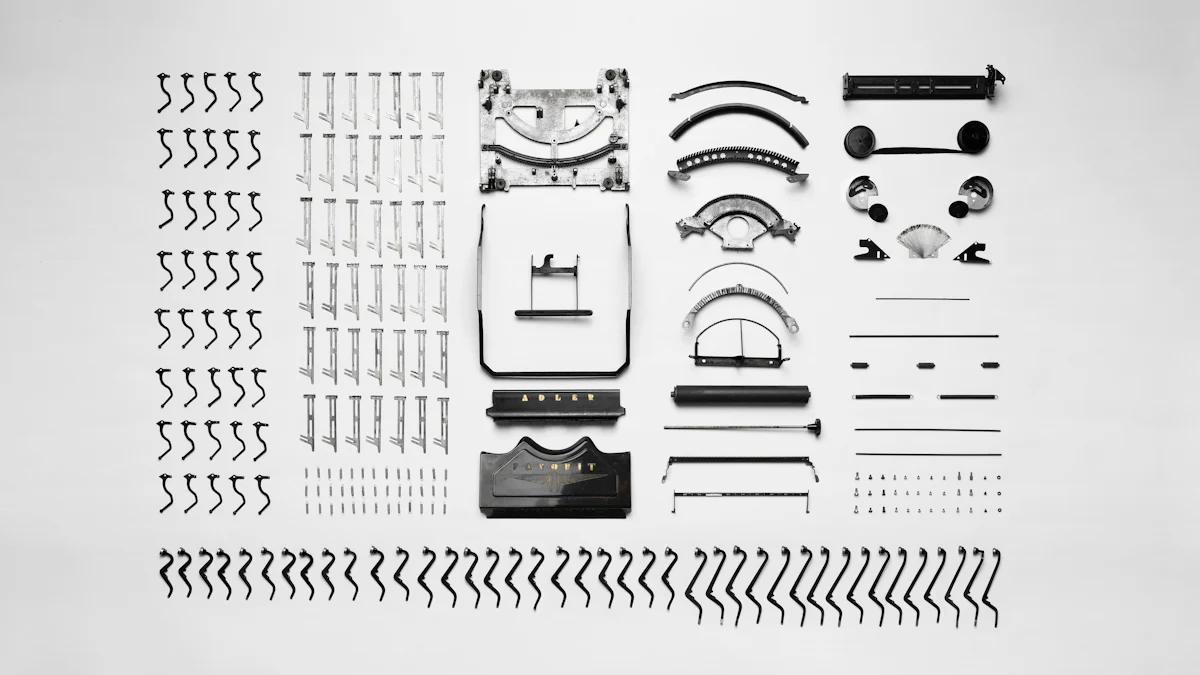
LangChain is a powerful framework designed to enhance the capabilities of conversational AI by integrating langchain memory into its systems. Implementing langchain memory is crucial for maintaining context across interactions, ensuring coherent and meaningful conversations. This guide aims to provide a comprehensive understanding of how to effectively implement and manage langchain memory within LangChain, enabling developers to optimize performance and resource management.
