the intelligence of your chatbot.
Setting Up TiDB
Installation Requirements
Before setting up TiDB, ensure your system meets the following requirements:
- A supported operating system (e.g., Windows, macOS, Linux)
- Sufficient disk space and memory
- Administrative privileges to install software
Additionally, you will need to download the TiDB installer from the official TiDB website.
Step-by-Step Installation Guide
Follow these steps to install TiDB on your system:
-
Download TiDB Installer: Visit the TiDB Downloads page and download the appropriate installer for your operating system.
-
Run the Installer: Launch the installer and follow the on-screen instructions. Choose the setup type that best suits your needs (e.g., Developer Default, Server Only).
-
Configure TiDB Server: During the installation process, you will be prompted to configure the TiDB server. Set the necessary parameters and create user accounts if required.
-
Complete Installation: Once the configuration is complete, finish the installation process. You can verify the installation by opening the TiDB Command Line Client and logging in with the root account.
-
Install TiDB Workbench (Optional): For a graphical interface to manage your databases, you can also install TiDB Workbench, available on the same downloads page.
Integrating TiDB with LangChain
Configuring the Connection
To connect LangChain to your TiDB database, you need to configure the connection settings. This involves setting up environment variables and initializing the connection in your Python script.
-
Set Environment Variables: Configure the necessary environment variables for secure database connections:
import os os.environ['TIDB_USER'] = 'your_username' os.environ['TIDB_PASSWORD'] = 'your_password' os.environ['TIDB_HOST'] = 'your_host' os.environ['TIDB_DB'] = 'your_database'
-
Initialize the Connection: Use the
sqlalchemy
library to create an engine that connects to your TiDB database:from sqlalchemy import create_engine engine = create_engine(f"mysql+pymysql://{os.getenv('TIDB_USER')}:{os.getenv('TIDB_PASSWORD')}@{os.getenv('TIDB_HOST')}/{os.getenv('TIDB_DB')}")
-
Integrate with LangChain: Initialize LangChain with the created engine:
from langchain import LangChain langchain = LangChain(engine)
Testing the Connection
Once the connection is configured, it’s crucial to test it to ensure everything is working correctly.
-
Run a Test Query: Execute a simple query to verify the connection:
result = engine.execute("SELECT 1") print(result.fetchone())
-
Check for Errors: If there are any issues, check the error messages and ensure that your environment variables and database credentials are correct.
By following these steps, you can seamlessly integrate LangChain MySQL with TiDB, enabling your chatbot to interact with the database using natural language queries. This integration leverages the advanced NLP capabilities of LangChain and the robust data management of TiDB, providing a powerful solution for building intelligent chatbots.
In this blog, we’ve explored the powerful combination of LangChain and MySQL for building intelligent chatbots. By leveraging LangChain’s advanced NLP capabilities and MySQL’s robust data management, developers can create chatbots that transform complex SQL queries into straightforward conversations.
Whether you’re a developer, database administrator, or someone looking to streamline data workflows, this integration offers an intuitive interface that simplifies database interactions. We encourage you to start building with LangChain and MySQL today and unlock the full potential of your data.
For further learning, check out the following resources:
See Also
Creating a Chatbot using LlamaIndex and TiDB Vector Search
Developing a Chatbot with AWS Bedrock and Streamlit
Guides on LangChain for Data Storage with TiDB Serverless
Innovative Web App Features with OpenAI and MySQL
Constructing a RAG Application with LlamaIndex and TiDB Serverless