Understanding LangChain Memory
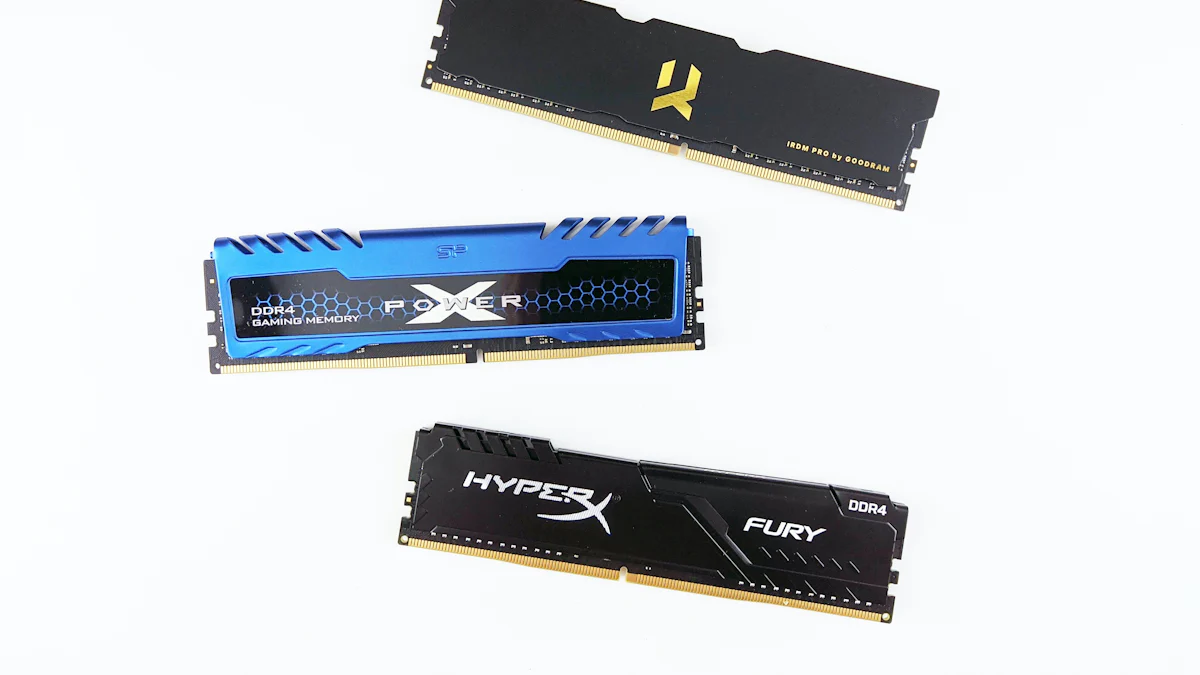
Basic Concepts
What is LangChain?
LangChain is a versatile framework designed to enhance conversational AI by integrating memory management into its core functionalities. This framework supports various types of memory, including Conversational Memory, Buffer Memory, and Entity Memory, each tailored to different use cases. By leveraging these memory types, LangChain ensures that chatbots and virtual assistants can maintain context across interactions, leading to more coherent and meaningful conversations.
Overview of Memory Management
Memory management in LangChain involves the efficient handling of data throughout the lifecycle of a conversation. This includes storing, retrieving, and updating information as needed. The langchain memory module is responsible for persisting the state between calls of a chain or agent, enabling the language model to remember previous interactions and use that information to make better decisions. This capability is crucial for applications that require maintaining context over multiple steps, such as customer support chatbots or virtual assistants.
Importance of Memory in LangChain
Performance Optimization
Implementing effective langchain memory is vital for optimizing the performance of conversational AI systems. By efficiently managing memory, LangChain reduces the computational overhead associated with repeatedly processing the same information. This leads to faster response times and a smoother user experience. For example, using memory pools and garbage collection techniques helps in reclaiming unused memory, thereby enhancing the overall performance of the system.
Resource Management
Resource management is another critical aspect of langchain memory. In complex conversational workflows, especially those involving multiple steps, managing resources effectively ensures that the system remains responsive and scalable. LangChain offers several memory types, such as Redis-Backed Chat Memory and DynamoDB-Backed Chat Memory, which are designed to handle large volumes of data efficiently. These memory types help in maintaining context and continuity in conversations, making them particularly beneficial for chatbots and virtual assistants.
By understanding and implementing these basic concepts and importance of memory in LangChain, developers can create more robust and efficient conversational AI systems. The next section will delve into setting up LangChain for memory implementation, providing a step-by-step guide to get you started.
Setting Up LangChain for Memory Implementation
Prerequisites
Before diving into the implementation of memory in LangChain, it’s essential to ensure that you have the necessary tools and a properly configured environment. This section will guide you through the prerequisites needed to get started.
Required Tools and Libraries
To implement memory in LangChain, you’ll need to have the following tools and libraries installed:
-
Python: Ensure you have Python 3.7 or higher installed on your system.
-
LangChain Library: The core library for implementing memory in LangChain.
-
Database Systems: Depending on your memory type, you might need databases like Redis, DynamoDB, or others.
-
Development Environment: An IDE or text editor such as VSCode, PyCharm, or Sublime Text.
You can install the LangChain library using pip:
pip install langchain
Additionally, if you plan to use specific memory types like Redis-Backed Chat Memory, you will need to install the corresponding database clients:
pip install redis
pip install boto3 # For DynamoDB
Environment Setup
Setting up your development environment is crucial for smooth implementation. Follow these steps to configure your environment:
- Create a Virtual Environment: It’s good practice to create a virtual environment to manage dependencies.
python -m venv langchain-env
source langchain-env/bin/activate # On Windows, use `langchain-envScriptsactivate`
- Install Dependencies: With the virtual environment activated, install the required libraries.
pip install langchain redis boto3
-
Configure Database Connections: Ensure your databases (e.g., Redis, DynamoDB) are running and accessible. Configure connection settings as needed.
-
Set Environment Variables: For secure handling of credentials and configurations, use environment variables.
export REDIS_URL=redis://localhost:6379
export AWS_ACCESS_KEY_ID=your_access_key
export AWS_SECRET_ACCESS_KEY=your_secret_key
Installation Guide
With the prerequisites in place, you can now proceed with the installation and setup of LangChain for memory implementation. This section provides a step-by-step guide to help you through the process.
Step-by-Step Installation
- Install LangChain: If you haven’t already installed LangChain, do so using pip.
pip install langchain
- Initialize a LangChain Project: Create a new project directory and initialize it.
mkdir langchain_project
cd langchain_project
- Create a Python Script: Create a new Python script (e.g.,
main.py
) where you will implement LangChain memory.
from langchain import LangChain
from langchain.memory import RedisMemory
# Initialize LangChain
lc = LangChain()
# Set up Redis-backed memory
memory = RedisMemory(redis_url="redis://localhost:6379")
lc.set_memory(memory)
# Example usage
lc.run("Hello, how can I assist you today?")
- Run Your Script: Execute your script to ensure everything is set up correctly.
python main.py
Common Issues and Troubleshooting
During the setup and installation process, you might encounter some common issues. Here are a few troubleshooting tips:
-
Dependency Conflicts: Ensure all dependencies are compatible with each other. Use a virtual environment to avoid conflicts.
-
Database Connection Errors: Verify your database connection settings and ensure the database server is running.
-
Environment Variable Issues: Double-check that all necessary environment variables are set correctly.
If you encounter any issues, refer to the official documentation or community forums for additional support.
By following these steps, you will have a fully functional LangChain setup ready for memory implementation. In the next section, we will explore various memory allocation techniques and management strategies to optimize your LangChain applications.
Implementing Memory in LangChain
Memory Allocation Techniques
Memory allocation is a critical aspect of implementing langchain memory effectively. It involves deciding how and where to allocate memory for various tasks within LangChain, ensuring optimal performance and resource utilization.
Static vs Dynamic Allocation
In the realm of memory allocation, two primary techniques are commonly employed: static and dynamic allocation.
-
Static Allocation: This technique involves allocating memory at compile time. The size and location of memory are determined before the program runs. Static allocation is straightforward and offers fast access since the memory addresses are fixed. However, it lacks flexibility, making it less suitable for applications with varying memory requirements.
-
Dynamic Allocation: Unlike static allocation, dynamic allocation occurs at runtime. Memory is allocated as needed, allowing for greater flexibility and efficient use of resources. This technique is particularly beneficial for conversational AI systems where the memory requirements can change based on user interactions. LangChain supports dynamic allocation through various memory types like Redis-Backed Chat Memory and DynamoDB-Backed Chat Memory, which can scale according to the application’s needs.
Best Practices
Implementing langchain memory effectively requires adhering to best practices that ensure optimal performance and resource management:
-
Choose the Right Memory Type: Select the appropriate memory type based on your application’s specific needs. For instance, use Conversation Buffer Memory for simple conversational contexts and Entity Memory for more complex scenarios involving multiple entities.
-
Monitor Memory Usage: Regularly profile and analyze memory usage to identify bottlenecks and optimize performance. Tools like memory profilers can help in tracking memory consumption and detecting leaks.
-
Combine Multiple Memory Types: Leverage the power of combining different memory types to enhance conversational context and recall. For example, combining Conversation Buffer Memory with Entity Memory can provide a comprehensive solution tailored to your application’s requirements.
-
Optimize Data Structures: Use efficient data structures to store and manage memory. This can significantly reduce memory overhead and improve access times.
Memory Management Strategies
Effective memory management is crucial for maintaining the performance and stability of LangChain applications. Two key strategies are garbage collection and memory pools.
Garbage Collection
Garbage collection is an automated process of reclaiming memory that is no longer in use. In the context of langchain memory, garbage collection helps in managing memory efficiently by automatically freeing up unused memory, thus preventing memory leaks and reducing the risk of application crashes.
-
Automatic Memory Reclamation: LangChain’s built-in garbage collection mechanisms ensure that memory is reclaimed without manual intervention, allowing developers to focus on building robust conversational AI systems.
-
Performance Optimization: By periodically cleaning up unused memory, garbage collection helps in maintaining optimal performance, especially in long-running applications where memory usage can accumulate over time.
Memory Pools
Memory pools are pre-allocated blocks of memory that can be reused for different tasks. This strategy is particularly useful in high-performance applications where frequent memory allocation and deallocation can lead to fragmentation and increased overhead.
-
Efficient Memory Allocation: Memory pools allow for quick allocation and deallocation of memory, reducing the time spent on managing memory dynamically. This leads to faster response times and improved user experience.
-
Reduced Fragmentation: By reusing pre-allocated memory blocks, memory pools minimize fragmentation, ensuring that memory is used efficiently and consistently.
Implementing these memory management strategies in LangChain can significantly enhance the performance and reliability of your conversational AI systems. By understanding and applying these techniques, developers can create more robust and efficient applications that maintain context and coherence across interactions.
Advanced Memory Management Techniques
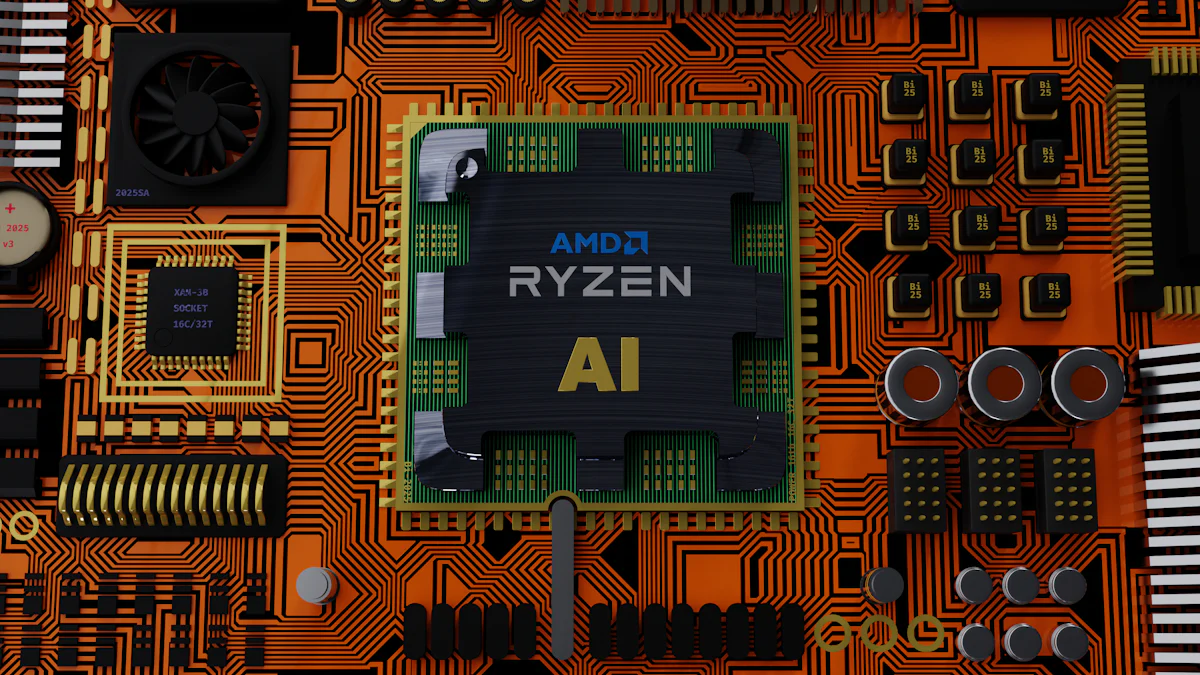
As we delve deeper into LangChain, it’s crucial to explore advanced memory management techniques that can significantly enhance the performance and efficiency of your conversational AI systems. This section covers optimizing memory usage through profiling and analysis, various optimization techniques, and real-world examples to illustrate these concepts.
Optimizing Memory Usage
Optimizing memory usage is essential for maintaining high performance and ensuring that your LangChain applications run smoothly. Here, we will discuss profiling and analysis, as well as specific optimization techniques.
Profiling and Analysis
Profiling and analyzing memory usage is the first step towards optimization. By understanding how memory is allocated and utilized, you can identify bottlenecks and areas for improvement.
-
Memory Profilers: Tools like
memory_profiler
andtracemalloc
in Python can help you track memory usage over time. These tools provide detailed reports on memory consumption, allowing you to pinpoint inefficient memory usage. -
Heap Analysis: Analyzing the heap can reveal how memory is being allocated and deallocated. This can help you understand the lifecycle of objects and identify memory leaks.
-
Garbage Collection Logs: Reviewing garbage collection logs can provide insights into how often garbage collection occurs and how much memory is being reclaimed. This information is crucial for tuning garbage collection settings to optimize performance.
Optimization Techniques
Once you have a clear understanding of memory usage through profiling and analysis, you can apply various optimization techniques to improve performance.
-
Efficient Data Structures: Use data structures that are optimized for memory usage. For example, using
deque
from thecollections
module for queues can be more memory-efficient than using lists. -
Memory Pools: Implement memory pools to reduce the overhead of frequent memory allocation and deallocation. Memory pools allow you to reuse pre-allocated memory blocks, which can significantly improve performance.
-
Lazy Loading: Load data only when it is needed. This technique, known as lazy loading, can help reduce memory consumption by avoiding the allocation of memory for unused data.
-
Batch Processing: Process data in batches to minimize memory usage. This technique is particularly useful for handling large datasets, as it allows you to process smaller chunks of data sequentially rather than loading everything into memory at once.
By applying these optimization techniques, you can ensure that your LangChain applications use memory efficiently, leading to faster response times and a better user experience.
Real-World Examples
To illustrate the effectiveness of these advanced memory management techniques, let’s look at some real-world examples, including PingCAP’s TiDB integration.
Case Study 1: PingCAP’s TiDB Integration
PingCAP’s TiDB database is renowned for its ability to handle large-scale data with high availability and strong consistency. Integrating TiDB with LangChain can significantly enhance memory management in conversational AI applications.
-
Scalability: TiDB’s horizontal scalability ensures that memory can be dynamically allocated based on the application’s needs. This is particularly beneficial for LangChain applications that require handling large volumes of conversational data.
-
Efficient Querying: TiDB’s advanced querying capabilities allow for efficient retrieval of memory data. This ensures that LangChain can quickly access and update memory, maintaining context across interactions without compromising performance.
-
Hybrid Transactional and Analytical Processing (HTAP): TiDB supports HTAP workloads, enabling LangChain to perform real-time analytics on conversational data. This capability allows for more intelligent decision-making and improved user experiences.
By leveraging TiDB’s robust features, LangChain can achieve optimal memory management, ensuring that conversational AI applications are both scalable and efficient.
Case Study 2: Another Real-World Application
Consider a customer support chatbot implemented using LangChain. This chatbot handles thousands of interactions daily, requiring efficient memory management to maintain context and provide accurate responses.
-
Dynamic Memory Allocation: The chatbot uses dynamic memory allocation to handle varying memory requirements based on user interactions. This ensures that memory is allocated only when needed, reducing overall memory consumption.
-
Garbage Collection: The chatbot employs garbage collection to automatically reclaim unused memory. This prevents memory leaks and ensures that the system remains responsive even during peak usage.
-
Memory Pools: To further optimize performance, the chatbot uses memory pools for frequently accessed data. This reduces the overhead of memory allocation and deallocation, leading to faster response times.
By implementing these advanced memory management techniques, the customer support chatbot can efficiently handle large volumes of interactions while maintaining high performance and reliability.
In summary, implementing LangChain memory is essential for optimizing conversational AI systems. By understanding basic concepts, setting up the environment, and applying advanced memory management techniques, developers can significantly enhance performance and resource efficiency.
LangChain memory, with its dynamic allocation and integrated memory management, offers a robust solution for maintaining context in interactions. We encourage you to apply these techniques to your projects and explore further learning opportunities.
Stay curious, keep experimenting, and continue pushing the boundaries of what’s possible with LangChain and conversational AI.
See Also
Guides for LangChain Data Management using TiDB Serverless
Overview of Powerful Large Language Model (LLM)
Guide to Constructing RAG System with Llama3 and TiDB